How to Convert a String to Boolean in JavaScript
-
Convert a String Representing a Boolean Value (e.g.,
true
,false
) Into a Boolean in JavaScript - Convert the String to Boolean to Check the Empty String:
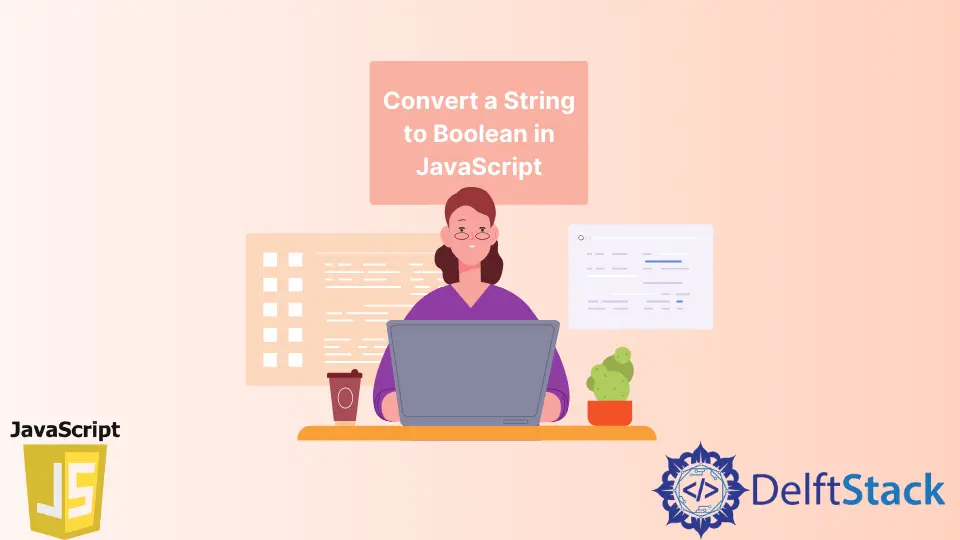
JavaScript has different ways to convert a string to boolean, but it depends on the situation or, in other words, it depends on your purpose of converting string to boolean, because we have two different scenarios, which we will cover below.
Convert a String Representing a Boolean Value (e.g., true
, false
) Into a Boolean in JavaScript
The first scenario is to convert a string representing a boolean value (e.g., true
, false
, yes
, no
, 0
, 1
) into an intrinsic type. We use this scenario in specific cases for example we have HTML form elements and a hidden form that is showed based upon a user’s selection within a check input or select input.
Example:
<input type="checkbox" id="display_hidden_form1" name="display_hidden_form1" value="true">
<label for="display_hidden_form1"> Display hide form1</label><br>
<input type="checkbox" id="display_hidden_form2" name="display_hidden_form2" value="false">
<label for="display_hidden_form2"> Display hide form2</label><br>
<script>
let myValue = document.getElementById("display_hidden_form1").value;
let isTrueval = myValue === 'true';
let myValue2 = document.getElementById("display_hidden_form2").value;
let isTrueval2 = myValue2 === 'true';
console.log({isTrueval, isTrueval2});
</script>Stenogramm Beispiel:
Output:
{isTrueval: true, isTrueval2: false}
Short Example:
const convertString = (word) => {
switch (word.toLowerCase().trim()) {
case 'yes':
case 'true':
case '1':
return true;
case 'no':
case 'false':
case '0':
case null:
return false;
default:
return Boolean(word);
}
} console.log(convertString('true'));
console.log(convertString('no'));
console.log(convertString('dasdasd'));
Output:
true
false
true
Convert the String to Boolean to Check the Empty String:
There are two ways to convert variables to a Boolean value. First by dual NOT operators (!!
), and Second by typecasting (Boolean(value))
.
let myBool = Boolean('false');
let myBool2 = !!'false';
console.log({myBool, myBool2});
Output:
{myBool: true, myBool2: true}
The value
is a variable. It returns false
for null
, undefined
, 0
, 000
, ""
and false
. It returns true
for string, and whitespace.
In the above example, "false"
is a string, therefore, Boolean("false")
returns true
.
You should probably be cautious about using these two methods for the first scenario, any string which isn’t the empty string will evaluate to true
by using them.
Related Article - JavaScript String
- How to Get Last Character of a String in JavaScript
- How to Convert a String Into a Date in JavaScript
- How to Get First Character From a String in JavaScript
- How to Convert Array to String in JavaScript
- How to Check String Equality in JavaScript
- How to Filter String in JavaScript