How to Count Occurrences in String Using JavaScript
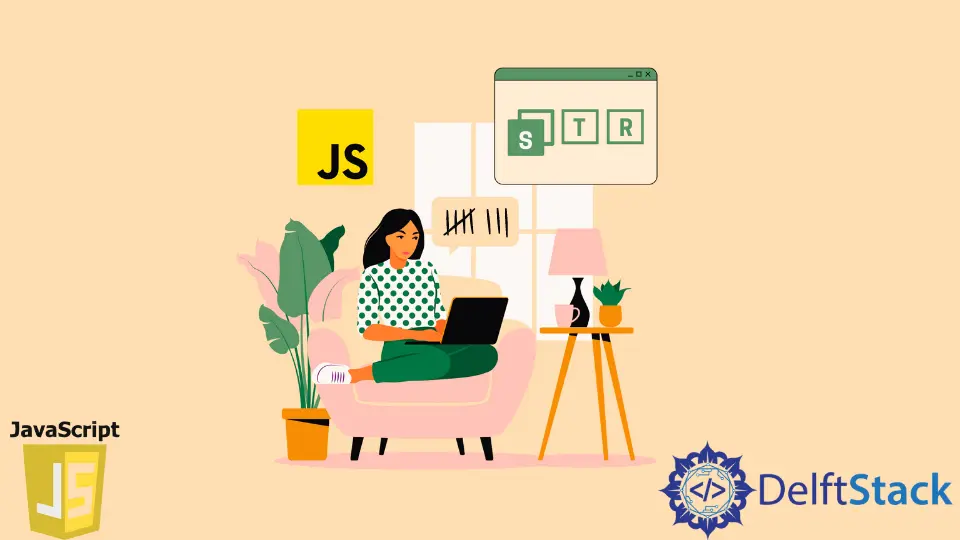
We will use the JavaScript match method to count the total occurrences of specific words/characters in a string. But before we learn that, let’s understand what regex is.
Concept of RegEx
in JavaScript
Regular expressions are sort of a search that may realize specific string patterns. JavaScript additionally supports regular expressions as an object.
These patterns are used with the split()
, replace()
, search()
, match()
, matchAll()
and replaceAll()
.
There are two ways to construct the regular expression, either using a literal regular expression or calling the RegEx’s constructor.
Further information on the RegEx can be found in the documentation for the RegEx
.
Before moving on to the matching strings counting occurrences, Let’s understand some common patterns.
- The
\d
is a regular expression that matches any number/digit. It is similar to[0-9]
. - The
\w
is a regular expression that matches any given word character code in the range ofA
-Z
,a
-z
,0
-9
, and_
. - The
\s
is a regular expression that matches every whitespace character, such as spaces, tabs, line breaks. - The
\b
is a regular expression that matches any word boundary position between a word character and a non-word character or a position (beginning/end of the character string). - The
[A-Z]
is a regular expression that matches any given character code in the range fromA
-Z
. - The
[0-9]
is a regular expression that matches any given character code in the range from0
-9
.
Each RegEx contains certain flags. Each flag has its meaning, which provides additional properties to the regex.
Some of them are listed below:
- The
i
flag is case-insensitive, which means that the entire expression is case-insensitive. - The
g
flag means a global search that preserves the index of the last match, allowing subsequent searches to start at the end of the previous match. Without the global flag, subsequent searches return the same match. - The
m
flag means multiline. When the multiline flag is on, the starting and ending anchor points^ and $
match the start and end of a line, not the start and end of the entire string. - The
u
flag stands for Unicode. Users can use Unicode extended escapes of the form\x{FFFFF}
by setting this flag.
Syntax:
const regEx = /pattern/;
const regEx = new RegExp('pattern');
function phonenumber() {
const indiaRegex = /^\+91\d{10}$/;
const inputText = document.getElementById('phoneNumber').value;
if (inputText.match(indiaRegex)) {
console.log('Valid phone number');
} else {
console.log('Not a valid Phone Number');
}
}
Count Occurrences in the String Using match()
in JavaScript
The match()
method is an in-built JavaScript method. This method retrieves the result of matching a string/character against a regular expression provided from the original string.
Syntax:
match(regexp)
It takes a regular expression as an input parameter and finds the matching string/character against the original string.
It is an optional parameter. If no regular expression is passed as a parameter in the match method, it will return the array with the empty string.
Depending on the regular expression’s g
(global) flag, it returns the array. If the g
flag is passed, all the matching strings/characters are returned.
See the match
method documentation for more information.
var inputString = 'Hello World. This is a string.';
var count = (inputString.match(/is/g) || []).length;
console.log(count);
In the above code, we are trying to determine the total occurrences of the is
word irrespective of where it is.
If you pass the g
(global) flag, it will try to find out all the matching is. E.g., is
in This
is a valid match.
Once you run the above code in any browser, it will print something like this.
Output:
2
To check the complete working code, click here.
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn