How to Shuffle Array in Java
-
Use the
random()
Method to Shuffle an Array in Java -
Use the
shuffle()
Method to Shuffle an Array in Java -
Use the
Arrays.sort()
Method with A Custom Comparator to Shuffle an Array in Java -
Use the
List
andListIterator
to Shuffle an Array in Java -
Shuffling an Array in Java Using
ThreadLocalRandom
(Java 7+) - Conclusion
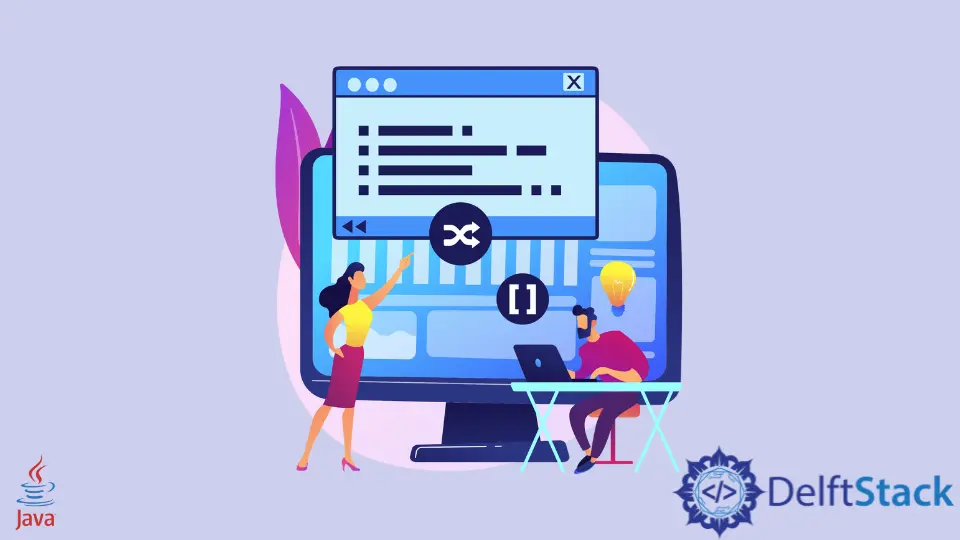
Arrays are a fundamental data structure in Java, providing a convenient way to store and manipulate collections of elements. Shuffling an array is essential in applications like card games, statistical simulations, and randomized algorithms. Java offers multiple techniques to achieve this.
In this article, we will explore various methods and techniques for shuffling arrays in Java, each with its advantages and use cases.
Use the random()
Method to Shuffle an Array in Java
We can use the Fisher-Yates shuffle array method to shuffle a given array randomly. This method aims to start from the last element of a given array and keep swapping it with a randomly selected element in the array.
We use the Random()
function from the random class to randomly pick the indexes of an array. We will be importing two classes, Random
and Arrays
, from the java.util
library.
For example,
import java.util.Arrays;
import java.util.Random;
public class ShuffleExample {
static void rand(int array[], int a) {
// Creating object for Random class
Random rd = new Random();
// Starting from the last element and swapping one by one.
for (int i = a - 1; i > 0; i--) {
// Pick a random index from 0 to i
int j = rd.nextInt(i + 1);
// Swap array[i] with the element at random index
int temp = array[i];
array[i] = array[j];
array[j] = temp;
}
}
public static void main(String[] args) {
int[] ar = {1, 2, 3, 4, 5, 6, 7, 8};
int b = ar.length;
rand(ar, b);
// Printing the random generated array
System.out.println(Arrays.toString(ar));
}
}
Output:
[5, 4, 6, 2, 8, 1, 7, 3]
Now, let’s break down the code into its components and explain how it shuffles an array:
Import Required Classes
import java.util.Arrays;
import java.util.Random;
In this section, we import two classes, Random
and Arrays
, from the java.util
library. The Random
class is used for generating random numbers, while the Arrays
class is used for printing the shuffled array.
Create a Shuffle Method
static void static void rand(int array[], int a) {
// Creating object for Random class
Random rd = new Random();
// Starting from the last element and swapping one by one.
for (int i = a - 1; i > 0; i--) {
// Pick a random index from 0 to i
int j = rd.nextInt(i + 1);
// Swap array[i] with the element at random index
int temp = array[i];
array[i] = array[j];
array[j] = temp;
}
}
The rand
method performs the actual shuffling. Here’s how it works:
- We create an instance of the
Random
class to generate random numbers. - We iterate through the array in reverse order, starting from the last element down to the first element (
0
). - For each element, we generate a random index
j
between0
andi
(inclusive), wherei
is the current index. - We swap the current element
array[i]
with the element at the randomly chosen indexarray[j]
.
