How to Check if String Contains a Case Insensitive Substring in Java
- Find Case Insensitive Substring in a String in Java
-
Find Case Insensitive Substring in a String Using
StringUtils
in Java -
Find Case Insensitive Substring in a String Using the
contains()
Method in Java -
Find Case Insensitive Substring in a String Using the
matches()
Method in Java
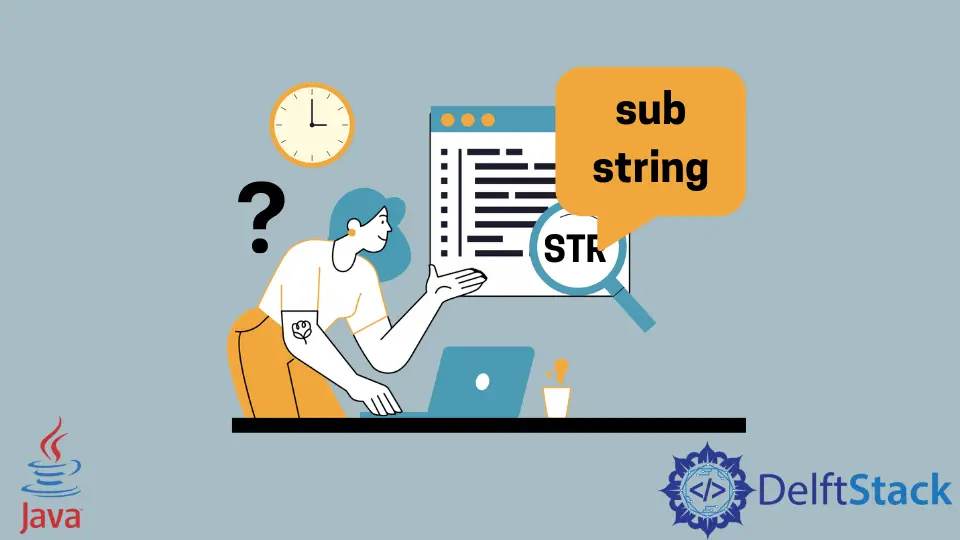
This tutorial introduces how to check or find if a string contains a substring in Java.
String
is a sequence of characters sometimes refers as an array of chars. In Java, String
is a class that handles all the string-related operations and provides utility methods to work on.
This article demonstrates how to find a substring in a string.
A substring is a part of a string that is also a string. It can have one or more chars.
A case-insensitive string is a string that does not bother about the lower or upper case of letters. Let’s understand with some examples.
Find Case Insensitive Substring in a String in Java
In this example, we used the Pattern
class and its compile()
, matcher()
, and find()
methods to check whether a string contains a substring or not. We used CASE_INSENSITIVE
, which returns a boolean value, either true
or false
.
See the example below.
import java.util.regex.Pattern;
public class SimpleTesting {
public static void main(String[] args) {
String str = "DelftStack";
String strToFind = "St";
System.out.println(str);
boolean ispresent =
Pattern.compile(Pattern.quote(strToFind), Pattern.CASE_INSENSITIVE).matcher(str).find();
if (ispresent)
System.out.println("String is present");
else
System.out.println("String not found");
}
}
Output:
DelftStack
String is present
Find Case Insensitive Substring in a String Using StringUtils
in Java
Working with the Apache commons library, you can use the StringUtils
class and its containsIgnoreCase()
method to find a substring. See the example below.
You must add Apache commons JARs to your project to execute this code.
import org.apache.commons.lang3.StringUtils;
public class SimpleTesting {
public static void main(String[] args) {
String str = "DelftStack";
String strToFind = "St";
System.out.println(str);
boolean ispresent = StringUtils.containsIgnoreCase(str, strToFind);
if (ispresent)
System.out.println("String is present");
else
System.out.println("String not found");
}
}
Output:
DelftStack
String is present
Find Case Insensitive Substring in a String Using the contains()
Method in Java
In this example, we used the contains()
method of the String class that returns true
if the substring is present. We used toLowerCase()
to convert all the chars in lowercase first then pass to the contains()
method.
See the example below.
public class SimpleTesting {
public static void main(String[] args) {
String str = "DelftStack";
String strToFind = "St";
System.out.println(str);
boolean ispresent = str.toLowerCase().contains(strToFind.toLowerCase());
if (ispresent)
System.out.println("String is present");
else
System.out.println("String not found");
}
}
Output:
DelftStack
String is present
Find Case Insensitive Substring in a String Using the matches()
Method in Java
In this example, we used the matches()
method of the String
class that returns true
if the substring is present. It takes regex as an argument.
See the example below.
public class SimpleTesting {
public static void main(String[] args) {
String str = "DelftStack";
String strToFind = "St";
System.out.println(str);
boolean ispresent = str.matches("(?i).*" + strToFind + ".*");
if (ispresent)
System.out.println("String is present");
else
System.out.println("String not found");
}
}
Output:
DelftStack
String is present