How to Implement Arraylist in JavaScript
- Creating Array Elements in JavaScript
- Accessing Array Elements in JavaScript
- Adding Elements to an Array in JavaScript
- Removing Elements from Array
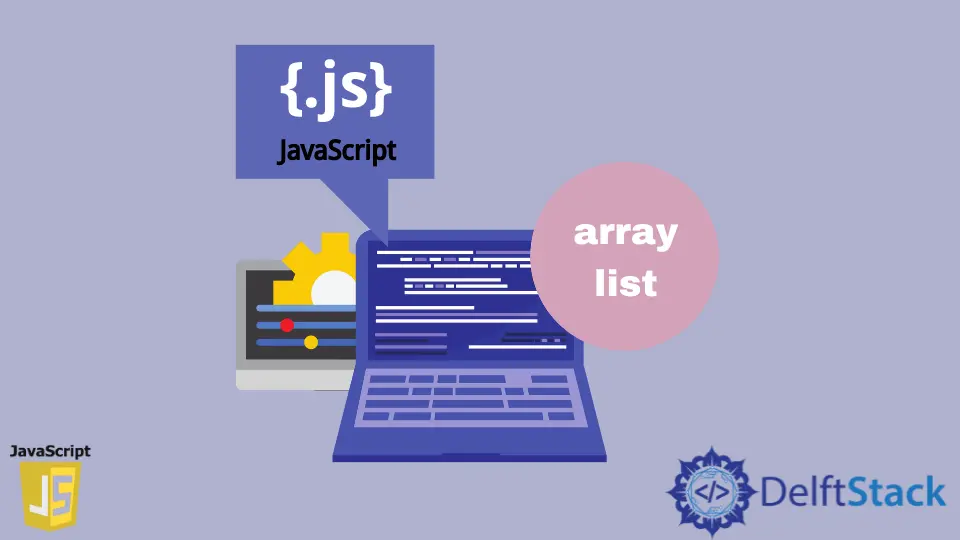
We are very familiar with the Arraylist
in Java that allows us to add elements to an array without specifying its size. It is a nice feature but do we have anything similar in JavaScript? Each language has its own set of data structures. In JavaScript, we have arrays that can store homogenous values as well as heterogeneous values. Heterogenous means the values may be of various data types like string, number, boolean, etc., all put together in a single array. It is a unique and better feature as compared to the ArrayList
in Java.
Creating Array Elements in JavaScript
Creating an array
in JavaScript is easy. We create an array using the var
keyword. It is similar to the way we create an Array using ArrayList
in Java. Java insists on specifying the data type of the ArrayList
. But in JavaScript, we do not explicitly declare the data type of the Array. We let the JavaScript interpreter make that decision based on the values assigned in the Array. Whatever be the case, for JavaScript, the type of the Array is object
. Refer to the following code to create an Array.
var a = [1, 6, 3, 5];
console.log(a.length);
console.log(typeof a);
console.log(typeof a[2]);
OOutput:
4
object
number
length
: JavaScript has thelength
attribute that returns the size of an array.typeof
:typeof
is a unary operator. And it is used to find the type of operand in JavaScript. The above code returns the data type of Array as anobject
with thetypeof
operator.
Accessing Array Elements in JavaScript
Just like the Arraylist
in Java, we can iterate over a JavaScript Array using loops, the for
loop and the while
loop. JavaScript also has the .foreach()
function for iterating over elements in an Array. We can execute certain lines of code on each Array element with foreach()
. The foreach
function accepts a function as a parameter. We can write this function as an inline function. Check the code snippet below to understand better.
var a = ['hello', 1, false];
a.forEach((i) => {
console.log(typeof i);
})
OOutput:
string
number
boolean
Here, we apply the typeof
operation on each element of the Array. The code further logs the output to the web browser console. JavaScript has another function, the .reverse()
function, that reverses the position of elements in the Array. Refer to the following code.
var a = ['hello', 1, false];
console.log(a.reverse());
OOutput:
[false, 1, "hello"]
The above log is as obtained in the Google Chrome web browser console.
Adding Elements to an Array in JavaScript
We can add elements to an ArrayList
with the .add()
function in Java. Similarly, in JavaScript, we have a few functions that we can use to add elements at various positions in an array.
.push()
: As the name suggests, we can add elements into an array with thepush()
function. It appends the element, passed as a parameter to the function, at the end of the Array. Thepush()
function alters the original Array. Hence, be aware of it while using it in code. It returns the modified size of the Array after adding the new element..unshift()
: If we have to add an element at the beginning of the Array, it will be a hectic task as we will need to reposition all the contents in the Array by an index. We can achieve this task with the.unshift()
function in JavaScript. Usingunshift()
, we can add one or more elements to the beginning of the Array. We pass the elements we wish to add to the Array as parameters to theunshift
function.
var a = ['hello', 1, false];
a.push(2.15);
console.log(a);
a.unshift(123);
console.log(a);
OOutput:
["hello", 1, false, 2.15]
[123, "hello", 1, false, 2.15]
The output is as obtained in the google chrome web browser. Note that the operations alter the original Array without having to use the assignment operator.
Removing Elements from Array
Likewise, we can remove an element from an array using the following JavaScript functions. It is comparable to the remove()
function of ArrayList
in Java.
.pop()
If we are to remove an element from behind thearray
we can use the.pop()
function. Just be aware that the function alters the original Array and returns the last element just popped by it..shift()
If we have to remove an element from the beginning of the Array, we can use the.shift()
function. Like.pop()
,.shift()
also alters the original array. So be aware while using the.shift()
function.
var a = [123, 'hello', 1, false, 2.15];
a.pop();
console.log(a);
a.shift();
console.log(a);
Output:
[123, "hello", 1, false]
["hello", 1, false]
There are a couple of things to note here.
- Both the
pop()
and theshift()
functions do not accept any parameters. And they are used to remove one element from an array at a given time. - They alter the original Array without the need for an assignment operator. Hence, we must use them judiciously else we may fall trapped to unknown side effects that will be hard to debug.
Add and Remove Multiple Elements from an Array
There is another method in JavaScript, the splice()
function. We can use it to add or remove one or more elements from an array. The splice()
function takes a few parameters as follows.
startIndex
: We specify the array index with thestartIndex
parameter.count
: This parameter specifies the number of elements we wish to delete from the Array. If no count is specified, then thesplice()
will delete all elements from thestartIndex
to the end of the Array.elements
: The last parameter(s) is the list of elements that we wish to add to the Array from thestartIndex
. If no element is specified in thesplice()
function, it will remove the element from the Array.
Let us look at the following code to understand more.
var a = [123, 'hello', 1, false, 2.15];
a.splice(2, 1, 'there');
console.log(a);
a.splice(2, 2);
console.log(a);
a.splice(2);
console.log(a);
Output:
[123, "hello", "there", false, 2.15]
[123, "hello", 2.15]
[123, "hello"]
Related Article - JavaScript Array
- How to Check if Array Contains Value in JavaScript
- How to Create Array of Specific Length in JavaScript
- How to Convert Array to String in JavaScript
- How to Remove First Element From an Array in JavaScript
- How to Search Objects From an Array in JavaScript
- How to Convert Arguments to an Array in JavaScript