How to Shift an Array in Java
-
Use the
for
Loop and atemp
Variable to Shift an Array in Java -
Use the
skip()
Method to Shift an Array in Java 8 -
Use the
Collections.rotate()
Method to Shift an Array in Java - Use a Temporary Array to Shift an Array in Java
- Use In-Place Rotation to Shift an Array in Java
- Conclusion
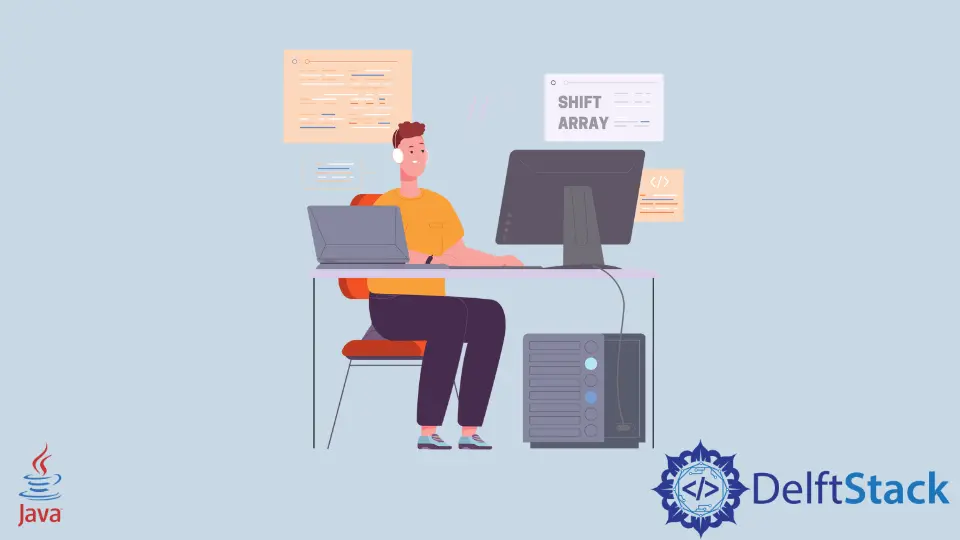
Arrays are fundamental data structures in Java, and there are various scenarios where shifting elements within an array become a necessity. Whether you’re implementing circular rotations, reordering, or any other operation that requires changing the position of array elements, Java provides several methods to accomplish this task.
In this article, we’ll explore different techniques for shifting elements in an array, each with its own merits and use cases.
Use the for
Loop and a temp
Variable to Shift an Array in Java
One straightforward approach to shift elements in an array is by using a for
loop along with a temporary variable (temp
).
The basic idea behind using a for
loop and a temp
variable is to iteratively shift each element to its next position. This involves temporarily storing the value of the last element in the array and then moving each element to the right by one position.
The temporary variable (temp
) serves as a placeholder for the last element, ensuring that it is not lost during the shifting process.
Let’s dive into the code to better illustrate the concept:
import java.util.Arrays;
public class ArrayShiftExample {
public static void main(String[] args) {
int[] array = {1, 2, 3, 4, 5};
int shift = 2;
System.out.println("Original Array: " + Arrays.toString(array));
for (int s = 0; s < shift; s++) {
int temp = array[array.length - 1];
for (int i = array.length - 1; i > 0; i--) {
array[i] = array[i - 1];
}
array[0] = temp;
}
System.out.println("Shifted Array: " + Arrays.toString(array));
}
}
Output:
Original Array: [1, 2, 3, 4, 5]
Shifted Array: [4, 5, 1, 2, 3]
In the provided example, we start with the declaration of an integer array array
and specify the desired shift amount (shift
).
The outer for
loop (for (int s = 0; s < shift; s++)
) controls the number of shifts.
On the other hand, the inner for
loop (for (int i = array.length - 1; i > 0; i--)
) is responsible for moving each element to the right.
The temp
variable is crucial in preserving the value of the last element (temp = array[array.length - 1]
). Inside the inner loop, each element is shifted to the right, effectively moving the entire array to the right by one position.
Finally, the first element is assigned the value stored in the temp
variable, completing the shift.
The output of the shifted array is then displayed using System.out.println
.
Use the skip()
Method to Shift an Array in Java 8
In Java, an alternative method for shifting elements within an array involves utilizing the skip()
method. This method, part of the Stream API introduced in Java 8, is used here to skip a specified number of elements in the array.
By creating a stream from the array, we can seamlessly skip the desired number of elements and concatenate the remaining ones to achieve the shift. This method provides a more functional and expressive way to handle array shifts compared to traditional loop-based approaches.
Here’s an example:
import java.util.Arrays;
public class ArrayShifter {
public static void main(String[] args) {
int[] array = {1, 2, 3, 4, 5};
int shift = 2;
System.out.println("Original Array: " + Arrays.toString(array));
int[] shiftedArray = Arrays.stream(array).skip(shift).toArray();
System.out.println("Shifted Array: " + Arrays.toString(shiftedArray));
}
}
Output:
Original Array: [1, 2, 3, 4, 5]
Shifted Array: [3, 4, 5]
In this example, we begin with an array (array
) containing elements and specify the desired shift value (shift
). Leveraging the Stream API, we transform the array into a stream using Arrays.stream(array)
.
The skip(shift)
method is then applied to skip the first shift
elements in the stream.
The result is a stream that contains the elements after the specified skip count. The toArray()
method is then invoked to convert the stream back into an array.
The shifted array is now ready for use.
Finally, the shifted array is displayed using Arrays.toString(shiftedArray)
. This method offers a concise and expressive way to shift elements within an array, showcasing the power and versatility of the Stream API in Java.
It’s particularly useful when a more functional programming style is preferred over traditional loop-based solutions.
Use the Collections.rotate()
Method to Shift an Array in Java
Achieving a seamless rotation of array elements in Java is made remarkably simple with the Collections.rotate()
method. This method, part of the Collections
framework, streamlines the process of rotating elements within an array while maintaining their order.
The Collections.rotate()
method is designed to operate on a list, making it an excellent choice for scenarios where a List implementation is permissible. By converting an array to a List and applying this method, we can effortlessly achieve array rotation.
The method accepts the list to be rotated and the distance of rotation as parameters, efficiently handling the intricacies of element reordering.
See the example below.
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
public class ArrayRotator {
public static void main(String[] args) {
Integer[] array = {1, 2, 3, 4, 5};
int rotation = 2;
System.out.println("Original Array: " + Arrays.toString(array));
List<Integer> list = Arrays.asList(array);
Collections.rotate(list, rotation);
Integer[] rotatedArray = list.toArray(new Integer[0]);
System.out.println("Rotated Array: " + Arrays.toString(rotatedArray));
}
}
Output:
Original Array: [1, 2, 3, 4, 5]
Rotated Array: [4, 5, 1, 2, 3]
In this example, we begin with an array (array
) and specify the desired rotation value (rotation
). To make use of Collections.rotate()
, we first convert the array to a List using Arrays.asList(array)
.
This List is then passed to the rotate()
method along with the rotation distance.
The magic happens within the rotate()
method, which intelligently reorders the elements of the List based on the rotation distance. After rotation, the List is converted back to an array using list.toArray(new Integer[0])
.
The final rotated array is displayed using Arrays.toString(rotatedArray)
. This method illustrates the simplicity and elegance of using Collections.rotate()
for array rotation, showcasing how Java’s Collections
framework provides powerful tools for array manipulation without sacrificing ease of use.
Use a Temporary Array to Shift an Array in Java
When it comes to shifting elements within an array in Java while maintaining their order, employing a temporary array proves to be a reliable and straightforward technique. This method involves creating a new array, copying elements with the desired shift, and then reassigning the modified array back to the original.
The methodology here revolves around the creation of a new array (newArray
) with the same length as the original array.
By using a for
loop, each element is copied to the new array at an index calculated to achieve the desired shift. This process ensures that the original order is preserved while introducing the necessary shift.
Finally, the modified array is reassigned to the original, completing the shift.
Here’s an example code:
import java.util.Arrays;
public class ArrayShifter {
public static void main(String[] args) {
int[] array = {1, 2, 3, 4, 5};
int shift = 2;
int[] newArray = new int[array.length];
System.out.println("Original Array: " + Arrays.toString(array));
for (int i = 0; i < array.length; i++) {
int newIndex = (i + shift) % array.length;
newArray[newIndex] = array[i];
}
array = newArray;
System.out.println("Shifted Array: " + Arrays.toString(array));
}
}
Output:
Original Array: [1, 2, 3, 4, 5]
Shifted Array: [4, 5, 1, 2, 3]
Beginning with an array (array
) and specifying the desired shift value (shift
), we initialize a new array (newArray
) with the same length as the original. The for
loop iterates through each element in the array, calculating the new index for each element based on the desired shift.
Elements are then copied to the new array at the calculated index.
Once the loop completes, the original array is reassigned to the modified array (array = newArray
). The final shifted array is then displayed using Arrays.toString(array)
.
Use In-Place Rotation to Shift an Array in Java
Achieving in-place rotation of array elements in Java is an efficient approach that eliminates the need for additional arrays. This method involves using a temporary variable and a loop structure to systematically rotate the elements within the array.
The in-place rotation method leverages a for
loop to iterate through the array elements. A temporary variable (temp
) is used to store the value of the current element, and the loop repositions each element to its correct rotated position.
This process continues until all elements have been correctly rotated. In order to ensure a complete rotation, the loop continues until the greatest common divisor (GCD) of the rotation distance and the array length is reached.
Let’s have example:
import java.util.Arrays;
public class ArrayRotator {
public static void main(String[] args) {
int[] array = {1, 2, 3, 4, 5};
int rotation = 2;
int n = array.length;
System.out.println("Original Array: " + Arrays.toString(array));
for (int i = 0; i < gcd(rotation, n); i++) {
int temp = array[i];
int j = i;
while (true) {
int k = (j + rotation) % n;
if (k == i)
break;
array[j] = array[k];
j = k;
}
array[j] = temp;
}
System.out.println("Rotated Array: " + Arrays.toString(array));
}
private static int gcd(int a, int b) {
if (b == 0)
return a;
return gcd(b, a % b);
}
}
Output:
Original Array: [1, 2, 3, 4, 5]
Rotated Array: [3, 4, 5, 1, 2]
Beginning with an array (array
) and specifying the desired rotation value (rotation
), a variable n
is assigned the length of the array. The for
loop iterates through the array until the GCD of the rotation distance and array length is reached.
Within the loop, a temporary variable (temp
) captures the value of the current element at index i
.
A second while
loop calculates the new index (k
) for each element based on the rotation distance. Elements are then repositioned until the original index (i
) is reached, completing the rotation for that cycle.
This process continues until all elements have been correctly rotated. The final rotated array is displayed using Arrays.toString(array)
.
Conclusion
Choosing the right method for shifting elements in an array depends on the specific requirements of your task. Whether you prioritize precision, memory efficiency, or in-place operations, Java offers a variety of tools to cater to your needs.
By understanding these techniques and their applications, you can confidently handle array element shifting in a way that best suits your programming goals.
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn