How to Display a JavaScript Variable in HTML Body
-
Use
innerHTML
to Display JavaScript Variable in the HTML Body -
Use
document.write()
Property to Display JavaScript Variable in HTML Body -
Use
window.alert
Property to Display JavaScript Variable in HTML Body
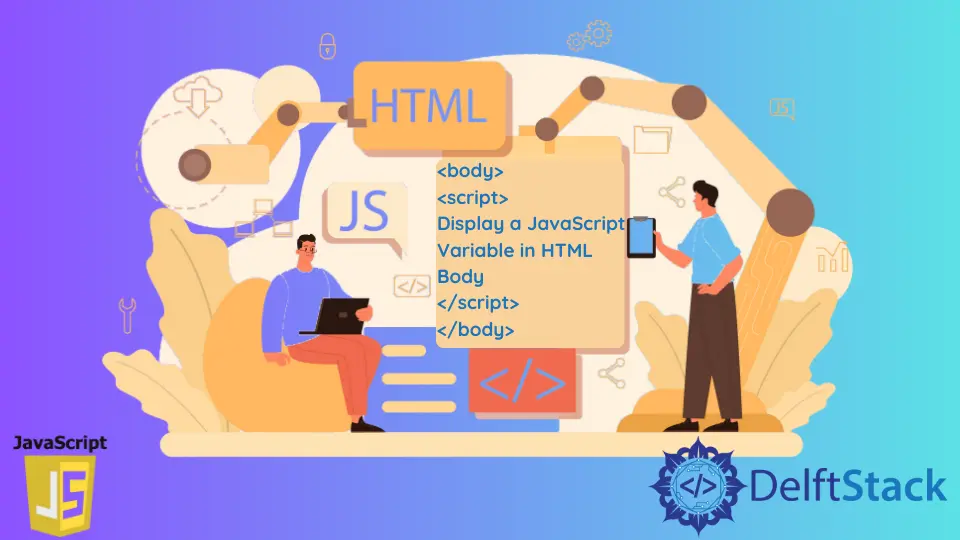
It is often easier to explicitly define a script
tag and add the code block for necessary output in an HTML body. We also use script
tags in the head
tag to load the code fence of JavaScript before the body
tag.
Every convention has its remarks; If we need to check if the code is bug-free, we can add JavaScript functions in the head
section to perform the task. We can also use JavaScript in the body
element for click events and other dynamic features.
Here, we will demonstrate how to display a JavaScript variable in the HTML body, and specifically, we will add our code blocks in the body
element. But there is a difference between the passing value in the HTML body and temporarily displaying a value triggered by JavaScript code conventions.
Use innerHTML
to Display JavaScript Variable in the HTML Body
The use of innerHTML
by fetching a specific id
or class
and passing the value of a variable is one of the most common ways to display a JavaScript variable in the HTML body.
We will initialize a span
tag in our HTML body with an id = "input"
. Then the script
tag will have a variable sent to that id = "input"
via the innerHTML
property. This DOM manipulation
is convenient when placing JavaScript variables in the HTML body.
Code Snippet:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<title>Test</title>
</head>
<body>
<p> Hello! I'm <span id="input"></span>.</p>
<script>
document.getElementById('input').innerHTML = "Robert" ;
</script>
</body>
</html>
Output:
Use document.write()
Property to Display JavaScript Variable in HTML Body
Here, the system to initialize the document.write(parameter)
property is mostly used for testing variables. In this case, the parameter
is the variable or object defined in JavaScript.
We will declare the variable in a script
tag before the segment to display it. Later, we will have a p
tag, and within it, another script
tag takes the upper script’s initiation.
Code Snippet:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<title>Test</title>
<script>
var input = "Gwen";
function doc(){
document.write(input);}
</script>
</head>
<body>
<p> Hello! I'm <script>doc(input)</script>.</p>
</body>
</html>
Output:
Use window.alert
Property to Display JavaScript Variable in HTML Body
This is a very easy process to test your variable via DOM manipulation
.
We will define a JavaScript object or variable and store a random value here. Inside the script
tag, we will declare the window.alert(variable)
, and this property will prompt a message as it triggers the window object.
Code Snippet:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<title>Test</title>
</head>
<body>
<script>
var input = "Hello! I'm Tom.";
window.alert(input);
</script>
</body>
</html>
Output:
Related Article - JavaScript Variable
- How to Access the Session Variable in JavaScript
- How to Check Undefined and Null Variable in JavaScript
- How to Mask Variable Value in JavaScript
- Why Global Variables Give Undefined Values in JavaScript
- How to Declare Multiple Variables in a Single Line in JavaScript
- How to Declare Multiple Variables in JavaScript