How to Compare Objects in JavaScript
-
Compare Objects Using the
JSON.stringify()
Function in JavaScript - Compare Objects Manually in JavaScript
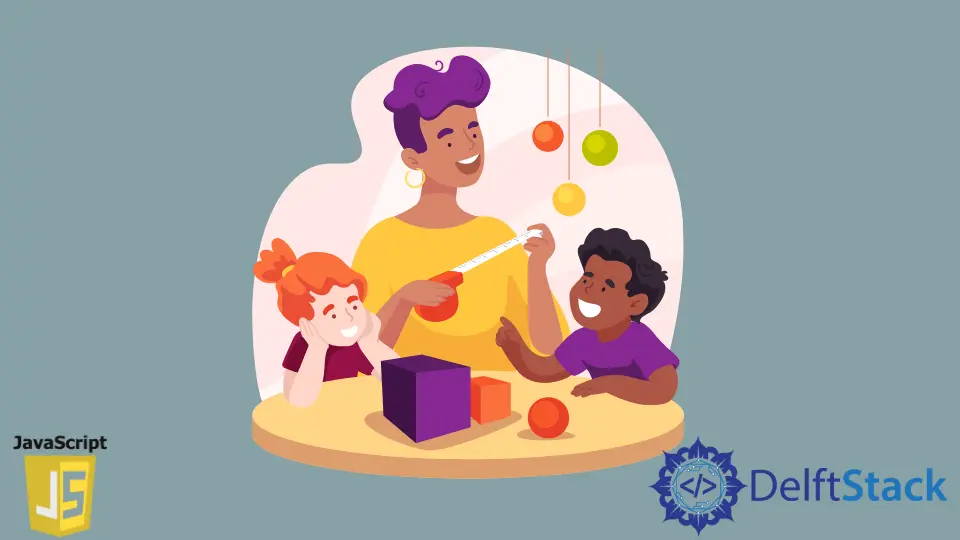
This tutorial will discuss how to compare objects manually or by using the JSON.stringify()
function in JavaScript.
Compare Objects Using the JSON.stringify()
Function in JavaScript
Comparing variables in JavaScript is relatively easy; we just need to use the comparison operator ===
between two variables. The variables can be of any data type, like strings and integers.
The comparison operator returns a boolean, which can be true
or false
depending on the variables being compared. For example, let’s compare two variables of a string type using the comparison operator.
See the code below.
var a = 'Apple';
var b = 'Apple';
console.log(a === b)
Output:
true
In the output, the boolean true
indicates that the two variables are identical. Similarly, you can also compare numbers. Now let’s talk about the comparison of objects in JavaScript.
Object comparison is not easy because they contain multiple values, and they can also contain objects inside them. The fast and easy method to compare two objects is to use the JASON.stringify()
function. The disadvantage of this method is the order of the properties matters in comparison.
This function compares the first property of one object with the first property of the other object, and so on. If we change the order of the properties in one of the objects, this method will not work. For example, let’s compare two objects using the JSON.strigify()
function.
See the code below.
const fruit1 = {
name: 'Apple',
price: '2'
};
const fruit2 = {
name: 'Apple',
price: '2'
};
console.log(JSON.stringify(fruit1) === JSON.stringify(fruit2))
Output:
true
In this code, we are comparing two objects containing two properties each. From the output, the two objects are equal. Now, let’s change the order of the two properties in one object and then compare it with the other using the JSON.stringify()
function.
See the code below.
const fruit1 = {
name: 'Apple',
price: '2'
};
const fruit2 = {
price: '2',
name: 'Apple'
};
console.log(JSON.stringify(fruit1) === JSON.stringify(fruit2))
Output:
false
In the output, the result is false
. We have changed the order of the properties of the second object; this is the disadvantage of this method.
Compare Objects Manually in JavaScript
The method above has a disadvantage, so we can make our own function to compare two objects so that we don’t have to care about the order of the properties of the objects.
For example, let’s make a function to compare the above objects in JavaScript. See the code below.
function ObjCompare(obj1, obj2) {
if (obj1.name === obj2.name && obj1.price === obj2.price) {
return true;
};
return false;
}
const fruit1 = {
name: 'Apple',
price: '2'
};
const fruit2 = {
price: '2',
name: 'Apple'
};
console.log(ObjCompare(fruit1, fruit2))
Output:
true
In the code above, we made the ObjCompare()
function, comparing two objects according to their properties regardless of their order. The function returns true
only when the name and the price of the two objects are the same; otherwise, it will return false
.
You can also make your own function to compare multiple properties or compare one property you like to compare from all the available properties. You can also make another function to compare more than two objects at a time.
Now, consider we have two objects with many properties, and we want to compare all those properties. Making this kind of function will take a lot of time.
Instead, we can use the Object.keys()
function to get all the keys or properties of a function and compare them using a loop. If all the properties are the same, the function will return true
; otherwise, it will return false
.
For example, let’s make this function and test it with the two objects above. See the code below.
function ObjCompare(obj1, obj2) {
const Obj1_keys = Object.keys(obj1);
const Obj2_keys = Object.keys(obj2);
if (Obj1_keys.length !== Obj2_keys.length) {
return false;
}
for (let k of Obj1_keys) {
if (obj1[k] !== obj2[k]) {
return false;
}
}
return true;
}
const fruit1 = {
name: 'Apple',
price: '2',
color: 'red'
};
const fruit2 = {
price: '2',
name: 'Apple',
color: 'red'
};
console.log(ObjCompare(fruit1, fruit2))
Output:
true
In this program, the first if
statement inside the ObjCompare()
function is used to check the length of properties or keys inside the two objects.
If they are not equal, the function will return false
and will not move to the loop. This method works fine even if we change the properties’ order or the keys of any of the objects. You can also change this function to compare three or more objects at a time.
Related Article - JavaScript Object
- How to Search Objects From an Array in JavaScript
- How to Get the Object's Value by a Reference to the Key
- How to Find Object in Array by Property Value in JavaScript
- How to Print Objects in JavaScript
- How to Destroy Object in JavaScript
- Nested Objects in JavaScriptn