How to Append Elements in an Array in JavaScript
-
Use the Array’s
.length
Property to Append Elements in an Array in JavaScript -
Use the
push()
Method to Append Elements in an Array in JavaScript -
Use the
unshift()
Method to Append Elements in an Array -
Use the
splice()
Method to Append Elements in an Array -
Use the
concat()
Method to Append Elements in an Array -
Use the
spread
Syntax to Append Elements in an Array
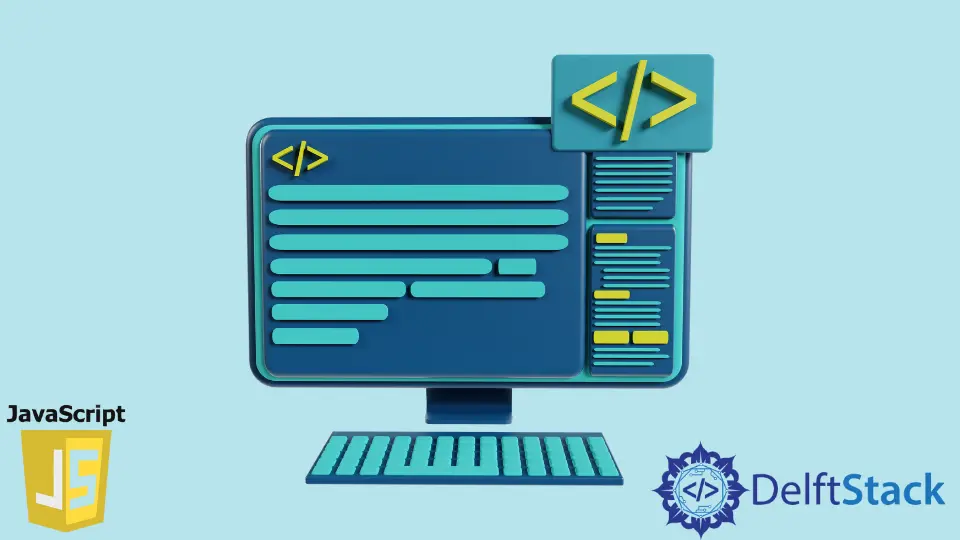
In this article, we will learn how to append elements in an array in JavaScript.
There are several methods to append an element to an array in JavaScript. We can append a single element, multiple elements, and even append a whole array to a given array. We can use a method depending on whether we want to mutate or not, speed and efficiency requirements, readability of the code.
Use the Array’s .length
Property to Append Elements in an Array in JavaScript
It is the classical approach in which we get the last empty index of the array using the total length of the array and insert an element at that index. This method is the easiest to use and provides excellent efficiency. It allows us only to append one element at a time. It is a mutative method because it changes the original array.
let arr = [0, 1, 2];
arr[arr.length] = 3;
console.log(arr);
Output:
[0, 1, 2, 3]
In the above code, we get the last index of the array using arr.length
as 3
and then add elements at those indexes.
Use the push()
Method to Append Elements in an Array in JavaScript
The push()
method is used to add elements to the end of the array. We can add a single element, multiple elements, or even an array. It is the simplest and one of the quickest options, it even beats the above method using Array.length
in large arrays in some cases. The actions performed by .push()
can be reversed by .pop()
method. When multiple elements are passed together, their order is preserved by the push()
operator. This method also changes the array, and hence it is mutative.
const arr = [1, 2, 3];
const arr2 = [8, 9, 10];
arr.push(4); // single item
arr.push(5, 6, 7); // multiple items
arr.push(...arr2); // spread operator
console.log(arr);
Output:
[1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
In the above code, we have demonstrated all 3
cases: adding a single element, multiple elements, and even a whole array using the spread syntax. All the elements are appended at the end of the array.
Use the unshift()
Method to Append Elements in an Array
The unshift()
method helps us add an element to the array’s beginning. It returns the new length of the array. It can be called or applied to objects resembling an array in properties. When multiple elements are passed together, their order is preserved by the unshift()
operator. This method also changes the array, and hence it is mutative.
const arr = [1, 2, 3];
const arr2 = [8, 9, 10];
arr.unshift(4); // single item
arr.unshift(5, 6, 7); // multiple items
arr.unshift(...arr2) // spread operator
console.log(arr);
Output:
[8, 9, 10, 5, 6, 7, 4, 1, 2, 3]
In the above code, we have demonstrated all 3 cases: adding a single element, multiple elements, and even a whole array using spread syntax. Notice how this operation is different from the push()
, all the elements are appended to the beginning of the array.
Use the splice()
Method to Append Elements in an Array
The splice()
method can modify the array’s content by adding/removing elements. It takes the following 3
arguments :
index
: An integer value specifying the position to add/remove elements. We can even specify an index from the back of the array by using negative indices.howmany
: It is an optional parameter. It specifies how many items are to be removed from the array. If it is set to0
then no items are removed.item1, item2, ... ,itemx
: The items to be added to the array.
This method also changes the array, and hence it is mutative.
const arr = [1, 2, 3, 4, 5];
arr.splice(4, 3, 7, 8, 9);
console.log(arr);
Output:
[1, 2, 3, 4, 7, 8, 9]
In the above code, we selected index 4
and added 3
elements 7,8,9
at that index.
Use the concat()
Method to Append Elements in an Array
The concat()
method takes arrays as input and concatenates them together i.e. it takes one array and append the rest to its end. But this operator does not modify the original array and returns an entirely new array containing the combined result. It can take two or more arrays and concatenate them together. Since this method does not modify the given array, it is non-mutative.
const arr = [1, 2, 3];
const arr2 = [8, 9, 10];
const arr3 = arr.concat(arr2);
console.log(arr3);
Output:
[1, 2, 3, 8, 9, 10]
In the above code, we take two arrays, arr
and arr2
, and call the concat()
function to concatenate them to form the new array - arr3
.
Use the spread
Syntax to Append Elements in an Array
We can use the spread syntax to append complete arrays to a given array. It is a non-mutative method as we just spread the array elements into a new array. It is very similar to the concat()
operation. It helps us to create a copy or merge two separated arrays. It is important to use the spread syntax carefully. If we use the syntax const arr = [arr1, arr2];
we get a nested array containing two subarrays whereas if we use const arr=[...arr1 , ...arr2];
we concatenate the elements of both arrays together.
const arr = [1, 2, 3];
const arr2 = [8, 9, 10];
const arr3 = [...arr, ...arr2];
Output:
[1, 2, 3, 8, 9, 10]
In the above code, we have used the spread operator to append two arrays, arr
and arr2
, into a new array - arr3
.
All the above methods are supported by all the major browsers except the spread syntax. Internet Explorer does not support the spread syntax.
Harshit Jindal has done his Bachelors in Computer Science Engineering(2021) from DTU. He has always been a problem solver and now turned that into his profession. Currently working at M365 Cloud Security team(Torus) on Cloud Security Services and Datacenter Buildout Automation.
LinkedInRelated Article - JavaScript Array
- How to Check if Array Contains Value in JavaScript
- How to Create Array of Specific Length in JavaScript
- How to Convert Array to String in JavaScript
- How to Remove First Element From an Array in JavaScript
- How to Search Objects From an Array in JavaScript
- How to Convert Arguments to an Array in JavaScript