How to Check if All Values in Array Are True in JavaScript
- Using the Array.prototype.every() Method
- Using the Array.prototype.reduce() Method
-
Using a
for
Loop - Using the Array.prototype.filter() Method
- Conclusion
- FAQ
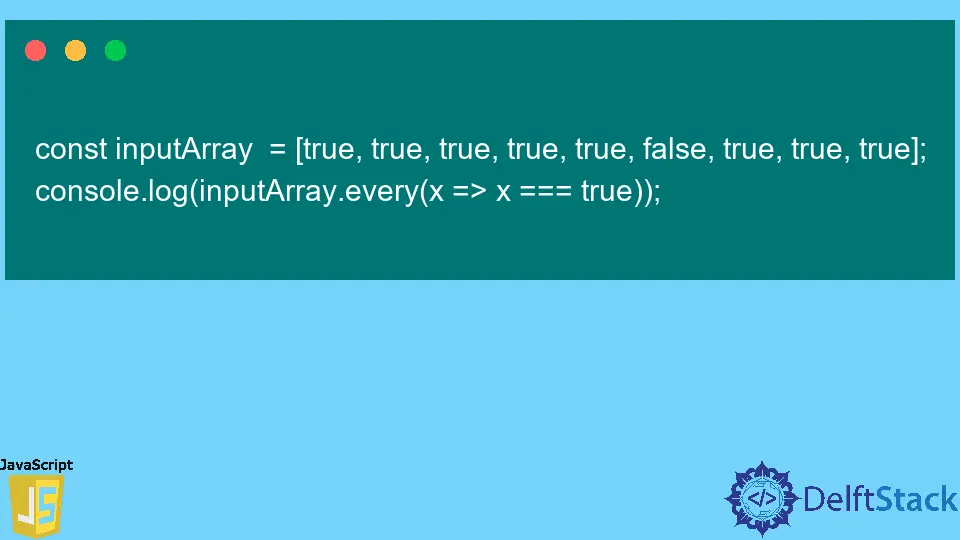
In today’s post, we’ll learn to check if all values in an array are true or not in JavaScript. This is a common task that developers encounter, especially when validating data. Whether you’re working with boolean values or need to evaluate truthy values, JavaScript provides several methods to achieve this efficiently. Understanding how to check array values can enhance your coding skills and improve your ability to write clean, effective scripts. So, let’s dive into the various methods available in JavaScript and explore how you can implement them in your projects.
Using the Array.prototype.every() Method
One of the simplest and most efficient ways to check if all values in an array are true is by using the every()
method. This method tests whether all elements in the array pass the test implemented by the provided function. If the array is empty, it returns true
.
Here’s a straightforward example:
const array = [true, true, true];
const allTrue = array.every(value => value === true);
console.log(allTrue);
Output:
true
In this example, we create an array containing three boolean values, all set to true
. The every()
method takes a callback function that checks if each value is true
. The result is logged to the console. If every value in the array meets the condition, allTrue
will be true
. Otherwise, it will return false
. This method is particularly useful for validating data and ensuring that all required conditions are met.
Using the Array.prototype.reduce() Method
Another effective way to determine if all values in an array are true is by leveraging the reduce()
method. This method executes a reducer function on each element of the array, resulting in a single output value.
Here’s how you can use it:
const array = [true, false, true];
const allTrue = array.reduce((accumulator, currentValue) => accumulator && currentValue, true);
console.log(allTrue);
Output:
false
In this code snippet, we apply the reduce()
method to the array. The reducer function takes two parameters: accumulator
and currentValue
. It combines them using the logical AND operator (&&
). We initialize the accumulator with true
, so if any value is false
, the entire expression will evaluate to false
. This method is versatile and can be adapted for more complex conditions, making it a powerful tool in your JavaScript toolkit.
Using a for
Loop
If you prefer a more traditional approach, you can use a simple for
loop to check if all values in an array are true. This method might be less concise than the previous ones, but it offers clear control over the iteration process.
Here’s how you can implement it:
const array = [true, true, true];
let allTrue = true;
for (let i = 0; i < array.length; i++) {
if (!array[i]) {
allTrue = false;
break;
}
}
console.log(allTrue);
Output:
true
In this example, we initialize a variable allTrue
to true
. We then iterate through the array using a for
loop. If we encounter a value that is falsy, we set allTrue
to false
and exit the loop using the break
statement. This approach is straightforward and allows for easy debugging, as you can inspect each iteration if necessary. While it may not be as elegant as the other methods, it remains an effective way to achieve the desired result.
Using the Array.prototype.filter() Method
Another method to check if all values in an array are true is by using the filter()
method. This method creates a new array with all elements that pass the test implemented by the provided function. If the length of the filtered array is equal to the original array, then all values are true.
Here’s an example:
const array = [true, true, false];
const allTrue = array.filter(value => !value).length === 0;
console.log(allTrue);
Output:
false
In this case, we use filter()
to create a new array that includes all falsy values. By checking if the length of this new array is zero, we can determine if all values in the original array are true. This method is quite readable and can be easily understood, making it a great choice for those who prefer clarity in their code.
Conclusion
Checking if all values in an array are true in JavaScript is a fundamental task that can be accomplished using various methods. From the concise every()
method to the more traditional for
loop, each approach has its advantages and can be selected based on your specific needs. Understanding these techniques not only enhances your coding skills but also allows you to write cleaner and more efficient JavaScript code. Experiment with these methods in your projects to see which one fits best for your use case.
FAQ
-
How can I check if all elements in an array are true in JavaScript?
You can use theevery()
method, which returns true if all elements pass the specified test. -
What is the difference between
every()
andreduce()
?
Theevery()
method checks if all elements meet a condition, whilereduce()
combines elements into a single value based on a specified operation. -
Can I use a
for
loop to check array values?
Yes, afor
loop is a straightforward way to iterate through an array and check each value. -
Is there a method that filters out false values?
Yes, you can use thefilter()
method to create a new array of falsy values and check its length. -
What happens if the array is empty?
Theevery()
method returns true for an empty array, whilereduce()
may throw an error unless initialized properly.
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedInRelated Article - JavaScript Array
- How to Check if Array Contains Value in JavaScript
- How to Create Array of Specific Length in JavaScript
- How to Convert Array to String in JavaScript
- How to Remove First Element From an Array in JavaScript
- How to Search Objects From an Array in JavaScript
- How to Convert Arguments to an Array in JavaScript