How to Get Key of a JavaScript Object
-
Get Key of a Javascript Object With the
Object.keys()
Method -
Get Keys of a Javascript Object With the
Object.entries(obj)
Method -
Get
key
of a Javascript Object Usingfor
Loop
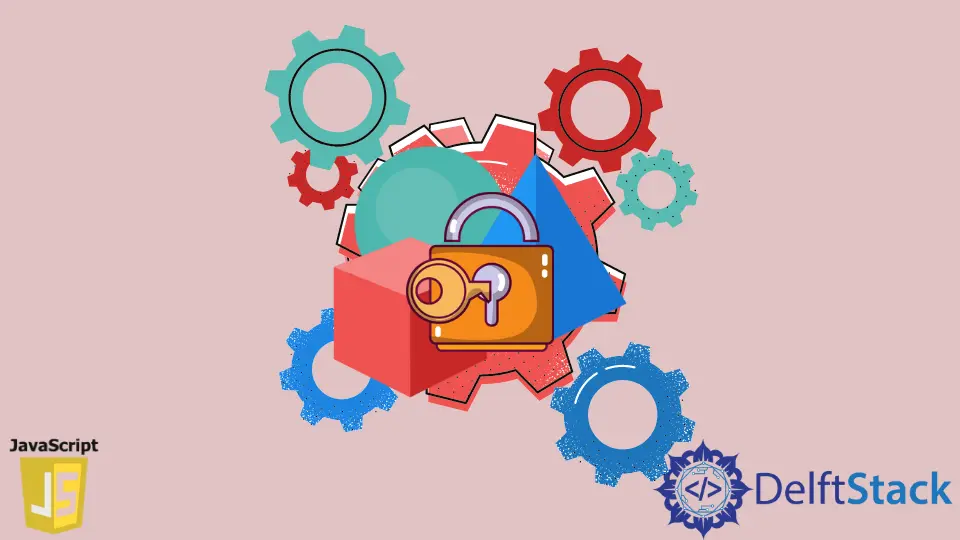
A javascript object is a collection of key-value pairs. We need a key to get its value from a javascript object. Key-value pairs are used widely in client-server communications and JavaScript programming. We are aware of retrieving a value from a JSON object provided we have its key. But what if we do not have the key
name? How do we get its value from the Javsscript object? Let us look at a few ways in which we can get the keys from a javascript object.
Get Key of a Javascript Object With the Object.keys()
Method
The Object.keys()
function returns an array containing the keys of the javascript object. We pass the javascript object as a parameter to the Object.keys()
function. The output array contains the keys in the same order in which they are in the original javascript object. If we pass an array to the Object.keys()
, it will return the array indices as output. And the parameter object is with indices, then the Object.keys()
will return an array of those indices. Refer to the following code to understand the usage and behavior of the Object.keys()
function.
var fruitsArr1 = ['Apple', 'Orange', 'Mango', 'Banana'];
var fruitsObj2 = {0: 'Apple', 4: 'Orange', 2: 'Mango', 3: 'Banana'};
var fruitsObj3 = {'id': '1', 'name': 'mango', 'color': 'yellow'};
console.log(Object.keys(fruitsArr1));
console.log(Object.keys(fruitsObj2));
console.log(Object.keys(fruitsObj3));
Output:
["0", "1", "2", "3"]
["0", "2", "3", "4"]
["id", "name", "color"]
If the keys are numbers, the Object.keys()
function will return an array of the numeric keys in sorted order. The fruitsObj2
has the keys numbered 0
, 4
, 2
, 3
. But when we apply the Object.Keys()
function, it returns the keys as ["0", "2", "3", "4"]
which is in a sorted order. The key-value will still hold the same value mapping as is it did for the original javascript object. For instance, the fruitsObj2
holds 4: "Orange"
and at 2: "Mango"
, but Object.keys(fruitsObj2)
returned their order as "2", "4"
. If we were console.log
their values at the keys 2
and 4
, we get the correct values as output. Hence, the function has not modified anything in the actual key-value mapping, even if the Object.keys
returns the numeric keys of an array or an object in sorted order. Refer to the following code.
console.log(fruitsObj2[2]);
console.log(fruitsObj2[4]);
Output:
Mango
Orange
Get Keys of a Javascript Object With the Object.entries(obj)
Method
Object.entries(obj)
method is diverse and is more flexible than the Object.keys()
function. It splits the entire object into small arrays. Each array consists of key-value pairs in the form [key, value]
. Using Object.keys()
, we get only the keys of an object, but using the Object.entries(obj)
, we can get all the entries in an object, including the keys
and their values
. Object.entries(obj)
is not a commonly used method. In most scenarios, we will require to get the keys from an object. The corresponding values can be obtained easily with the help of keys
.
Syntax
Object.entries(object)
Parameters
Same as that of the Object.keys()
method, the Object.entries(obj)
accepts the javascript object
as the parameter.
Return
Object.entries(obj)
returns the key-value pairs destructed into arrays. The return type will be an array of arrays, each sub-array holding two elements: the key and the value. Something like [[key1, value1], [key2, value2], [key3, value3] ... ]
. The function preserves the order of the object attributes. In the implementation behind the scenes, the key-value pairs are formed by iterating over the object properties. Refer to the following code to better understand the behavior of the Object.entries()
function. We use JSON.stringify()
to get a human-readable string version of the values output by the function.
var fruitsObj3 = {'id': '1', 'name': 'mango', 'color': 'yellow'};
console.log(JSON.stringify(Object.entries(fruitsObj3)));
Output:
"[["id","1"],["name","mango"],["color","yellow"]]"
We can use the Object.entries()
in another way. We can iterate over the javascript object and log the attribute keys and their values. The approach is as depicted by the following code snippet.
for (const [key, value] of Object.entries(fruitsObj3)) {
console.log(`${key}: ${value}`);
}
Output:
id: 1
name: mango
color: yellow
If we are interested in getting the key
alone from the Object.entries()
function, we can log the key
and discard the value part.
Get key
of a Javascript Object Using for
Loop
Can we use a simple for
loop to get the keys from an object? It is not a commonly known feature of a for
loop. We can iterate through any javascript object as we do for arrays using the for-in
combination. It iterates through each parameter with the i
(the iterator) holding the key
of the object and the object[i]
holding the value corresponding to the key
in the object. Let us look at the following code to understand the concept better.
var obj = {'id': '1', 'name': 'mango', 'color': 'green'};
for (let i in obj) {
console.log(i); // key
console.log(obj[i]); // value against the key
}
Output:
id
1
name
mango
color
green
If we wish to extract only the keys from the object, we can use the iterator value. We can use the console.log(i)
in the above code in the for(let i in obj)
block.
If we have nested structures in the javascript object, for(let i in obj)
can be used to get the key. But, the value for the nested structure will be the structure itself. Refer to the following code.
var a = {
'id': '1',
'name': 'mango',
'color': {'name': 'yellow', 'appearance': 'bright'}
};
for (let i in a) {
console.log(i);
console.log(a[i]);
}
Output:
id
1
name
mango
color
{name: "yellow", appearance: "bright"}