Stream Filter in Java
-
Stream With
filter()
andcollect()
Methods in Java -
Stream With
filter()
andforEach()
Method in Java -
Stream With
filter()
and Multiple Conditions in Java -
Stream With
filter()
andmap()
in Java -
Stream With Stacking Several
filter()
Methods in Java
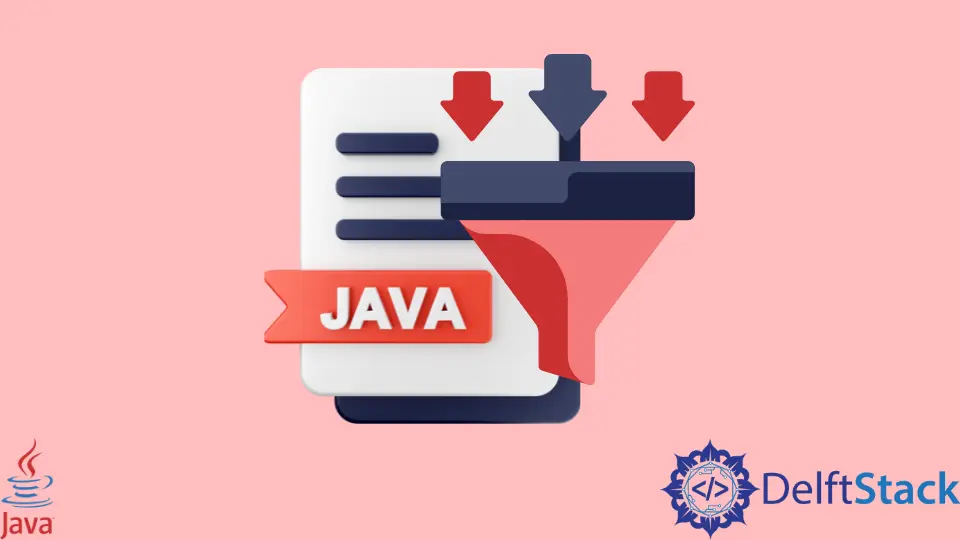
This tutorial introduces the stream API and its filter()
method in Java. In Java, a stream is a collection of objects.
A stream doesn’t store any data, and therefore it is not a data structure. A stream has various methods which can be pipelined together to get the desired result.
One of those methods is the filter
method. We will see various examples using this article’s filter()
operation.
Let us first understand the filter()
operation. The filter()
method returns a new stream with elements, which match the predicate passed into it as an argument.
In other words, the filter()
operation is used to filter an incoming stream and output a new stream. The syntax for this method is:
Stream<T> filter(Predicate<? super T> predicate)
A predicate is a functional interface which means we can pass a lambda function or method reference here. Let us now look at some examples.
Stream With filter()
and collect()
Methods in Java
The collect()
operation is a type of terminal operation.
Terminal operations are applied to a stream at the end to produce a result. After a terminal operator is applied, the stream is consumed and can no longer be consumed.
The collect()
operation returns the output of the intermediate operations; it can also be used to convert the output into the desired data structure. Look at the example below.
Suppose we have a list of all the students in a class, and we want to create a new list containing all the students’ names except for one. Suppose that one student has left the school.
We can do this by this given code.
import java.util.*;
import java.util.stream.Collectors;
public class MyClass {
public static void main(String args[]) {
List<String> students = Arrays.asList("Kashish", "Riyan", "Ritu");
List<String> new_students =
students.stream().filter(sName -> !"Riyan".equals(sName)).collect(Collectors.toList());
new_students.forEach(System.out::println);
}
}
Output:
Kashish
Ritu
In this code, first, a stream of student names is created.
The stream is then filtered using a predicate: all the names not equal to Riyan. The filter
operation generates a new stream with all the elements satisfying this predicate.
The newly created stream is then converted into a List
using the collect
operation.
Stream With filter()
and forEach()
Method in Java
The forEach()
method is also a terminal operation. It applies the function passed as an argument to each stream element.
It works like a foreach
loop but works for stream only. See the example below.
Suppose we want to print all the numbers divisible by five. The code below works like that.
import java.util.*;
import java.util.stream.Collectors;
public class MyClass {
public static void main(String args[]) {
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6, 7, 8, 9, 21, 11, 10, 25);
numbers.stream().filter(n -> n % 5 == 0).forEach(System.out::println);
}
}
Output:
5
10
25
Stream With filter()
and Multiple Conditions in Java
We can pass multiple conditions into the filter()
operation using logical operators to get more refined results. Suppose we want to find all the elements divisible by five and greater than ten; see the example below.
import java.util.*;
import java.util.stream.Collectors;
public class MyClass {
public static void main(String args[]) {
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6, 7, 8, 9, 21, 11, 10, 25);
numbers.stream().filter(n -> n % 5 == 0 && n > 10).forEach(System.out::println);
}
}
Output:
25
Stream With filter()
and map()
in Java
The map()
operation is just like the forEach()
operation.
It applies a function to each element of the stream. It is an intermediate operation rather than a terminal operation.
Suppose we have to square all the elements divisible by 5. We can do this by pipelining the filter
and map
operations.
See the example below.
import java.util.*;
import java.util.stream.Collectors;
public class MyClass {
public static void main(String args[]) {
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6, 7, 8, 9, 21, 11, 10, 25);
List<Integer> new_numbers =
numbers.stream().filter(n -> n % 5 == 0).map(x -> x * x).collect(Collectors.toList());
new_numbers.forEach(System.out::println);
}
}
Output:
25
100
625
In the above code, first, the list of integers is filtered. After filtering the list, each element remaining in the stream is squared using the map()
operation.
At last, we convert the stream into the list and store it in the new_numbers
list.
Stream With Stacking Several filter()
Methods in Java
We can stack the filter()
method by applying one after another to get a more refined result.
Suppose we want first to filter the strings whose length is greater than three and then filter the strings that contain stack
as a substring. We can do this by using the below code example.
import java.util.*;
import java.util.stream.Collectors;
public class MyClass {
public static void main(String args[]) {
List<String> str = Arrays.asList("Hello", "I", "Love", "Delftstack");
List<String> new_string = str.stream()
.filter(n -> n.length() > 3)
.filter(n -> n.contains("stack"))
.collect(Collectors.toList());
new_string.forEach(System.out::println);
}
}
Output:
Delftstack
Note that the above code is equivalent to using multiple conditions using the &&
operator. Look below:
import java.util.*;
import java.util.stream.Collectors;
public class MyClass {
public static void main(String args[]) {
List<String> str = Arrays.asList("Hello", "I", "Love", "Delftstack");
List<String> new_string = str.stream()
.filter(n -> n.length() > 3 && n.contains("stack"))
.collect(Collectors.toList());
new_string.forEach(System.out::println);
}
}
Output:
Delftstack
Related Article - Java Method
- Method Chaining in Java
- Optional ifPresent() in Java
- How to Override and Overload Static Methods in Java
- How to Use of the flush() Method in Java Streams
- How to Use the wait() and notify() Methods in Java
- How to Custom Helper Method in Java