How to Append to String in JavaScript
-
Append a String to Another String Using the
concat()
Function in JavaScript -
Append a String to Another String Using the Append Operator
+
in JavaScript
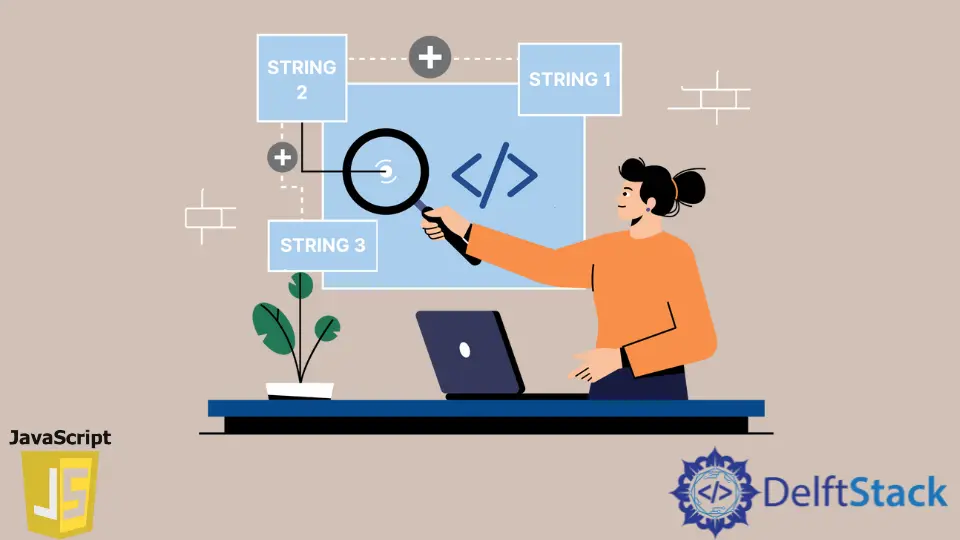
This tutorial will discuss how to append a string to another string using the concat()
function and append operator +
in JavaScript.
Append a String to Another String Using the concat()
Function in JavaScript
To append one string with another string, we can use the predefined concatenation function concat()
in JavaScript. This function concatenates two given strings together and returns the one string as output. For example, let’s define two strings and join the first string with the second string using the concat()
function. See the code below.
var a = 'abcd';
var b = 'efg';
a = a.concat(b);
console.log(a);
Output:
abcdefg
As you can see in the output, the second string is concatenated with the first string, and the result is stored in the first string. If you want to create a new string from the combination of two given strings, you need to store the result of concatenation in a new variable.
Append a String to Another String Using the Append Operator +
in JavaScript
To append one string with another string, we can also use the append operator +
in JavaScript. See the code below.
var a = 'abcd';
var b = 'efg';
a += b;
console.log(a);
Output:
abcdefg
If you want to create a new string from the combination of two given strings, you have to store the result of concatenation in a new variable, as shown below.
var c = a + b;
The result of both the above methods is the same, but the difference is in the performance of these methods. The performance append operator +
is better than the concat()
function.