JavaScript String.concat() Method
-
Syntax of JavaScript
string.concat()
: -
Example Codes: Join Two String Using JavaScript
String concat()
Method -
Example Codes: Join multiple strings With JavaScript
string.concat()
Method -
Example Codes: Join Strings With Commas using JavaScript
String concat()
Method
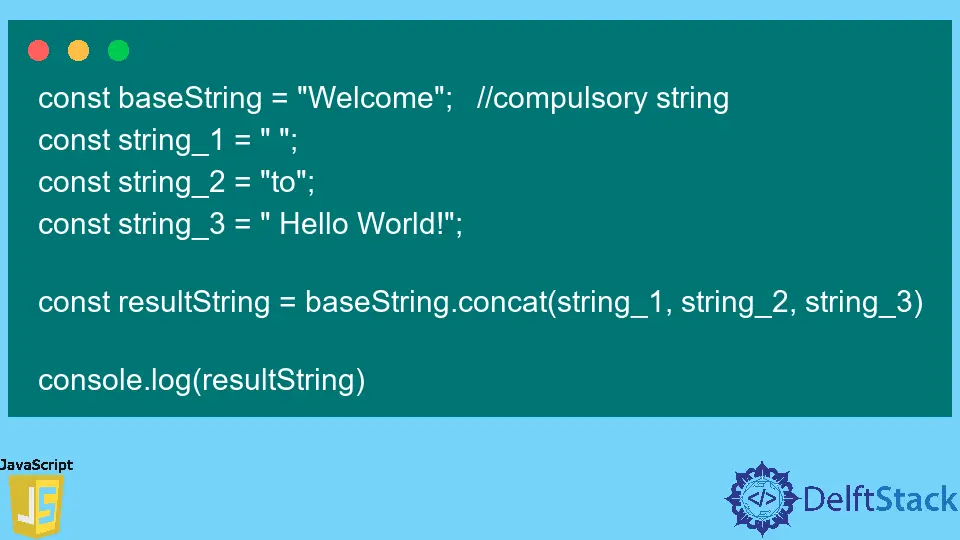
The String concat()
is a built-in method in JavaScript that concatenates two or more strings at the end of another without altering any strings.
Syntax of JavaScript string.concat()
:
baseString.concat(string_1, string_2, ..., string_N);
Parameters
baseString |
This is a compulsory string type calling object |
string_1 |
The string to be concatenated with base string |
string_N |
N is the number of strings to be concatenated |
Return
A new resultant string is returned after joining the strings in the same order as strings passed in the parameter.
Example Codes: Join Two String Using JavaScript String concat()
Method
Let’s start with a simple example where JavaScript String concat()
method combines two strings and prints the result on the console.
const baseString= "I am a ";
const string_1= "programmer.";
console.log(baseString.concat(string_1))
Output:
I am a programmer.
A new string is created when the String concat()
method is called. The characters in the string object baseString
on which the concat()
method is called will first populate this new array.
Then the characters of string_1
are concatenated at the end of the new string without changing two strings.
Example Codes: Join multiple strings With JavaScript string.concat()
Method
In this example, the JavaScript concat()
method combines multiple strings and returns the concatenated four strings in a new array - resultString
.
const baseString = "Welcome"; //compulsory string
const string_1 = " ";
const string_2 = "to";
const string_3 = " Hello World!";
const resultString = baseString.concat(string_1, string_2, string_3)
console.log(resultString)
Output:
Welcome to Hello World!
In the above example, concat()
makes a new array. The elements of each parameter string are then concatenated end to end into the new array.
The new array is copied to a const string object, resultString
.
Example Codes: Join Strings With Commas using JavaScript String concat()
Method
The below example joins strings with a comma in-between using concat()
and prints the result on the console.
var s_1 = "Roses"
var s_2 = "sunflowers"
var s_3 = "and lilies."
console.log(s_1.concat( ", ", s_2, ", ", s_3))
Output:
Roses, sunflowers, and lilies.
The string ","
is initialized when the string concat()
function is called.