How to Create Temporary Files in Java
- Create Temporary Files in Java Using Java Legacy IO
- Create Temporary Files in Java Using the Java NIO Library
- Hidden Exceptions to Handle When Creating Temporary Files in Java
- Conclusion
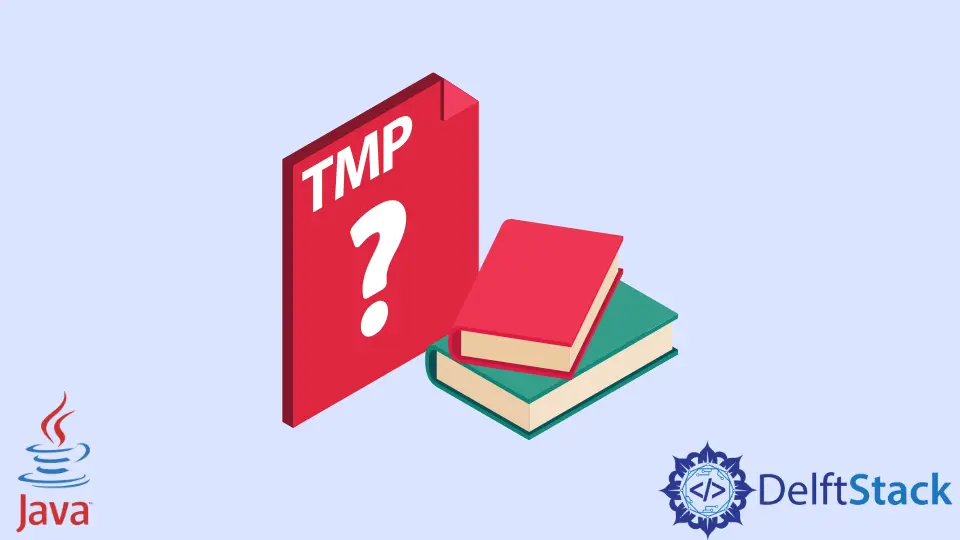
This article will demonstrate how to create temporary files in Java using different alternatives.
We use the temporary files in Java to store the temporary information during the execution of a program, to free up resources, or to communicate information to other parts of the program.
Create Temporary Files in Java Using Java Legacy IO
We can use the standard File library in the java.io
package to create temporary files in Java. We use one of the createTempFile()
methods to make the temporary file.
Let us understand both of the polymorphic forms one by one.
the First Polymorphic Form of Method to Create Temporary Files in Java
We can pass two arguments in the first implementation: prefix
and suffix
. The file name is generated by adding the prefix and the suffix to an identifier.
The JVM itself generates the identifier. The name of the temporary file is similar to the form given below.
prefixIDsuffix
The temporary file is stored in the default directory for temporary files when we create it using this method. The default directory for storing temporary files can differ depending on the operating system.
The \tmp
directory is the default directory for the temporary files in the Linux operating system. If we use the Windows platform, the default directory is generally C:\\WINNT\\TEMP
.
Let us see the definition of the first polymorphic form of the createTempFile()
method.
public static File createTempFile(String prefix, String suffix) throws IOException
As we can see, this method throws IOException. Therefore, we should use a try-catch
block while using this method.
If we do not want to use a try-catch block, our method should throw the same exception to handle it later, either by us or the JVM.
The method returns a File object that contains the absolute path of the created temporary file.
Code:
import java.io.File;
import java.io.IOException;
public class tempFile {
public static void main(String[] args) {
try {
File file = File.createTempFile("myTemp", ".tmp");
System.out.print(file);
file.deleteOnExit();
} catch (IOException e) {
e.printStackTrace();
}
}
}
Output:
/tmp/myTemp14969130618851885659.tmp
If we do not provide any suffix argument, the JVM adds the default .tmp
suffix. However, we can not omit the prefix argument, and it should be at least three characters in length.
We have used the deleteOnExit()
method that deletes the file when the JVM exits. We should always delete the temporary file when its use is over as a good practice.
the Second Polymorphic Form of Method to Create Temporary Files in Java
We can provide three arguments in the second polymorphic implementation of the createTempFile()
method. The first two arguments are similar, as we discussed above.
A third argument is a File object that we can use to provide the specific path for our temporary file. If we pass null
to the third argument, this implementation works the same way as the first.
