How to Check if Object Is Empty in JavaScript
-
Use
hasOwnProperty()
to Check if an Object Is Empty in JavaScript -
Use
Object.key()
to Check if an Object Is Empty in JavaScript -
Use the
Underscore.js
Library to Check if an Object Is Empty in JavaScript
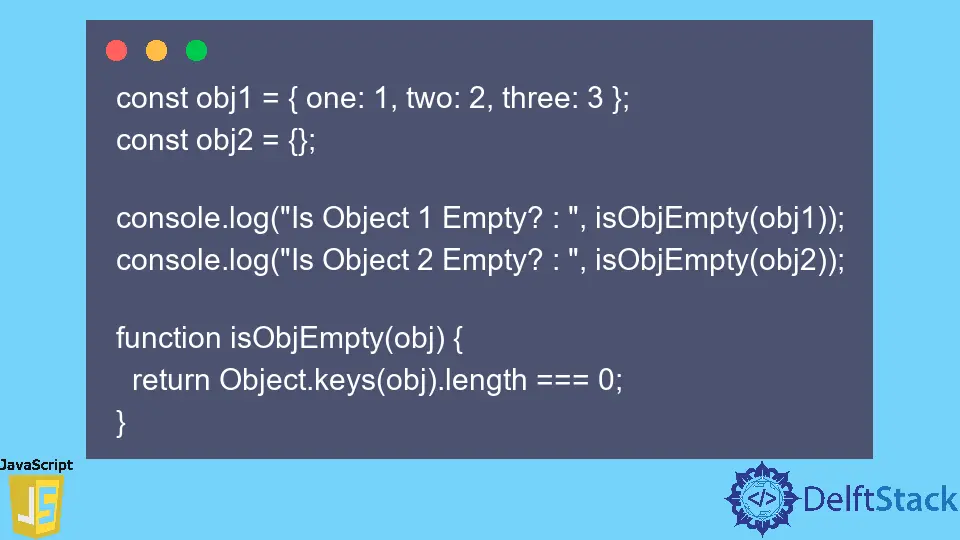
Objects play a significant role in JavaScript as they allow us to structure, maintain, and transfer data; however, there are times when the objects we get are empty.
In this article, we’ll demonstrate a few methods of how you can check if an object is empty in JavaScript.
Use hasOwnProperty()
to Check if an Object Is Empty in JavaScript
We can check if the specified property is present in the object or not. In the example below, we are checking two objects for the presence of the prop
property. The isObjEmpty()
function returns the boolean
command if the object is empty.
This function returns False
if the specified property is present; otherwise, it returns True
. Take note that this method is only useful if there’s no support for ECMAScript 5; check the following process if ECMAScript 5 is available:
const obj1 = {
one: 1,
two: 2,
three: 3
};
const obj2 = {};
console.log('Is Object 1 Empty? : ', isObjEmpty(obj1));
console.log('Is Object 2 Empty? : ', isObjEmpty(obj2));
function isObjEmpty(obj) {
for (var prop in obj) {
if (obj.hasOwnProperty(prop)) return false;
}
return true;
}
Output:
Is Object 1 Empty? : false
Is Object 2 Empty? : true
Use Object.key()
to Check if an Object Is Empty in JavaScript
In the previous example, we saw how we could check if the object is empty in JavaScript; however, different results occur if we have the support for ECMAScript 5.
In this example, we’re going to using the Object.keys()
command to check if the object has any keys. If the length of the keys is zero, then it’s empty; otherwise, it’s not empty.
const obj1 = {
one: 1,
two: 2,
three: 3
};
const obj2 = {};
console.log('Is Object 1 Empty? : ', isObjEmpty(obj1));
console.log('Is Object 2 Empty? : ', isObjEmpty(obj2));
function isObjEmpty(obj) {
return Object.keys(obj).length === 0;
}
Output:
Is Object 1 Empty? : false
Is Object 2 Empty? : true
Use the Underscore.js
Library to Check if an Object Is Empty in JavaScript
Another easy way to check if there are no data in an object is by using the Undescore.js
library; it’s a JavaScript library that has several helpful methods. One of the methods is _.isEmpty()
, which returns true if the object is empty.
We can include the library by specifying its URL in the <script>
tag. Additionally, we can use an underscore to access its functions as its name suggests.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Empty Object Example</title>
<script src="https://cdn.jsdelivr.net/npm/underscore@1.11.0/underscore-min.js"></script>
</head>
<body>
<script>
const obj1 = { one: 1, two: 2, three: 3 };
const obj2 = {};
console.log("Is Obj1 Empty? : ", _.isEmpty(obj1));
console.log("Is Obj2 Empty? : ", _.isEmpty(obj2));
</script>
</body>
</html>
Output:
Is Obj1 Empty? : false
Is Obj2 Empty? : true
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn