How to Get the Day of a Month in JavaScript
-
Use
getDate()
to Get the Day of a Month in JavaScript -
Use
getDay()
to Get the Day of a Month in JavaScript -
Use
getUTCDay()
to Get the Day of a Month in JavaScript
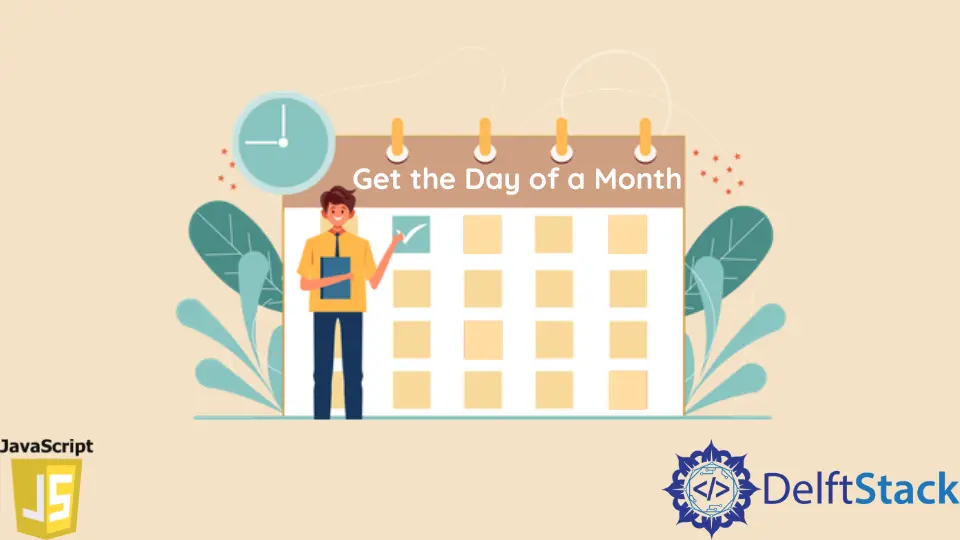
JavaScript has a built-in object called date
, which provides different methods for dates and times. We can use them to extract a day, month, year, time of a given date, or current time.
Date.now()
: Returns current date (ECMA Script).getDate()
: Returns the date ranges(1-31).getDay()
: Returns the day range(0-6).
A list of methods can be found here in MDN web docs.
Let’s initialize a date object that contains the current date. Say, the current day is February 07, 2022.
Code Snippet:
const date = new Date()
console.log(date)
Output:
Mon Feb 07 2022 00:19:29 GMT+0600 (Bangladesh Standard Time)
Use getDate()
to Get the Day of a Month in JavaScript
We will use the getDate()
function to get the day of the current month.
Code snippet:
console.log(date.getDate())
Output:
7
Use getDay()
to Get the Day of a Month in JavaScript
You can use getDay()
to get the day of the date. It returns a value from 0-6
respectively for Saturday to Friday.
We initialize a days
array object containing the week’s days. Instead of returning the value from 0-6
, it will return the name of the day of the week.
Code Snippet:
Const days = [
'Saturday', 'Sunday', 'Monday', 'Tuesday', 'Wednesday', 'Thursday', 'Friday'
]
console.log(days[date.getDay()])
Output:
Monday
Use getUTCDay()
to Get the Day of a Month in JavaScript
UTC methods are used more frequently. It returns values according to the UTC.
For example, in UTC, the method for extracting a day is getUTCDay()
. It also returns values from 0
to 6
. But here, 0
means "Sunday"
.
Example:
const date = new Date()
console.log('getDay() returns: ', date.getDay())
console.log('getUTCDay() returns: ', date.getUTCDay())
Output:
getDay() returns: 2
getUTCDay() returns: 1
If you notice, date.getDay()
returns 2
, meaning it’s Monday, but when we called the UTC method, it returned 1
. In UTC format, the 0th index of the day is Sunday.
Follow the next code snippet if you convert the above code segment in UTC format.
Code Snippet:
const date = new Date()
const days = [
' Sunday', ' Monday', ' Tuesday', ' Wednesday', ' Thursday', ' Friday',
' Saturday'
]
console.log(days[date.getDay()])
Output:
Sunday
For more documentation, visit here.