Let us see the method definition of the second polymorphic form of the createTempFile()
method.
public static File createTempFile(String prefix, String suffix, File directory) throws IOException
The naming convention, exceptions, and all other workings of this form are the same as the previous form of the method.
Let us see the code to create a temporary file using this method.
import java.io.File;
import java.io.IOException;
public class tempFile {
public static void main(String[] args) {
try {
File path = new File("/home/stark/eclipse-workspace-java/JavaArticles");
File file = File.createTempFile("myTemp", ".tmp", path);
System.out.print(file);
file.deleteOnExit();
} catch (IOException e) {
e.printStackTrace();
}
}
}
Output:
/home/stark/eclipse-workspace-java/JavaArticles/myTemp10717312880412401466.tmp
We should note that the temporary file is created in the directory as provided in the code rather than the default directory.
Create Temporary Files in Java Using the Java NIO Library
We can use the alternative java.nio.file.Files
library to create the temporary files during run time. This library behaves similarly to the legacy Java IO library that we have discussed.
The NIO library also provides two polymorphic forms of the createTempFile()
method. The difference between the two polymorphic forms is that the other one lets us pass the file path.
The file’s name is created using prefix and suffix and an identifier similar to the Java legacy IO library. The default path of the temporary file is also the same as the Java legacy IO library, as discussed above.
The significant difference between the legacy Java IO method for creating temporary files and the NIO library is that we get more control over the security parameters of the file using the NIO library.
Let us see the method definition of both polymorphic forms of the createTempFile()
method.
public static Path createTempFile(String prefix, String suffix, FileAttribute<?>... attrs)
throws IOException
The above given polymorphic form is the first form. The second polymorphic form is given below.
public static Path createTempFile(Path dir, String prefix, String suffix, FileAttribute<?>... attrs)
throws IOException
We can find the FileAttribute
argument in both the polymorphic forms. We can use this argument to provide the list of file-level attributes such as permissions.
The methods return a Path object that represents the path of the temp file created by the method.
Let us see the code to create a temp file using this library.
import java.io.File;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.nio.file.attribute.FileAttribute;
import java.nio.file.attribute.PosixFilePermission;
import java.nio.file.attribute.PosixFilePermissions;
import java.util.Set;
public class tempFile2 {
public static void main(String[] args) {
try {
Path path = Paths.get("/home/stark/eclipse-workspace-java/JavaArticles");
Set<PosixFilePermission> filePerm = PosixFilePermissions.fromString("rwxrwxrwx");
FileAttribute<Set<PosixFilePermission>> fileAttr =
PosixFilePermissions.asFileAttribute(filePerm);
Path temp = Files.createTempFile(path, "myTemp", ".tmp", fileAttr);
System.out.print(temp);
File file = new File(path.toString());
file.deleteOnExit();
} catch (IOException e) {
}
}
}
The code uses all four parameters of the createTempFile()
method. We can omit the parameters according to our needs.
However, remember that the prefix parameter can not be omitted.
If we omit the Path
parameter, we will use the first polymorphic form of the method, and the temp file will be saved to the default directory for temp files.
The file will be created with default permissions if we omit the FileAttribute
parameter.
The code also uses the PosixFilePermissions library to define the file permissions using simple strings. We can read more about it here.
We should note that the NIO library does not have the deleteOnExit()
method of its own. Therefore, the code uses the legacy IO file library to delete temp files.
Output:
/home/stark/eclipse-workspace-java/JavaArticles/myTemp5731129001304860331.tmp
Hidden Exceptions to Handle When Creating Temporary Files in Java
Although we have already seen that Java legacy IO library and NIO library methods for creating temporary files throw IOException, there are also some hidden exceptions that we should keep in mind.
These exceptions are hidden because they are not directly raised using the method itself. These are instead thrown because of other executions inside the method.
SecurityException
: We can get this exception due to the operating system not letting the program create the temp file.IllegalArgumentException
: We will get this exception when we pass some weird set of arguments that Java Virtual Machine does not recognize.FileAlreadyExistsException
: If we specify theCREATE_NEW
file attribute option, and the file already exists, we get this exception.UnsupportedOperationException
: The method throws this exception when we specify a file attribute that can not be recognized.
Conclusion
In the article, we have seen two different libraries to create temp files in Java. The Java legacy IO library is widely used, but we can use the NIO library to have tighter control of the file permissions.
However, if we do not specify that the file is created with default permissions, it can have highly restricted permissions.