Exception Types and Their Handling in Java
- Exception Types in Java and Their Handling
- Built-in Exceptions in Java
- User-defined Exceptions in Java
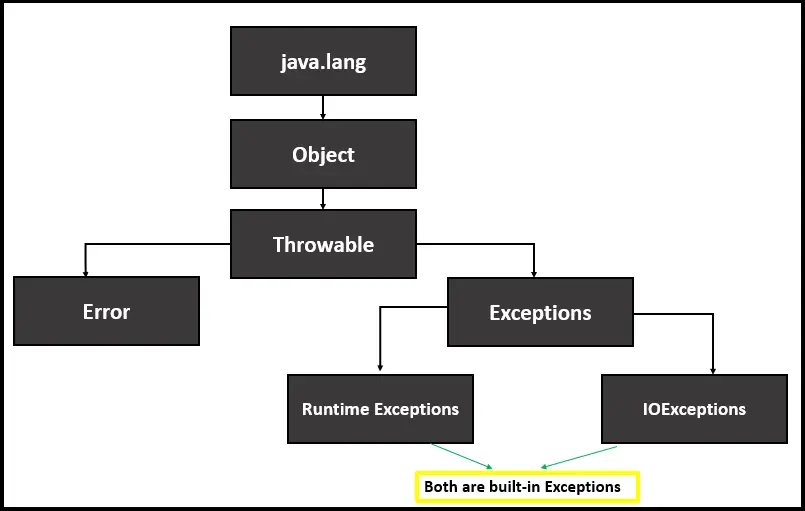
We will learn about the exception types in Java and their handling. We will see built-in and user-defined exceptions on the definition level and understand them by writing code examples.
Exception Types in Java and Their Handling
The exception is the unexpected event that occurs during a program’s execution that disturbs the program’s normal execution flow, which results in the program’s termination abnormally. We, as computer programmers, can handle these exceptions in our program.
The following is the hierarchy of the Java Exceptions.
Java has some built-in exceptions that relate to its different class libraries. Java also lets the users write their exceptions as per their project requirements.
The exceptions are classified in two ways that are listed below.
- Built-in Exceptions
1.1 Checked Exceptions
1.2 Unchecked Exceptions - User-defined Exceptions
These exceptions can be handled using a try-catch
block. Let’s understand each of them theoretically as well as practically.
Built-in Exceptions in Java
The exceptions already accessible in Java libraries are known as built-in exceptions. These are very useful to demonstrate specific error situations; for instance, FileNotFoundException
occurs when the program cannot find the expected file.
The built-in exceptions are further categorized into two categories, Checked Exceptions & Unchecked Exceptions. Let’s deep dive into each of them.
Checked Exceptions in Java
The checked exceptions are known as IOExceptions
. These are also called compile-time exceptions because a compiler can check these exceptions at compile-time.
The compilers ensure that the computer programmer has handled the exception to avoid the program termination.
It is necessary to handle these exceptions; otherwise, the program would not be compiled, and a compilation error would be generated. Some of the checked exceptions are listed below.
ClassNotFoundException
- occurs when we attempt to access the class which is not defined. Or we can say whose definition is not available.InterruptedException
- occurs when a thread is interrupted while waiting, sleeping, or processing.InstantiationException
- occurs when we try to create an object (instance) of the class but fail to instantiate.IOException
- this exception occurs whenever the IO (input-output) operations are interrupted or failed.FileNotFoundException
- occurs when the program can’t find the specified file.
The code examples are given below to practice.
Example Code (for ClassNotFoundException
):
public class Test {
public static void main(String args[]) {
try {
// the forName() looks for the class "ABC" whose definition
// is missing here
Class.forName("ABC");
} catch (ClassNotFoundException e) {
System.out.println("The ClassNotFoundException exception has been raised.");
}
}
}
Output:
The ClassNotFoundException exception has been raised.
Example Code (for InterruptedException
):
class practiceClass extends Thread {
public void run() {
try {
for (int i = 0; i < 5; i++) {
// current thread sleeps to give another
// thread an opportunity to execute
System.out.println("Child Thread is being executed.");
Thread.sleep(1000);
}
} catch (InterruptedException e) {
System.out.println("InterruptedException has been raised.");
}
}
}
public class Test {
public static void main(String[] args) throws InterruptedException {
// instance of praticeClass
practiceClass thread = new practiceClass();
// start thread
thread.start();
// interrupt thread
thread.interrupt();
System.out.println("The execution of the Main thread was accomplished.");
}
}
Output:
The execution of the Main thread was accomplished.
Child Thread is being executed.
InterruptedException has been raised.
Example Code (for InstantiationException
):
// we can't instantiate this class
// because it has a private constructor
class practiceClass {
private practiceClass() {}
}
public class Test {
public static void main(String args[]) {
try {
practiceClass c = new practiceClass();
} catch (InstantiationException e) {
e.printStackTrace();
}
}
}
Output:
/Test.java:11: error: practiceClass() has private access in practiceClass
practiceClass c = new practiceClass();
^
1 error
Example Code (for IOException
):
import java.io.*;
public class Test {
public static void main(String args[]) {
FileInputStream file = null;
try {
file = new FileInputStream("E:/Test/Hello.txt");
} catch (FileNotFoundException e) {
System.out.println("File Not Found!");
}
int i;
try {
while ((i = file.read()) != -1) {
System.out.print((char) i);
}
file.close();
} catch (IOException e) {
System.out.println("I/O Exception has occurred.");
}
}
}
Output:
File Not Found!
