How to Concatenate String and Integer in JavaScript
- Concatenate String and Integer Using Template String in JavaScript
-
Concatenate String and Integer Using the
+
Operator in JavaScript -
Concatenate String and Integer Using the
String()
Constructor in JavaScript -
Concatenate String and Integer Using
toString()
Method in JavaScript - Conclusion
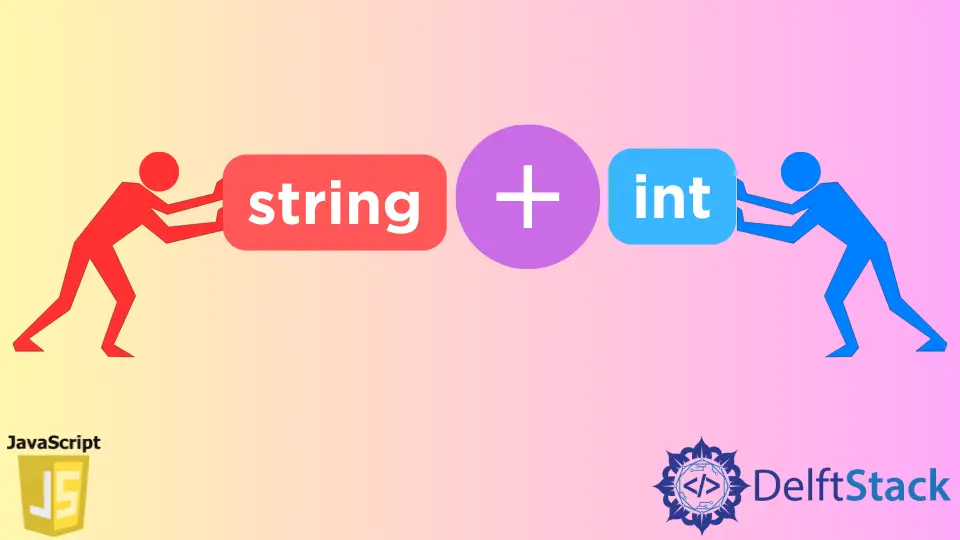
Whenever we develop web applications in JavaScript, we always encounter a situation where we want to combine a variable of any type (integer, float, etc.) with a string. This process, known as concatenation, allows you to create dynamic and informative output.
In this article, we will explore various methods to concatenate strings and integers in JavaScript. Each approach offers its advantages, providing you with a toolkit to seamlessly merge text and numerical data.
Concatenate String and Integer Using Template String in JavaScript
Template strings, also known as template literals, were introduced in ECMAScript 6 (ES6) to provide a more flexible and readable way to work with strings. They are denoted by backticks (``
) and allow for the embedding of expressions within strings.
The template string in JavaScript allows you to add variables of any type within the given string value. To use this feature, we have to enclose our string inside the backtick characters.
We don’t use the traditional double-quotes operator (" "
) here.
Here is the basic syntax:
let variableName = `Your template string goes here with ${placeholders}`;
In a template string, ${}
is used to insert expressions or variables. These will be evaluated and replaced with their respective values in the resulting string.
Let’s say we have a string and an integer variable value
with a value of 8
, as shown below. We aim to concatenate the value of the variable value
with the string and then print it on the console.
Code Snippet - JavaScript:
let value = 8;
console.log("Number is the value.");
Output:
Number is the value.
To do this, first, we have to replace the double quotes (" "
) of the string with the backtick characters (``
).
After enclosing the string with backticks, we can concatenate or insert the variable value
anywhere within the string. For this, we have to use the ${}
, and inside this, we add the variable ${value}
, as shown below.
Example 1: Basic Example
let value = 8;
console.log(`Number ${value} is the value.`);
Output:
Number 8 is the value.
As you can see, the value 8
is printed within the string. Whenever we want to concatenate a string with a variable, we use the concept of string literals.
Similarly, you can concatenate many variables having any type in the string with this feature.
Example 2: Two variables
let name = "John";
let age = 30;
console.log(`Hello, my name is ${name} and I am ${age} years old.`);
In the example above, we define a template string with backticks.
The variables name
and age
are then embedded within the string using ${}
. This allows us to seamlessly combine strings and variables to create dynamic output.
