How to Check if a Character in a String Is Uppercase or Not in JavaScript
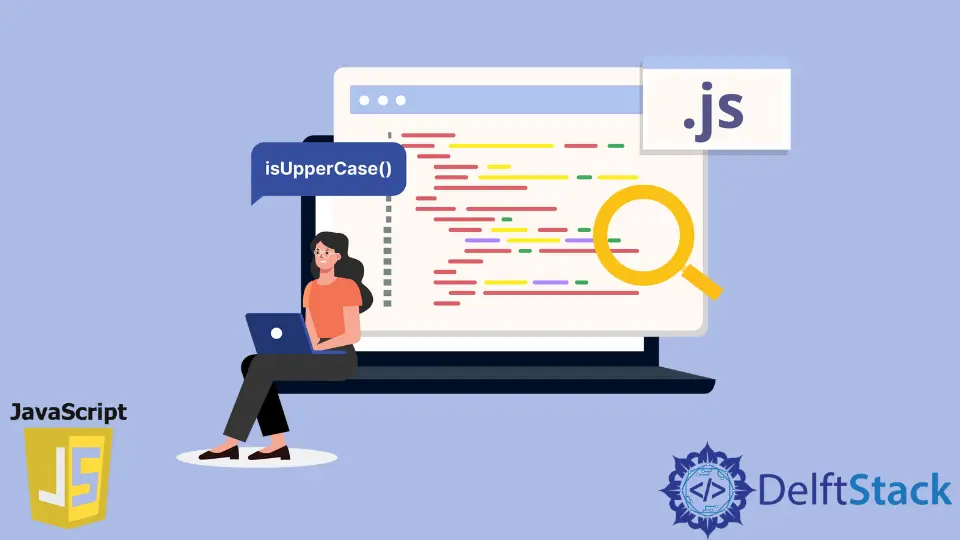
In this tutorial, we will demonstrate how to check if a given string containing various characters has all the characters in an uppercase format or not.
Check if a Character in a String Is Uppercase or Not in JavaScript
In JavaScript, there is no built-in function to check if every character in a given string is in uppercase format or not. So, we have to implement our function.
Here, we will create a function called isUpperCase()
, an anonymous arrow function, to tell us whether all the characters in a string are in uppercase. If so, it prints a message in the console.
If any of the characters in a string are not in the uppercase format, it will print them on the console window. The isUpperCase()
function will take a single parameter providedStr
as the input.
Before writing the logic for the isUpperCase()
function, let us first declare some of the variables. First, we will declare two string variables, str_1
and str_2
, containing some string values.
We will pass both these variables to the isUpperCase()
function as a parameter for checking the uppercase format.
Code snippet:
let str_1 = 'AB% ^M';
let str_2 = 'IO(|12c';
let allUpperCase = true;
let isUpperCase =
providedStr => {
for (i in providedStr) {
if (providedStr[i] !== providedStr[i].toUpperCase()) {
allUpperCase = false;
console.log(`Letter ${providedStr[i]} is not uppercase.`);
}
}
if (allUpperCase) {
console.log('All characters in a given string are uppercase...')
}
}
isUpperCase(str_1);
isUpperCase(str_2);
Then, we will declare another variable called the allUpperCase
, a boolean variable. By default, we will assign its value to True.
We assume that all the characters in the provided strings are already in the uppercase format.
Inside the isUpperCase()
function, we will apply a for...in
loop on the providedStr
variable. At every iteration, this for...in
loop will give us the index i
of every character of the string.
Now that we have the index of the individual character of the string, we can take this i
variable and access that character using providedStr[i]
. Here, we will take the character as it is from the string using the providedStr[i]
and then compare it with providedStr[i].toUpperCase()
.
The toUpperCase()
function will make that character to uppercase. So, if the character is already in the uppercase format, it will not do anything.
The if
statement will not be executed if the character is already in the uppercase format. If any character in the string is not in the uppercase format, the program will go inside the if
statement.
It will make the variable allUpperCase
false, which means that we have found a character, not in uppercase format, and then we will print that character on the console window.
After iterating all the characters in a string, we will perform a conditional check to know if the value inside the allUpperCase
variable is true or not. If it is true
, all the characters in the string are in uppercase format.
Output:
Here, since our first-string variable str_1
contains all the uppercase values AB% ^M
. Therefore, it has printed all the characters are in uppercase.
Note that there is a space character inside this string as well. Inside the second string variable str_2
, from all the characters IO(|12c
, we only have character c
, which is not in uppercase format, so it is printing this character on the console window.
Sahil is a full-stack developer who loves to build software. He likes to share his knowledge by writing technical articles and helping clients by working with them as freelance software engineer and technical writer on Upwork.
LinkedIn