How to Compress String in JavaScript
-
Use
js-string-compression
to Compress String in JavaScript - Use the LZString Library to Compress String in JavaScript
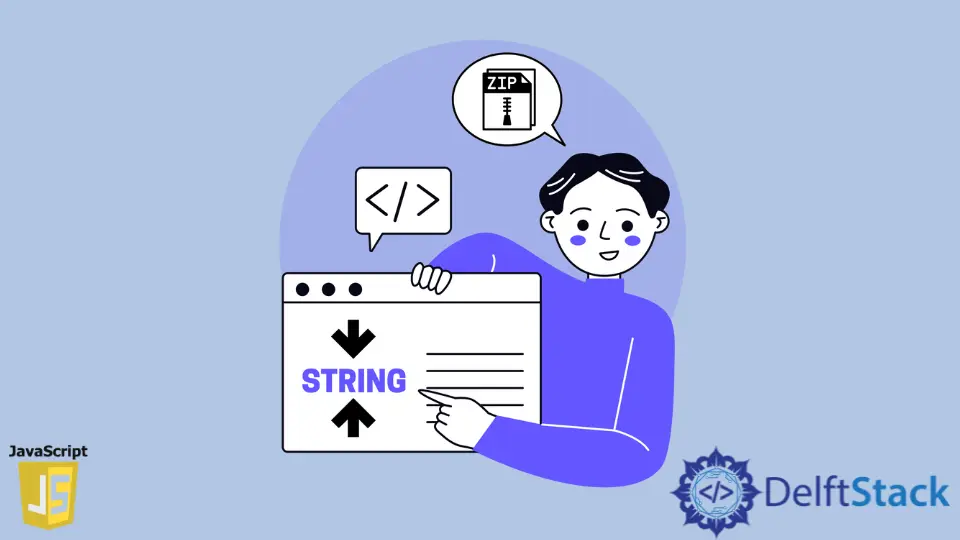
In JavaScript, there can be a wide range of compression, the compression of files like gzip, and many more. Here, we will discuss two ways of compressing a string.
Initially, we will highlight the Huffman algorithm. And later, we will cover the LZString way of solving the task.
Use js-string-compression
to Compress String in JavaScript
We will first set up a folder consisting of a file (okay.js
). We are using VSCode for the code editor, and in its terminal, we will write the following command.
npm i js-string-compression
This will add the necessary package needed to implement the Huffman algorithm. Once the packages are installed, you will have a package.json
and package-lock.json
file in the root directory.
The package.json
should look similar to the following.
{
"dependencies": {
"js-string-compression": "^1.0.1"
}
}
In the next stage, we will write our basic code, defining a string and setting the parameters that we want to check. Let’s check the code lines.
Code Snippet:
var jsscompress = require('js-string-compression');
var raw_text =
'Lorem Ipsum is simply dummy text of the printing and typesetting industry.';
var hm = new jsscompress.Hauffman();
var compressed = hm.compress(raw_text);
console.log('before compressed: ' + raw_text);
console.log('length: ' + raw_text.length);
console.log('after compressed: ' + compressed);
console.log('length: ' + compressed.length);
console.log('decompressed: ' + hm.decompress(compressed));
Output:
Use the LZString Library to Compress String in JavaScript
For compressing your string via the LZString library, we need to have an HTML file and a js file. The LZString is a Perl implementation (lz-string
) to compress strings.
To import the dependencies, we can follow up the command line below in our terminal of the root directory, where we have our HTML and js files.
npm install -g lz-string
Next, we will create an HTML file named new.html
and a new.js
file. We will also create another file called lz-string.js
, where we will store the LZString implementation.
For the full code, we considered this repository.
Now, we will write some lines in our new.js
, and the preview is similar to the below.
Code Snippet:
var string =
'Lorem Ipsum is simply dummy text of the printing and typesetting industry.';
console.log('Size of sample is: ' + string.length);
var compressed = LZString.compress(string);
console.log('Size of compressed sample is: ' + compressed.length);
console.log(compressed);
string = LZString.decompress(compressed);
console.log('Sample is: ' + string);
After prepping the new.js
file, we will import the lz-string.js
and new.js
to the new.html
file. When we open the HTML file in the browser, the output would be similar to the one below.
Output:
As it can be noticed, if we compare both of the solutions, the LZString implementation gives a better output. It was dedicatedly evolved and designed to solve issues in larger cases where you can store huge-sized data in server storage.