Output:
Hello, my name is John and I am 30 years old.
Example 3: Handling Expressions
Template strings also allow for the inclusion of expressions within ${}
. This enables more complex operations to be performed while concatenating strings and integers.
let quantity = 3;
let unitPrice = 49.99;
let totalPrice = `Total Price: $${(quantity * unitPrice).toFixed(2)}`;
console.log(totalPrice);
In this example, we calculate the totalPrice
by multiplying the quantity
and unitPrice
. The result is then formatted to two decimal places using toFixed(2)
before being embedded in the template string.
Output:
Total Price: $149.97
Example 4: Handling Functions
Template strings provide the flexibility to include function calls, allowing for dynamic data manipulation during concatenation.
function calculateTax(price, taxRate) {
return (price * (1 + taxRate)).toFixed(2);
}
let itemPrice = 99.99;
let taxRate = 0.1;
console.log(`Total Price (including tax): $${calculateTax(itemPrice, taxRate)}`);
Here, the calculateTax
function takes the itemPrice
and taxRate
as arguments, calculates the total price, including tax, and returns it. This result is then embedded in the template string.
Output:
Total Price (including tax): $109.99
Concatenate String and Integer Using the +
Operator in JavaScript
The +
operator in JavaScript serves a dual purpose: it is used for both arithmetic addition and string concatenation. When applied to strings, it combines them into a single string, and when applied to numbers, it performs addition.
The +
operator can also concatenate a string with an integer. We will take the same example that we have seen above, but we will use the +
operator instead of a template string.
Example 1: Basic example
let value = 8;
console.log("Number " + value + " is the value.");
Output:
Number 8 is the value.
Here, we have to use the traditional double quotes on a string, and at whichever position we want to concatenate the integer variable value
with the string, we first have to close the double quotes. Then, we use a +
operator before and after the variable value
.
After this, we can again continue with the remaining string with the help of double quotes, as shown above.
The only issue while using the +
operator is that if you want to concatenate many variables between the string, you need to separate the string by using multiple +
operators, as shown below.
If there are many variables that we want to concatenate with the string, it might create issues while reading and understanding the code.
Example 2: Multiple variables
let value1 = 8;
let value2 = 16;
let value3 = 24;
console.log("Table of " + value1 + " has values " + value2 + " and " + value3);
Output:
Table of 8 has values 16 and 24
Therefore, it’s always recommended that you use the template literals feature of ES6 as much as possible. And if there are very few variables that need to be concatenated with the string, then, in that case, you might use the +
operator.
Example 3: Handling Expressions
The +
operator can also be used to concatenate expressions involving both strings and integers.
let apples = 5;
let oranges = 3;
let totalFruit = "Total fruit count: " + (apples + oranges);
console.log(totalFruit);
Here, we have two variables, apples
and oranges
, both containing integers. The expression (apples + oranges)
adds the two numbers together, resulting in 8
.
This sum is then concatenated with the string "Total fruit count: "
, forming the variable totalFruit
, which will hold the string "Total fruit count: 8"
.
Output:
Total fruit count: 8
Example 4: Handling Functions
The +
operator can also be used to concatenate the result of a function with a string.
function greet(name) {
return "Hello, " + name + "!";
}
let userName = "Alice";
let greeting = greet(userName);
console.log(greeting);
In this example, we have a greet
function that takes a name
parameter and returns a greeting message. The +
operator is used to concatenate the result of calling the function with the string "Hello, "
. This allows for dynamic generation of messages based on function outputs.
Output:
Hello, Alice!
Concatenate String and Integer Using the String()
Constructor in JavaScript
The String()
constructor in JavaScript is used to create a new string object. When called with a value, it converts that value to its string representation.
Here is the basic syntax:
let stringVariable = String(value);
Here, value
is the value you want to convert to a string. It can be a variable, a literal, or an expression.
To concatenate a string and an integer using the String()
constructor, we first convert the integer to its string representation and then concatenate it with the desired string.
Example 1: Basic example
let age = 30;
let message = "I am " + String(age) + " years old.";
console.log(message);
Here, the variable age
contains the integer 30
. By using the String()
constructor, we convert it to a string before concatenating it with the strings "I am "
and " years old."
