How to Check Whether a String Matches a Regex in JavaScript
-
Use the
test()
Method for String Match With Regex -
Use the
match()
Method for String Match With Regex -
Use the
exec()
Method for String Match With Regex -
Use the
matchAll()
Method for String Match With Regex
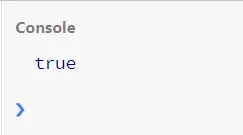
JavaScript has a different way of notating the Regex modifiers, quantifiers, and meta-characters. But these often denote the same meaning as any other regular expression convention.
The regex matches are counted when matching a string or even searching a string or character in a sentence. Technically, we extract the substring from a given string compared with a regular expression.
Here we will discuss three ways of retrieving matches through a generated regex. And in that case we will use the test()
, match()
, exec()
, and the matchAll()
method.
Use the test()
Method for String Match With Regex
The first thing to know about the test()
method is the format infers the matching condition. This specific method only prefers the boolean
format to answer.
It is convenient in conditional cases to further operate the functions. So, the general form is regular_expression.test(string)
.
Let’s jump to the code.
Code Snippet:
var string = 'abc123 is a ridiculous name';
var regularExpression = /\w\d+/;
var found = regularExpression.test(string);
console.log(found);
Output:
As you can see, the regular expression here depicts finding a single word followed by at least one or more digits. And according to our string, it has a matching, and thus the result turns out to be true
.
Use the match()
Method for String Match With Regex
Matching the exact string with a regex requires showing the match. When we depend on the match()
method, it returns the correct match of the whole string.
It will utilize each expression from the regex, and the final output will be the matched string.
Code Snippet:
var string = 'abc123 is a ridiculous name';
var regex = /i\w/g;
var found = string.match(regex);
console.log(found);
Output:
In this case, we have the format string.match(regex)
, and here the regex asks to find subsets that have i
followed by any other character word. So it has succeeded in doing so, but in the next section, we will also see how match()
differs from the exec()
method.
Use the exec()
Method for String Match With Regex
In the previous example of the match()
method, we visualized how the regex /i\w/g
extracts all possible matches. But here, for the exec()
method, the answer will only have one initial match of the string.
This is what the exec()
method does, and this can be handy and often work as a constraint. Let’s see the code for better understanding.
Code Snippet:
var string = 'abc123 is a ridiculous name';
var regex = /i\w/g;
var found = regex.exec(string);
console.log(found);
Output:
Use the matchAll()
Method for String Match With Regex
The exec()
method could not define all the possible matches from the string. So, the updates included the matchAll()
method to return all subsets that match the regex in an array format.
Code Snippet:
var string = 'abc123 is a ridiculous name';
var regex = /i\w/g;
var found = string.matchAll(regex);
for (var match of found) {
console.log(match);
}
Output:
The core difference between the exec()
and matchAll()
method is the exec()
method returns only the first match, and when followed by a while
loop, it can iterate through all the possible matches.
But in the case of the matchAll()
method, you cannot access the matches without initiating a loop system. Though it indeed results in possible matches.
This convention complies with the for...of
looping as well. Overall, the match()
method satisfy all kind of query finding the string matches.