How to Convert UTC to Local Time in JavaScript
- Method 1: Using the Date Object
- Method 2: Using toLocaleString()
- Method 3: Using Moment.js
- Method 4: Using Luxon
- Conclusion
- FAQ
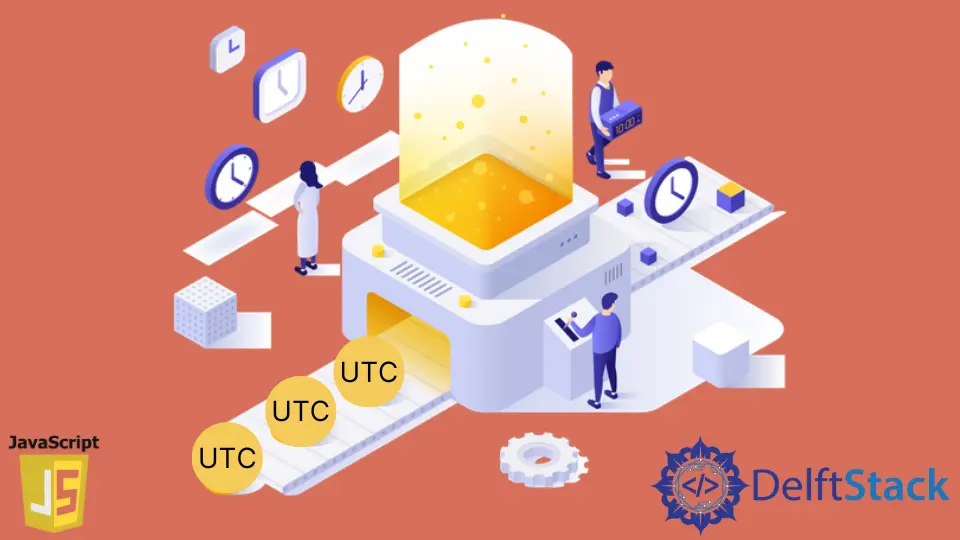
When working with dates and times in JavaScript, you may often encounter the need to convert Coordinated Universal Time (UTC) to your local time. This is especially common in applications that operate across different time zones. Understanding how to perform this conversion is crucial for displaying accurate timestamps to users.
In this tutorial, we will explore several effective methods to convert UTC to local time in JavaScript. By the end of this article, you’ll be equipped with the knowledge and code snippets necessary to handle time conversions seamlessly. Let’s dive in!
Method 1: Using the Date Object
One of the simplest ways to convert UTC to local time in JavaScript is by utilizing the built-in Date object. The Date object automatically handles time zone conversions based on the user’s local settings.
Here’s how you can do it:
const utcDate = new Date('2025-03-12T12:00:00Z');
const localDate = utcDate.toString();
console.log(localDate);
Output:
"Wed Mar 12 2025 13:00:00 GMT+0100 (Central European Standard Time)"
In this example, we first create a new Date object using a UTC date string. The ‘Z’ at the end of the string indicates that the time is in UTC. By calling the toString()
method on this Date object, it automatically converts the UTC time to the local time based on the user’s time zone. This method is straightforward and effective for most use cases.
Method 2: Using toLocaleString()
Another powerful method for converting UTC to local time is using the toLocaleString()
function. This method provides more control over the formatting of the output, allowing you to specify options such as time zone and locale.
Here’s how it works:
const utcDate = new Date('2025-03-12T12:00:00Z');
const options = { timeZone: 'America/New_York', hour: '2-digit', minute: '2-digit', second: '2-digit' };
const localDate = utcDate.toLocaleString('en-US', options);
console.log(localDate);
Output:
08:00:00 AM
In this example, we again start with a UTC date. We then define an options object that specifies the time zone and the desired output format. By calling toLocaleString()
with these options, we can easily convert the UTC time to a formatted string representing the local time in New York. This method is particularly useful when you need to display the time in a specific format or locale.
Method 3: Using Moment.js
For more complex date and time manipulations, many developers turn to libraries like Moment.js. This library simplifies date handling in JavaScript, including conversions between UTC and local time.
Here’s an example of how to use Moment.js:
const moment = require('moment-timezone');
const utcDate = '2025-03-12T12:00:00Z';
const localDate = moment.utc(utcDate).tz('America/New_York').format('YYYY-MM-DD HH:mm:ss');
console.log(localDate);
Output:
2025-03-12 08:00:00
In this snippet, we first import the Moment.js library and then define a UTC date string. Using moment.utc()
, we create a moment object in UTC, and then we convert it to the desired time zone using the tz()
method. Finally, we format the output to a more readable string. Moment.js is a robust solution for applications that require extensive date and time manipulation.
Method 4: Using Luxon
Luxon is another modern library for handling dates and times in JavaScript. It offers a clean API and is built on the native DateTime object, making it a great alternative to Moment.js.
Here’s how you can convert UTC to local time using Luxon:
const { DateTime } = require('luxon');
const utcDate = '2025-03-12T12:00:00Z';
const localDate = DateTime.fromISO(utcDate, { zone: 'utc' }).setZone('America/New_York').toString();
console.log(localDate);
Output:
2025-03-12T08:00:00.000-04:00
In this example, we import the DateTime module from Luxon. We then parse the UTC date string using fromISO()
and specify the UTC zone. By calling setZone()
, we convert it to the desired local time zone. Finally, we convert the DateTime object to a string. Luxon provides an elegant and efficient way to handle time conversions.
Conclusion
Converting UTC to local time in JavaScript is essential for creating user-friendly applications that operate across various time zones. Whether you choose the built-in Date object, the toLocaleString()
method, or opt for powerful libraries like Moment.js or Luxon, you have plenty of options to achieve accurate time conversions. By implementing these methods, you can ensure that your users receive the correct time information, enhancing their overall experience with your application.
FAQ
-
What is UTC?
UTC stands for Coordinated Universal Time, which is the primary time standard by which the world regulates clocks and time. -
Why do I need to convert UTC to local time?
Converting UTC to local time ensures that users see the correct time based on their geographical location, which is crucial for scheduling and time-sensitive applications. -
Can I convert UTC to local time without using libraries?
Yes, you can use the built-in Date object in JavaScript to convert UTC to local time without any external libraries. -
What is the difference between Moment.js and Luxon?
Moment.js is an older library with a comprehensive feature set for date manipulation, while Luxon is a more modern library that utilizes the native DateTime object for better performance and simplicity. -
How do I handle daylight saving time in JavaScript?
Both Moment.js and Luxon automatically account for daylight saving time based on the specified time zone when converting dates.