. The resulting message
will be "I am 30 years old."
.
Output:
I am 30 years old.
Example 2: Handling Expressions
The String()
constructor can also be used in conjunction with expressions involving both strings and integers.
let apples = 5;
let oranges = 3;
let totalFruit = "Total fruit count: " + String(apples + oranges);
console.log(totalFruit);
In this example, we have two variables, apples
and oranges
, both containing integers. The expression (apples + oranges)
adds the two numbers together, resulting in 8
.
This sum is then converted to a string using the String()
constructor and concatenated with the string "Total fruit count: "
, forming the variable totalFruit
, which will hold the string "Total fruit count: 8"
.
Output:
Total fruit count: 8
Example 3: Handling Functions
The String()
constructor can also be used in conjunction with function outputs to concatenate strings and integers.
function greet(name) {
return "Hello, " + String(name) + "!";
}
let userName = "Bob";
let greeting = greet(userName);
console.log(greeting);
In this example, the greet
function takes a name
parameter and returns a greeting message. The String()
constructor is used to convert the name
parameter to a string before concatenating it with the strings "Hello, "
and "!"
.
This allows for dynamic generation of messages based on function outputs.
Output:
Hello, Bob!
Concatenate String and Integer Using toString()
Method in JavaScript
The toString()
method in JavaScript is used to convert a value to its string representation. It can be used on various types, including numbers, Booleans, objects, arrays, and more.
Here’s the syntax for using toString()
:
value.toString()
Here, value
is the variable or value that you want to convert to a string. When you call toString()
on a value, it returns the string representation of that value.
To concatenate a string and an integer using the toString()
method, we first convert the integer to a string using this method. Once both values are in string form, they can be concatenated using the +
operator.
Example 1: Basic example
let age = 30;
let message = "I am " + age.toString() + " years old.";
console.log(message);
In this example, the variable age
contains the integer 30
. We apply the toString()
method to convert it to the string "30"
.
The resulting string is then concatenated with the strings "I am "
and " years old."
using the +
operator. This forms the variable message
, which will hold the string "I am 30 years old."
.
Output:
I am 30 years old.
Example 2: Handling Expressions
The toString()
method can also be used to concatenate expressions involving both strings and integers.
let apples = 5;
let oranges = 3;
let totalFruit = "Total fruit count: " + (apples + oranges).toString();
console.log(totalFruit);
In this example, we have two variables, apples
and oranges
, both containing integers. The expression (apples + oranges)
adds the two numbers together, resulting in 8
.
We then apply the toString()
method to convert it to the string "8"
. This string is then concatenated with the string "Total fruit count: "
using the +
operator, forming the variable totalFruit
, which will hold the string "Total fruit count: 8"
.
Output:
Total fruit count: 8
Example 3: Handling Functions
The toString()
method can also be used to concatenate the result of a function with a string.
function greet(name) {
return "Hello, " + name + "!";
}
let userName = "Bob";
let greeting = greet(userName).toString();
console.log(greeting);
In this example, we have a greet
function that takes a name
parameter and returns a greeting message. The +
operator is used to concatenate the result of calling the function with the string "Hello, "
.
We then apply the toString()
method to convert the resulting value to a string. This allows for dynamic generation of messages based on function outputs.
Output:
Hello, Bob!
Conclusion
In this article, we explored various methods to concatenate strings and integers in JavaScript. Each approach offers its advantages, providing you with a toolkit to seamlessly merge text and numerical data.
First, the Template String (Template Literal) allows for dynamic string generation by embedding expressions or variables within backticks. It provides a more readable and flexible way to work with strings.
Then, the +
operator serves a dual purpose in JavaScript, both for arithmetic addition and string concatenation. It can be used to combine strings and integers but may become less readable when dealing with multiple variables.
Next, the String()
constructor converts a value to its string representation. By applying it to integers before concatenation, you can seamlessly merge them with strings.
And lastly, the toString()
Method converts a value to its corresponding string representation. It can be used on various data types, including numbers. By using this method, you can convert integers to strings before concatenation.
Sahil is a full-stack developer who loves to build software. He likes to share his knowledge by writing technical articles and helping clients by working with them as freelance software engineer and technical writer on Upwork.
LinkedIn