Shuffle the Array
public static void main(String[] args) {
int[] ar = {1, 2, 3, 4, 5, 6, 7, 8};
int b = ar.length;
rand(ar, b);
// Printing the random generated array
System.out.println(Arrays.toString(ar));
}
In the main
method:
- We create an array named
ar
with the elements we want to shuffle. - We calculate the length of the array using
ar.length
. - We call the
rand
method to shuffle the array. - Finally, we print the shuffled array using
System.out.println
.
Use the shuffle()
Method to Shuffle an Array in Java
The shuffle()
function of the Collection
class takes a list given by the user and shuffles it randomly. This function is easy to use and takes lesser time than the previous method. Also, it reduces the line of codes for us.
We take an array and first convert it into a list. Then, we use the shuffle()
function to shuffle this list. Finally, we change this list back to an array and print it.
Here’s the complete Java code to shuffle an array using the shuffle()
method:
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collections;
public class ArrayShuffler {
public static void main(String[] args) {
Integer[] arrayToShuffle = {1, 2, 3, 4, 5, 6, 7, 8};
// Convert the array to a list
ArrayList<Integer> list = new ArrayList<>(Arrays.asList(arrayToShuffle));
// Shuffle the list using Collections.shuffle()
Collections.shuffle(list);
// Convert the shuffled list back to an array
Integer[] shuffledArray = list.toArray(new Integer[0]);
// Print the shuffled array
System.out.println("Shuffled Array: " + Arrays.toString(shuffledArray));
}
}
Code Explanation
Now, let’s break down the code into its components and explain how it shuffles an array:
Import Required Classes
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collections;
In this section, we import three classes: ArrayList
, Collections
, and Arrays
. ArrayList
is used to create a list from the array, Collections
provides the shuffle()
method, and Arrays
is used to convert the list back to an array.
Convert the Array to a List
Integer[] arrayToShuffle = {1, 2, 3, 4, 5, 6, 7, 8};
// Convert the array to a list
ArrayList<Integer> list = new ArrayList<>(Arrays.asList(arrayToShuffle));
We create an ArrayList
named list
and use Arrays.asList(arrayToShuffle)
to convert the arrayToShuffle
array into a list.
Shuffle the List
// Shuffle the list using Collections.shuffle()
Collections.shuffle(list);
We use Collections.shuffle(list)
to shuffle the elements in the list
randomly.
Convert the Shuffled List Back to an Array
// Convert the shuffled list back to an array
Integer[] shuffledArray = list.toArray(new Integer[0]);
We convert the shuffled list
back to an array named shuffledArray
using the toArray()
method.
Print the Shuffled Array
// Print the shuffled array
System.out.println("Shuffled Array: " + Arrays.toString(shuffledArray));
Finally, we print the shuffled array using System.out.println()
.
Use the Arrays.sort()
Method with A Custom Comparator to Shuffle an Array in Java
Java’s Arrays.sort()
method is primarily used for sorting arrays. However, by providing a custom comparator, you can use it to shuffle an array as well. The basic idea is to generate random comparison results between elements in the array, causing them to be rearranged randomly.
Here’s how you can shuffle an array using Arrays.sort()
with a custom comparator:
- Create a custom comparator that generates random comparison results.
- Use the
Arrays.sort()
method, passing in the array and the custom comparator. - The
Arrays.sort()
method will rearrange the elements in the array based on the custom comparator’s logic, effectively shuffling the array.
Complete Code
Here’s a complete Java program that demonstrates how to shuffle an array using Arrays.sort()
with a custom comparator:
import java.util.Arrays;
import java.util.Comparator;
import java.util.Random;
public class ArrayShuffleWithCustomComparator {
public static void main(String[] args) {
// Create an array of strings
String[] array = {"apple", "banana", "cherry", "date", "fig"};
// Shuffle the array
shuffleArray(array);
// Print the shuffled array
System.out.println(Arrays.toString(array));
}
// Custom comparator for shuffling
static class ShuffleComparator implements Comparator<Object> {
private final Random random = new Random();
@Override
public int compare(Object o1, Object o2) {
// Generate random comparison results (-1, 0, 1)
return Integer.compare(random.nextInt(3) - 1, 0);
}
}
// Function to shuffle an array using Arrays.sort() with custom comparator
static <T> void shuffleArray(T[] array) {
Arrays.sort(array, new ShuffleComparator());
}
}
Code Explanation
Let’s break down the code and understand how it works:
- We start by creating an array of strings called
array
. This is the array we want to shuffle. - We define a custom comparator class named
ShuffleComparator
. This class implements theComparator
interface and overrides thecompare()
method. - Inside the
ShuffleComparator
class:- We create a
Random
object namedrandom
to generate random numbers.
- We create a
- In the
compare()
method:- We generate random comparison results by subtracting 1 from a random integer between 0 and 2 (
nextInt(3) - 1
). This generates values of -1, 0, or 1 with roughly equal probability.
- We generate random comparison results by subtracting 1 from a random integer between 0 and 2 (
- We call the
shuffleArray()
function, passing thearray
as an argument. This function usesArrays.sort()
with the custom comparator to shuffle the elements. - Finally, we print the shuffled array.
Use the List
and ListIterator
to Shuffle an Array in Java
One elegant and flexible way to shuffle an array in Java is to use the List
interface along with ListIterator
.
The process of shuffling an array using List
and ListIterator
involves the following steps:
Step 1: Convert the Array to a List
To use ListIterator
, we first need to convert our array into a List
. Java provides a utility method Arrays.asList(T... a)
for this purpose. Here’s how you can do it:
import java.util.*;
public class ArrayShuffleUsingListIterator {
public static void main(String[] args) {
// Create an array
Integer[] array = {1, 2, 3, 4, 5};
// Convert the array to a List
List<Integer> list = new ArrayList<>(Arrays.asList(array));
}
}
In this code snippet, we have an array of integers, and we convert it into a List<Integer>
named list
.
Step 2: Shuffle the List
Next, we shuffle the List
using Collections.shuffle(List<?> list)
method, which randomly permutes the elements within the List
. Here’s how to do it:
Collections.shuffle(list);
After this step, the elements in the list
are shuffled randomly.
Step 3: Convert the List Back to an Array
To get the shuffled elements back into an array, we need to create a new array and copy the elements from the shuffled List
to the array. Here’s how you can achieve this:
// Create a new array of the same type and size as the original array
Integer[] shuffledArray = new Integer[list.size()];
// Copy the shuffled elements from the List to the array
list.toArray(shuffledArray);
Now, shuffledArray
contains the elements of the original array but in a shuffled order.
Full Code Example
Here’s the complete Java program that shuffles an array using List
and ListIterator
:
import java.util.*;
public class ArrayShuffleUsingListIterator {
public static void main(String[] args) {
// Create an array
Integer[] array = {1, 2, 3, 4, 5};
// Convert the array to a List
List<Integer> list = new ArrayList<>(Arrays.asList(array));
// Shuffle the List
Collections.shuffle(list);
// Create a new array of the same type and size as the original array
Integer[] shuffledArray = new Integer[list.size()];
// Copy the shuffled elements from the List to the array
list.toArray(shuffledArray);
// Print the shuffled array
System.out.println("Shuffled Array: " + Arrays.toString(shuffledArray));
}
}
In this code snippet, we have an array of integers, and we convert it into a List<Integer>
named list
.
Step 3: Shuffle the List
Next, we shuffle the List
using Collections.shuffle(List<?> list, Random rnd)
method from the java.util.Collections
class. However, instead of using a traditional Random
object, we use ThreadLocalRandom.current()
as the source of randomness. Here’s how to do it:
Collections.shuffle(list, ThreadLocalRandom.current());
After this step, the elements in the list
are shuffled randomly.
Step 4: Convert the List Back to an Array
To get the shuffled elements back into an array, we need to create a new array and copy the elements from the shuffled List
to the array. Here’s how you can achieve this:
// Create a new array of the same type and size as the original array
Integer[] shuffledArray = new Integer[list.size()];
// Copy the shuffled elements from the List to the array
list.toArray(shuffledArray);
Now, shuffledArray
contains the elements of the original array but in a shuffled order.
Full Code Example
Here’s the complete Java program that shuffles an array using ThreadLocalRandom
:
import java.util.*;
import java.util.concurrent.ThreadLocalRandom;
public class ArrayShuffleUsingThreadLocalRandom {
public static void main(String[] args) {
// Create an array
Integer[] array = {1, 2, 3, 4, 5};
// Convert the array to a List
List<Integer> list = new ArrayList<>(Arrays.asList(array));
// Shuffle the List using ThreadLocalRandom
Collections.shuffle(list, ThreadLocalRandom.current());
// Create a new array of the same type and size as the original array
Integer[] shuffledArray = new Integer[list.size()];
// Copy the shuffled elements from the List to the array
list.toArray(shuffledArray);
// Print the shuffled array
System.out.println("Shuffled Array: " + Arrays.toString(shuffledArray));
}
}
When you run this program, you will see the elements of the original array printed in a shuffled order.
Conclusion
Shuffling an array is a common operation in Java development, and understanding multiple techniques for achieving this task is valuable.
Depending on your specific requirements and the Java version you’re using, you can choose the method that best suits your needs.
Whether you opt for the simplicity of the random()
method, the convenience of the shuffle()
method, the customization of Arrays.sort()
, the flexibility of List
and ListIterator
, or the thread safety and performance of ThreadLocalRandom
, these techniques offer a wide range of options for shuffling arrays in Java.