How to Load CSV to Array in JavaScript
-
Use the
jQuery-csv
Plugin to Load CSV to an Array in JavaScript - Create a User-Defined Function to Load CSV to an Array in JavaScript
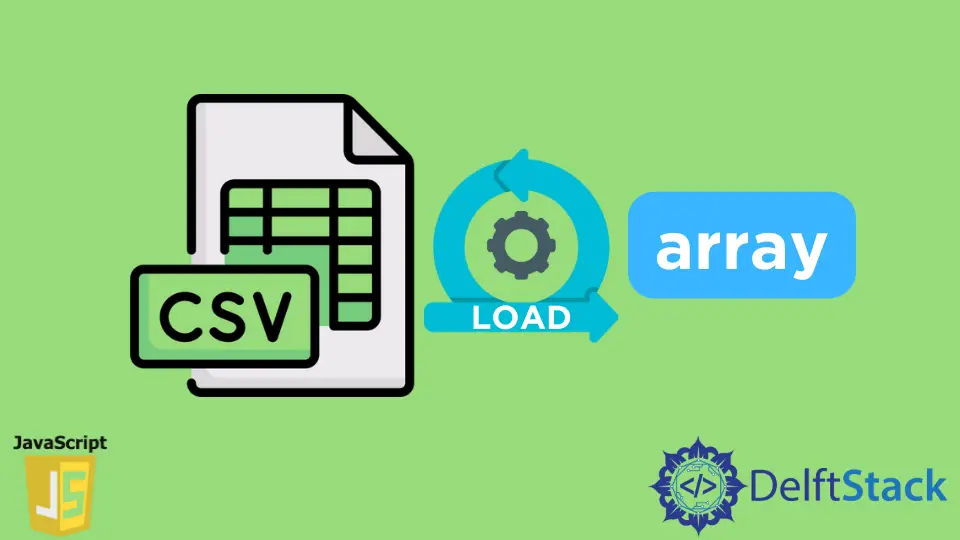
A CSV is a text file. It separates different values using a comma as a delimiter.
We will load a CSV file to an array using JavaScript in this article.
Use the jQuery-csv
Plugin to Load CSV to an Array in JavaScript
The jQuery library provides the jquery-csv
plugin that can read CSV files into arrays.
For example,
array = $.csv.toArrays(csv, {
delimiter: '\'',
separator: ';', // Sets a custom field separator character
});
We can set a custom delimiter character and a separator using the delimiter
and separator
properties. The final result is stored in an array.
Create a User-Defined Function to Load CSV to an Array in JavaScript
We will use the FileReader
class to read the required CSV file as a string. To store this into an array, we will use the slice()
, split()
, and map()
functions. The slice()
function helps return a new array with a portion of an old array on which it is implemented. The map()
function helps return a new array by providing the functions provided on every element in the array. The split()
functions help split the strings into substrings in the form of an array using a specified separator.
See the code below.
function csv_To_Array(str, delimiter = ',') {
const header_cols = str.slice(0, str.indexOf('\n')).split(delimiter);
const row_data = str.slice(str.indexOf('\n') + 1).split('\n');
const arr = row_data.map(function(row) {
const values = row.split(delimiter);
const el = header_cols.reduce(function(object, header, index) {
object[header] = values[index];
return object;
}, {});
return el;
});
// return the array
return arr;
}
We assume that the first line of the file contains the headers. The slice()
function starts from the beginning to the first \n
index and using the split()
function to split the string to an array based on the delimiter. Similarly, we use these two functions to store the rows. Next, we map the rows to an array. We use the map()
function to split each value of a row into an array. The reduce()
function is used with the headers to create an object, add the rows’ values with the necessary headers, and return this object. This object is added to the array. Finally, we return the final array, which is an array of objects.
Related Article - JavaScript Array
- How to Check if Array Contains Value in JavaScript
- How to Create Array of Specific Length in JavaScript
- How to Convert Array to String in JavaScript
- How to Remove First Element From an Array in JavaScript
- How to Search Objects From an Array in JavaScript
- How to Convert Arguments to an Array in JavaScript