How to Use the setAlignment Method in JavaFX
-
Use the
setAlignment()
Method in JavaFX -
Use the
Hbox.setAlignment()
andVbox.setAlignment()
Method in JavaFX -
Use the
Gridpane.setAlignment()
Method in JavaFX - Conclusion
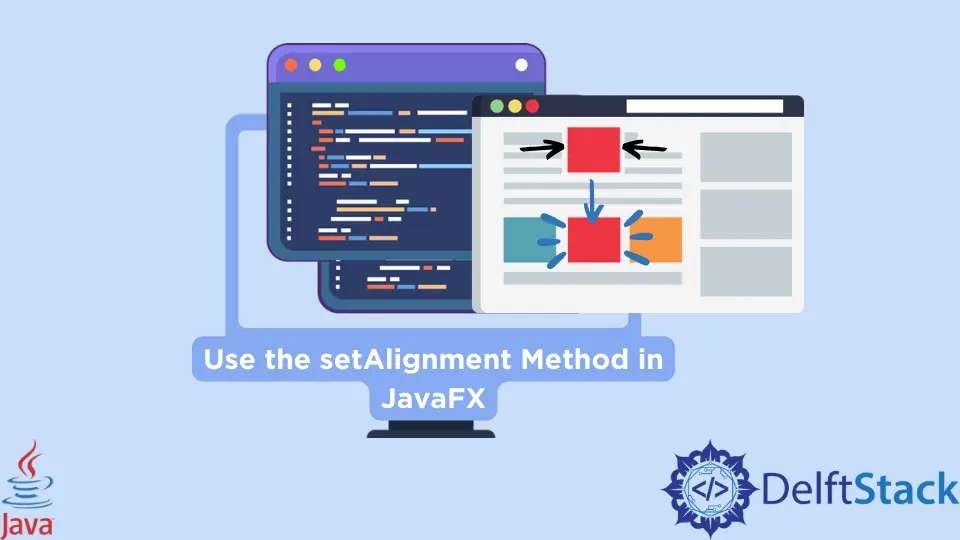
In this tutorial, we will demonstrate how to utilize the setAlignment
method in JavaFX.
setAlignment()
plays a vital role in JavaFX, offering precise control over the positioning of nodes within layouts. It empowers developers to craft visually attractive and well-organized user interfaces by defining the alignment of nodes within their designated containers.
In this article, we will explore the setAlignment()
method in layout classes that we can utilize to create visually pleasing user interfaces.
Use the setAlignment()
Method in JavaFX
The setAlignment()
method in JavaFX is used to specify the alignment of content within a layout or container. It takes parameters such as Pos.CENTER
, Pos.TOP_LEFT
, etc., to determine how the content should be positioned within its allocated space.
This method provides a quick way to control the positioning of UI elements, ensuring a consistent and visually appealing layout in JavaFX applications.
To use the setAlignment()
methods, you typically need to import classes from the javafx.scene.layout
package, as this package contains the layout classes such as HBox
, VBox
, BorderPane
, GridPane
, etc., which have the setAlignment()
methods.
Make sure that you have the JavaFX library added to your project.
If you are using Java 8, JavaFX might be bundled with the JDK. If you are using a later version of Java, you might need to add the JavaFX libraries to your project manually.
Remember that starting from Java 11, JavaFX is no longer included in the JDK by default, and you need to include it as a separate module or use a standalone JavaFX SDK.
In JavaFX, the setAlignment()
method is typically associated with layout classes, and it is used to set the alignment of a node within its layout bounds. The specific methods and their usage can vary depending on the layout class.
Here are examples of some common layout classes:
Layout Manager | Definition | Alignment Method | Example |
---|---|---|---|
HBox |
Horizontal layout for nodes left to right. | HBox.setAlignment(Node child, Pos value) |
java HBox hbox = new HBox(); hbox.setAlignment(Pos.CENTER); |
VBox |
Vertical layout for nodes top to bottom. | VBox.setAlignment(Node child, Pos value) |
java VBox vbox = new VBox(); vbox.setAlignment(Pos.CENTER); |
BorderPane |
Divides space into five regions: top, bottom, left, right, center. | BorderPane.setAlignment(Node child, Pos value) |
java BorderPane borderPane = new BorderPane(); borderPane.setTop(nodeTop); BorderPane.setAlignment(nodeTop, Pos.CENTER); |
GridPane |
Grid-based layout for arranging nodes in cells. | GridPane.setHalignment(Node child, HPos value) GridPane.setValignment(Node child, VPos value) |
java GridPane gridPane = new GridPane(); Button button = new Button("Click me"); gridPane.add(button, 0, 0); GridPane.setHalignment(button, HPos.CENTER); GridPane.setValignment(button, VPos.CENTER); |
These methods allow you to control the alignment of nodes within their respective layout containers.
The Pos
enumeration is used to specify the position, and it includes constants like Pos.CENTER
, Pos.TOP_LEFT
, etc. The HPos
and VPos
enumerations are used for horizontal and vertical alignment in GridPane
.
The apparent difference in the number of alignments between GridPane
, HBox
, and VBox
is due to the nature of the layouts and the purposes they serve.
In contrast, HBox
and VBox
are simpler layout panes that primarily deal with a single dimension: horizontal for HBox
and vertical for VBox
. In these cases, the alignments are in only one dimension, so there are more possibilities for combinations.
Now, let’s see the total view of our example code. You can copy and paste the code below to have a test run.
import javafx.application.Application;
import javafx.geometry.HPos;
import javafx.geometry.Pos;
import javafx.geometry.VPos;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.Label;
import javafx.scene.layout.BorderPane;
import javafx.scene.layout.GridPane;
import javafx.scene.layout.HBox;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class HelloApplication extends Application {
@Override
public void start(Stage primaryStage) {
primaryStage.setTitle("Node Alignment Example");
// Create buttons
Button button1 = new Button("GB 1");
Button button2 = new Button("GB 2");
Button button3 = new Button("GB 3");
Button button4 = new Button("HBOX 1");
Button button5 = new Button("HBOX 2");
Button button6 = new Button("HBOX 3");
Label label1 = new Label("Vertical 1");
Label label2 = new Label("Vertical 2");
Label label3 = new Label("Vertical 3");
Label topLabel = new Label("BP Top");
Label centerLabel = new Label("BP Center");
Label bottomLabel = new Label("BP Bottom");
// Horizontal Box (HBox) Example
HBox hbox = new HBox(10); // 10 is the spacing between nodes
hbox.getChildren().addAll(button4, button5, button6);
// Set alignment for all nodes in the HBox
hbox.setAlignment(Pos.TOP_RIGHT); // Center alignment horizontally
// Vertical Box (VBox) Example
VBox vbox = new VBox(10); // 10 is the spacing between nodes
vbox.getChildren().addAll(label1, label2, label3);
// Set alignment for all nodes in the VBox
vbox.setAlignment(Pos.CENTER); // Center alignment vertically
// GridPane Example
GridPane gridPane = new GridPane();
gridPane.add(button1, 0, 0);
gridPane.add(button2, 1, 0);
gridPane.add(button3, 0, 1);
// Set horizontal alignment for a specific node in the GridPane
GridPane.setHalignment(button1, HPos.RIGHT); // Align to the right
// Set vertical alignment for a specific node in the GridPane
GridPane.setValignment(button2, VPos.BOTTOM); // Align to the bottom
// Create a BorderPane
BorderPane borderPane = new BorderPane();
// Set alignment for each region
BorderPane.setAlignment(topLabel, Pos.CENTER);
BorderPane.setAlignment(centerLabel, Pos.CENTER_LEFT);
BorderPane.setAlignment(bottomLabel, Pos.BOTTOM_RIGHT);
// Add nodes to the BorderPane
borderPane.setTop(topLabel);
borderPane.setCenter(centerLabel);
borderPane.setBottom(bottomLabel);
// Create scenes
Scene hboxScene = new Scene(hbox, 300, 100);
Scene vboxScene = new Scene(vbox, 100, 300);
Scene gridPaneScene = new Scene(gridPane, 200, 200);
Scene bpscene = new Scene(borderPane, 400, 300);
// Set the stage
// Uncomment the following lines to see the other layouts
primaryStage.setScene(hboxScene);
// primaryStage.setScene(vboxScene);
// primaryStage.setScene(gridPaneScene);
// primaryStage.setScene(bpscene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
This JavaFX code creates a graphical user interface with different layouts: an HBox
, a VBox
, a GridPane
, and a BorderPane
. Each layout contains various UI elements, such as buttons and labels.
The scene displayed is determined by the active line (commented/uncommented) in primaryStage.setScene(...)
. In its current state, the HBox
scene is shown, aligning three buttons horizontally with a top-right alignment.
To explore other layouts, you can uncomment and comment on the corresponding lines in primaryStage.setScene(...)
, switching between HBox
, VBox
, GridPane
, and BorderPane
layouts.
