How to Copy Array in JavaScript
-
Use
slice()
to Copy Array Elements From an Array in JavaScript -
Use Spread
...
Operator to Copy Array Elements From an Array in JavaScript
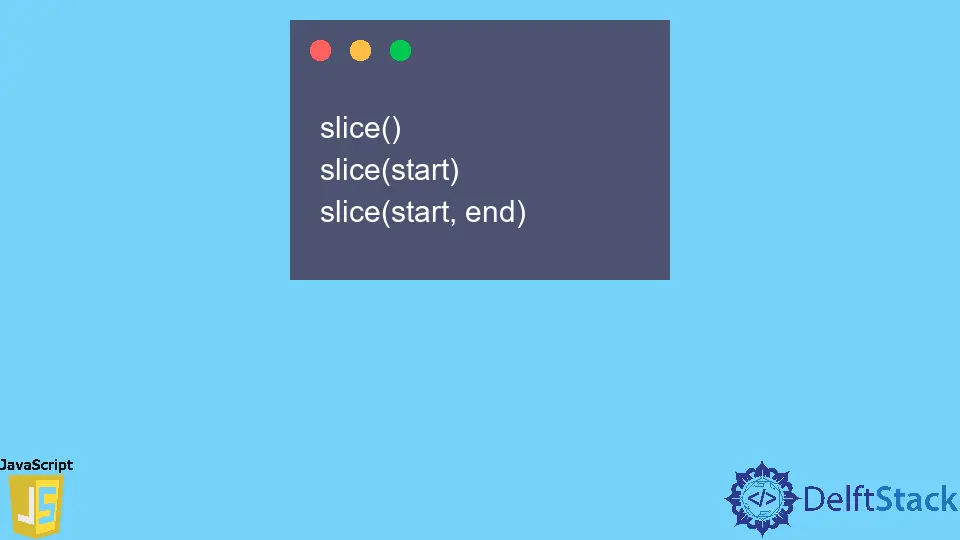
In this article, we will learn how to copy elements of an array into a new array of JavaScript.
In JavaScript, arrays are normal objects containing the value in the desired key, which can be numeric.
Arrays are JavaScript objects with a fixed numerical key and dynamic values containing any amount of data in a single variable. An array is one-dimensional or multi-dimensional.
The JavaScript array can store anything like direct values or store JavaScript objects. In contrast to different languages, JavaScript arrays can hold different data on different indexes of identical arrays.
Use slice()
to Copy Array Elements From an Array in JavaScript
The slice()
method is a built-in method provided by JavaScript. This method splits the array into two places.
This cut is performed by taking two inputs, the start index and the end index. And based on that, the part will return an array over the indexes.
If only the starting index is specified, the last element is returned. The advantage of using slice
over splice
is that it doesn’t mutate the original array with a splice
.
Syntax:
slice()
slice(start)
slice(start, end)
Any element present at the start
and end
indices (including the start and stop elements before the end) is inserted into the new array. The end index is a completely optional parameter.
You can find more information about the slice
function in the documentation for slice()
.
const inputArray = ['Kiwi', 'Orange', 'Apple', 'Banana'];
const outputArray1 = inputArray.slice();
console.log(outputArray1);
When we call slice()
, all elements are copied from the original array, i.e., inputArray
to outputArray1
. The entire array is copied since we don’t pass the start or end index.
If the starting index is greater than the length of an array, it will be returned empty, and the empty array will also be returned as output. The interesting part is that if you specify a negative index, the input parameter will be treated as a change from the end of the sequence.
Once you run the code above in any browser, it will print something like this.
Output:
["Kiwi","Orange","Apple","Banana"]
Use Spread ...
Operator to Copy Array Elements From an Array in JavaScript
The spread
(...
) syntax enables the expansion of an iterable, for example, an expression or an array where zero or more arguments (for function calls) or elements (for array literals) are expected or extends an object expression in places where zero or more key-value pairs are expected (for object literals).
Syntax:
const newArray = [...oldArray];
The spread
syntax can be used when all elements of an object or array must be contained in some list.
It’s commonly used to add a new item to a local data store or view all saved items and new addition. A very simple version of this type of action might look like below.
const inputArray = ['Kiwi', 'Orange', 'Apple', 'Banana'];
const outputArray1 = [...inputArray, 'Grapes'];
console.log(outputArray1);
In the example above, you can execute the last row as many times as possible to add more grapes to the end of the array.
Output:
["Kiwi","Orange","Apple","Banana"]
["Kiwi","Orange","Apple","Banana", "Grapes"]
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedInRelated Article - JavaScript Array
- How to Check if Array Contains Value in JavaScript
- How to Create Array of Specific Length in JavaScript
- How to Convert Array to String in JavaScript
- How to Remove First Element From an Array in JavaScript
- How to Search Objects From an Array in JavaScript
- How to Convert Arguments to an Array in JavaScript