How to Check if Sring Is a Number in JavaScript
-
Use the
isNaN()
Function to Check Whether a Given String Is a Number or Not in JavaScript -
Use the
+
Operator to Check Whether a Given String Is a Number or Not in JavaScript -
Use the
parseInt()
Function to Check Whether a Given String Is a Number or Not in JavaScript -
Use the
Number()
Function to Check Whether a Given String Is a Number or Not in JavaScript - Use the Regular Expressions to Check Whether a Given String Is a Number or Not in JavaScript
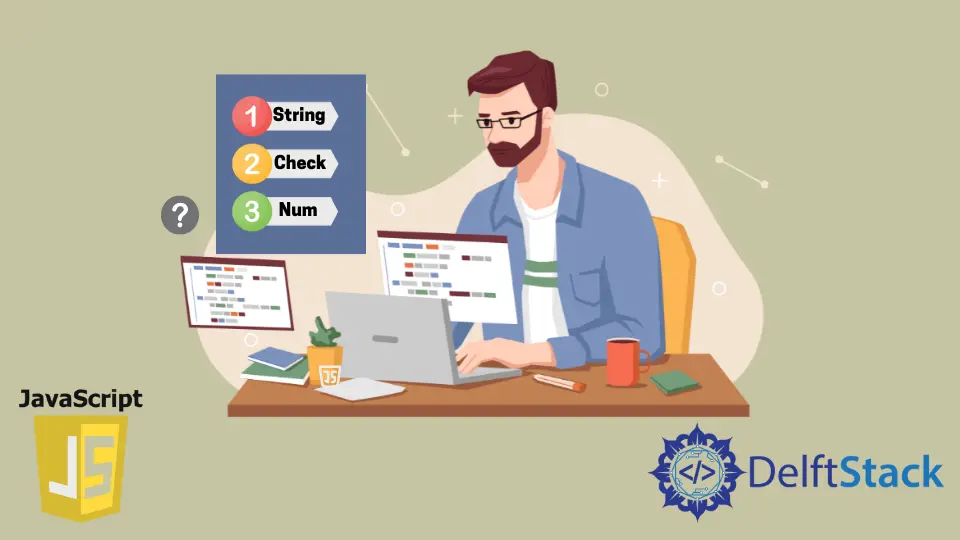
Number in the programming language is the data type that denotes integers, floats, etc. Strings represent all characters rather than just numeric values. Strings can contain numerical values.
We will check whether a given string is a number or not in this article.
Use the isNaN()
Function to Check Whether a Given String Is a Number or Not in JavaScript
The isNaN()
function stands for is Not-a-Number
. It is a built-in JavaScript function used to determine if a given value is not a numeric value, which includes both actual numbers and numeric strings.
The isNaN()
function returns true
if the value is not a number and false
if it is a number or can be converted into a valid number.
Here’s the basic syntax of the isNaN()
function:
isNaN(value)
value
: The value you want to check if it is a number. This can be a variable, constant, or an expression.
Example:
console.log(isNaN('195'))
console.log(isNaN('boo'))
console.log(isNaN('100px'))
Output:
false
true
true
If we want to create a function that returns true
for a valid value, we can always do that by creating a function that negates the output of the isNaN()
function.
function isNum(val) {
return !isNaN(val)
}
console.log(isNum('aaa'));
console.log(isNum('13579'));
console.log(isNum('-13'));
Output:
false
true
true
Now this function named isNum()
will return true
for a valid numeric value.
Handling Numeric Strings with Decimals
The isNaN()
function is versatile and can handle numeric strings with decimal points as well. For instance:
const str = '3.14'; // The string we want to check
const isNumeric = isNaN(str);
if (isNumeric) {
console.log(`${str} is NOT a number.`);
} else {
console.log(`${str} is a number.`);
}
The output will correctly identify '3.14'
as a number:
3.14 is a number.
Use the +
Operator to Check Whether a Given String Is a Number or Not in JavaScript
The +
operator in JavaScript serves a dual purpose: it can be used for arithmetic addition and as a unary operator for type coercion.
When applied to strings, the +
operator attempts to convert them into numeric values. If the string can be successfully converted into a number, the result will be a valid numeric value; otherwise, it will result in NaN
(Not-a-Number
).
For example,
console.log(+'195')
console.log(+'boo')
Output:
195
NaN
Use the parseInt()
Function to Check Whether a Given String Is a Number or Not in JavaScript
The parseInt()
method in JavaScript is used to parse a string and convert it into an integer (a whole number). It reads the string from left to right until it encounters a character that is not a valid part of an integer, and then it stops parsing.
Here’s the basic syntax of using parseInt()
:
parseInt(string[, radix]);
string
: The string to be parsed and converted into an integer.radix
(optional): An integer between 2 and 36 that represents the base of the numeral system used in the string. This parameter is optional, and if omitted, JavaScript assumes base 10 (decimal).
It returns NaN when it is unable to extract numbers from the string.
For example,
console.log(parseInt('195'))
console.log(parseInt('boo'))
Output:
195
NaN
Handling Numeric Strings with Radix
The parseInt()
method also allows you to specify the base (radix) of the numeral system used in the string. For example, you can parse binary (base 2), octal (base 8), or hexadecimal (base 16) numbers.
Here’s an example of parsing a hexadecimal string:
const hexString = '1A'; // Hexadecimal string
const intValue = parseInt(hexString, 16); // Specify base 16
if (isNaN(intValue)) {
console.log(`${hexString} is NOT a number.`);
} else {
console.log(`${hexString} is a number.`);
}
In this case, the output will correctly identify '1A'
as a hexadecimal number:
1A is a number.
Use the Number()
Function to Check Whether a Given String Is a Number or Not in JavaScript
The Number()
function converts the argument to a number representing the object’s value. If it fails to convert the value to a number, it returns NaN.
We can use it with strings also to check whether a given string is a number or not.
For example,
console.log(Number('195'))
console.log(Number('boo'))
Output:
195
NaN
Use the Regular Expressions to Check Whether a Given String Is a Number or Not in JavaScript
A regular expression, often referred to as a regex, is a pattern that specifies a set of strings. It can be used for pattern matching within strings, making it a versatile tool for tasks like string validation.
In JavaScript, you can create regular expressions using either the RegExp
constructor or by using the regex literal notation between slashes, like /pattern/
.
We can use such patterns to check whether a string contains a number or not.
First, we need to define a regular expression pattern that matches numeric values. We can create a simple pattern that matches positive and negative integers, as well as floating-point numbers:
const numberPattern = /^[-+]?[0-9]*\.?[0-9]+([eE][-+]?[0-9]+)?$/;
Here’s what this pattern does:
^
: Asserts the start of the string.[-+]?
: Matches an optional positive or negative sign.[0-9]*
: Matches zero or more digits before the decimal point.\.?
: Matches an optional decimal point.[0-9]+
: Matches one or more digits after the decimal point.([eE][-+]?[0-9]+)?
: Matches an optional exponent part, likee-3
orE+4
.
Now, apply the test()
method of the regular expression pattern to the string. The test()
method returns true
if the string matches the pattern and false
otherwise:
const isNumber = numberPattern.test(str);
For example,
function isNumeric(val) {
return /^-?\d+$/.test(val);
}
console.log(isNumeric('aaa'));
console.log(isNumeric('13579'));
console.log(isNumeric('-13'));
Output:
false
true
true