Output:
![]() |
![]() |
---|---|
![]() |
![]() |
The output of the provided code displays a window with three buttons (HBOX 1
, HBOX 2
, HBOX 3
) aligned horizontally at the top-right corner using an HBox
layout. The buttons are arranged with a spacing of 10 units between them.
The VBox
scene displays three labeled nodes (Vertical 1
, Vertical 2
, and Vertical 3
) vertically aligned in the center with a spacing of 10 units between them. The labels are arranged in a column, showcasing a vertical layout.
The GridPane
output shows three buttons (GB 1
, GB 2
, GB 3
) arranged in a grid. The first button (GB 1
) is aligned to the right, and the second button (GB 2
) is aligned to the bottom within the grid, demonstrating specific node alignments in a GridPane
layout.
The BorderPane
output shows a graphical user interface with labeled regions (Top
, Center
, Bottom
). The labels BP Top
, BP Center
, and BP Bottom
are positioned according to their specified alignments (Top: Center
, Center: Left
, Bottom: Right
) within the BorderPane
layout.
Use the Hbox.setAlignment()
and Vbox.setAlignment()
Method in JavaFX
HBox.setAlignment()
and VBox.setAlignment()
in JavaFX set the alignment of child nodes within an HBox
(horizontal layout) and VBox
(vertical layout), respectively.
Using parameters like Pos.CENTER
or Pos.TOP_LEFT
, these methods control the positioning of elements within the box, ensuring a cohesive and organized arrangement.
These alignments are commonly used in HBox
and VBox
layouts to control the positioning of child nodes within the available space:
Alignment | Description |
---|---|
Pos.CENTER |
Centers the node within its layout bounds. |
Pos.TOP_LEFT |
Aligns the node to the top-left corner. |
Pos.TOP_CENTER |
Aligns the node to the top-center. |
Pos.TOP_RIGHT |
Aligns the node to the top-right corner. |
Pos.CENTER_LEFT |
Centers the node vertically and aligns it to the left. |
Pos.CENTER_RIGHT |
Centers the node vertically and aligns it to the right. |
Pos.BOTTOM_LEFT |
Aligns the node to the bottom-left corner. |
Pos.BOTTOM_CENTER |
Aligns the node to the bottom-center. |
Pos.BOTTOM_RIGHT |
Aligns the node to the bottom-right corner. |
Use the Gridpane.setAlignment()
Method in JavaFX
GridPane.setAlignment()
in JavaFX adjusts the alignment of child nodes within a GridPane
, a grid-based layout manager. It allows precise control over the positioning of elements both horizontally (HPos
) and vertically (VPos
).
By specifying alignment parameters such as HPos.CENTER
or VPos.BOTTOM
, developers can easily organize and structure the layout of UI components within the grid, ensuring a visually appealing and well-organized user interface in JavaFX applications.
In a GridPane
, you have both horizontal (HPos
) and vertical (VPos
) alignments, and each of these can be combined, resulting in a total of 6 possible alignments.
Horizontal Alignment (GridPane.setHalignment()
):
Alignment | Description |
---|---|
HPos.CENTER |
Centers the node horizontally within its grid cell. |
HPos.LEFT |
Aligns the node to the left of its grid cell. |
HPos.RIGHT |
Aligns the node to the right of its grid cell. |
HPos.BASELINE_LEFT |
Aligns the node to the left baseline of its cell. |
HPos.BASELINE_CENTER |
Aligns the node to the center baseline of its cell. |
HPos.BASELINE_RIGHT |
Aligns the node to the right baseline of its cell. |
Vertical Alignment (GridPane.setValignment()
):
Alignment | Description |
---|---|
VPos.CENTER |
Centers the node vertically within its grid cell. |
VPos.TOP |
Aligns the node to the top of its grid cell. |
VPos.BOTTOM |
Aligns the node to the bottom of its grid cell. |
VPos.BASELINE |
Aligns the node to the baseline of its cell. |
VPos.CENTER_BASELINE |
Centers the node both vertically and on the baseline of its cell. |
These alignments are commonly used in GridPane
layouts to specify the horizontal and vertical positioning of child nodes within their respective grid cells.
Conclusion
setAlignment()
is crucial in JavaFX for precise control over node positioning within layouts. It enables developers to create visually appealing and organized user interfaces by specifying the alignment of nodes within their respective containers.
HBox
in JavaFX is a horizontal layout that arranges nodes in a single row.
VBox
in JavaFX is a vertical layout that organizes nodes in a single column.
BorderPane
in JavaFX is a versatile layout that divides the container into five regions: top, bottom, left, right, and center.
GridPane
in JavaFX is a grid-based layout that enables precise control over the positioning of nodes in rows and columns.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn