How to Compare Two Arrays in JavaScript
-
Array.prototype.equals
to Compare Two Arrays in JavaScript -
JSON.stringify()
to Compare Arrays in JavaScript - Compare Two Arrays in JavaScript Using Loops
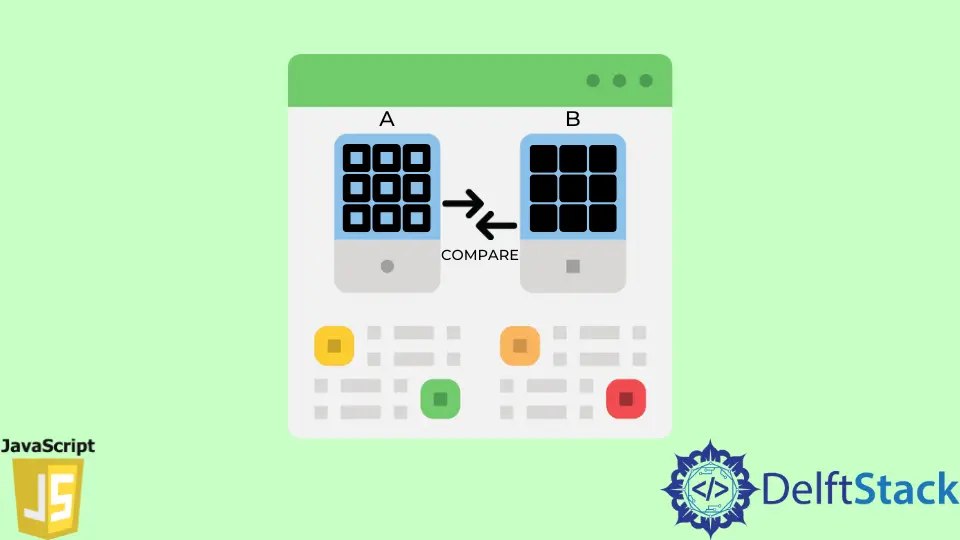
This tutorial introduces three ways to compare arrays in JavaScript. There are multiple ways of doing this, but the performance may vary.
Array.prototype.equals
to Compare Two Arrays in JavaScript
JavaScript provides us the capability to add new properties and methods to the existing classes. We can use Array.prototype
to add our custom method equals
into the Array object.
In the below example, we will first check the length of both the arrays and then comparing each item. We are also checking if a1
and a2
are Array
instances because if they are not, they are not identical. At last, we use the method to compare the first array with the second one by a1.equals(a2)
.
Example:
var a1 = [1, 2, 3];
var a2 = [1, 2, 3];
var a3 = [1, 2, 3, 4];
Array.prototype.equals = function(getArray) {
if (this.length != getArray.length) return false;
for (var i = 0; i < getArray.length; i++) {
if (this[i] instanceof Array && getArray[i] instanceof Array) {
if (!this[i].equals(getArray[i])) return false;
} else if (this[i] != getArray[i]) {
return false;
}
}
return true;
};
console.log('Comparing a1 and a2', a1.equals(a2));
console.log('Comparing a1 and a3', a1.equals(a3));
Output:
Comparing a1 and a2 true
Comparing a1 and a3 false
JSON.stringify()
to Compare Arrays in JavaScript
Another technique to compare two arrays is to first cast them to the string type and then compare them. JSON
is used to transfer data from/to a web server, but we can use its method here. We can do this is using JSON.stringify()
that converts an Array
to a string
. As now both the a1
and a2
are strings, we can use ===
to see if they are equal or not.
Example:
var a1 = [1, 2, 3];
var a2 = [1, 2, 3];
var a3 = [1, 2, 3, 4];
console.log('Comparing a1 and a2', JSON.stringify(a1) === JSON.stringify(a2));
console.log('Comparing a1 and a3', JSON.stringify(a1) === JSON.stringify(a3));
Output:
Comparing a1 and a2 true
Comparing a1 and a3 false
Compare Two Arrays in JavaScript Using Loops
Looping is the most traditional way of comparing arrays in JavaScript because it involves looping through the arrays and then comparing every single element with each other to check if they match.
To make it cleaner, we can use functions and then return boolean
as a result.
Example:
var a1 = [1, 2, 3];
var a2 = [1, 2, 3];
var a3 = [1, 2, 3, 4];
const getResult = function(a1, a2) {
var i = a1.length;
if (i != a2.length) return false;
while (i--) {
if (a1[i] !== a2[i]) return false;
}
return true;
};
console.log('Comparing a1 and a2', getResult(a1, a2));
console.log('Comparing a1 and a3', getResult(a1, a3));
Output:
Comparing a1 and a2 true
Comparing a1 and a3 false
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedInRelated Article - JavaScript Array
- How to Check if Array Contains Value in JavaScript
- How to Create Array of Specific Length in JavaScript
- How to Convert Array to String in JavaScript
- How to Remove First Element From an Array in JavaScript
- How to Search Objects From an Array in JavaScript
- How to Convert Arguments to an Array in JavaScript