Exception in thread "main" java.lang.NullPointerException: Cannot invoke "java.io.FileInputStream.read()" because "<local1>" is null
at Test.main(Test.java:13)
Example Code (for FileNotFoundException
):
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileReader;
public class Test {
public static void main(String args[]) {
try {
// The specified file doesn't exist in the machine
File file = new File("E://test.txt");
FileReader fileReader = new FileReader(file);
} catch (FileNotFoundException e) {
System.out.println("The specified file does not exist.");
}
}
}
Output:
The specified file does not exist.
Unchecked Exceptions in Java
The unchecked exceptions are the opposite of the checked exceptions and are not detected at compile time even if we did not handle them properly. Normally, these kinds of exceptions occur when bad data is provided by the user while interacting with the program.
The unchecked exceptions, also known as runtime exceptions, occur due to an error in the program. Some of the unchecked exceptions are listed below.
ArithmeticException
- occurs when an unexpected condition is found in an arithmetic operation, for instance, dividing a number by zero.ClassCastException
- occurs when we try to cast a class inappropriately from one type to another.NullPointerException
- raises when the program refers to thenull
object’s members.ArrayIndexOutOfBoundsException
- occurs when we attempt to access an element at an invalid index.ArrayStoreException
- raises when we try to store the object’s incorrect type into the array of objects.
Let’s understand them with code examples which are given below.
Example Code (for ArithmeticException
):
public class Test {
public static void main(String args[]) {
try {
int num1 = 5, num2 = 0;
System.out.println("Answer = " + num1 / num2);
} catch (ArithmeticException e) {
System.out.println("Division by 0 is not allowed.");
}
}
}
Output:
Division by 0 is not allowed.
Example Code (for ClassCastException
):
public class Test {
public static void main(String[] args) {
try {
Object object = new Integer(1000);
System.out.println((String) object);
} catch (ClassCastException e) {
System.out.println("The Object can't be converted to String.");
}
}
}
Output:
The Object can't be converted to String.
Example Code (for NullPointerException
):
public class Test {
public static void main(String args[]) {
try {
String message = null;
System.out.println(message.charAt(0));
} catch (NullPointerException e) {
System.out.println("NullPointerException has been raised.");
}
}
}
Output:
NullPointerException has been raised.
Example Code (for ArrayIndexOutOfBoundsException
):
public class Test {
public static void main(String args[]) {
try {
int array[] = new int[5];
for (int i = 0; i < array.length; i++) array[i] = i;
System.out.println(array[6]);
} catch (ArrayIndexOutOfBoundsException e) {
System.out.println("ArrayIndexOutOfBoundsException has occurred.");
}
}
}
Output:
ArrayIndexOutOfBoundsException has occurred.
Example Code (for ArrayStoreException
):
public class Test {
public static void main(String args[]) {
try {
Number[] array = new Double[3];
array[0] = new Integer(5);
} catch (ArrayStoreException e) {
System.out.println("You're allowed to store Double Type numbers only.");
}
}
}
Output:
You're allowed to store Double Type numbers only.
User-defined Exceptions in Java
In some situations, the built-in exceptions do not serve as expected. For that, the users (computer programmers) have to define their exception by extending the Exception
class and considering the project requirement, and this exception is called a user-defined exception.
Let’s write a program that throws an exception whenever the marks would be less than 50.
Example Code (user-defined exception):
public class userDefinedException extends Exception {
// store students' roll numbers
private static int rollNumber[] = {101, 102, 103, 104};
// store students' names
private static String firstname[] = {"Sara", "John", "Jelly", "Daniel"};
// store students' obtained marks
private static double marks[] = {80.00, 70.00, 65.0, 49.00};
// write default constructor
userDefinedException() {}
// write parametrized constructor
userDefinedException(String str) {
super(str);
}
// write main method
public static void main(String[] args) {
try {
// write table's header
System.out.println("Roll#"
+ "\t"
+ "Student"
+ "\t"
+ "Marks");
// display the actual information using loop
for (int i = 0; i < marks.length; i++) {
System.out.println(rollNumber[i] + "\t\t" + firstname[i] + "\t" + marks[i]);
// display user-defined exception if marks < 50
if (marks[i] < 50) {
userDefinedException me = new userDefinedException("The marks are less than 50.");
throw me;
}
}
} catch (userDefinedException e) {
e.printStackTrace();
}
}
}
Output:
Roll# Student Marks
101 Sara 80.0
102 John 70.0
103 Jelly 65.0
104 Daniel 49.0
userDefinedException: The marks are less than 50.
at userDefinedException.main(userDefinedException.java:26)
This program raises the exception by saying The marks are less than 50.
if any of the student’s marks are less than 50.