JavaFX で setAlignment メソッドを使用する
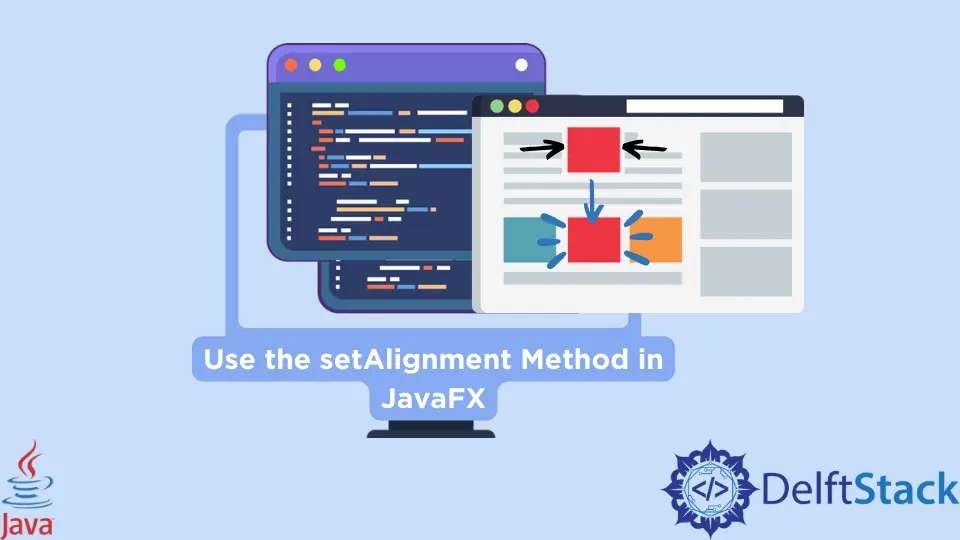
HBox は、すべてのコンポーネントを配置するレイアウト コンポーネントです。 デフォルトでは、すべてのコンポーネントが横一列に編成されますが、左、右、中央、上、ボタンなどに揃える必要がある場合があります。
この記事では、HBox
を独自の形式で配置する方法を説明します。 例を見て、理解しやすくするために行ごとに説明します。
JavaFX で setAlignment()
メソッドを使用する
この例で使用するライブラリは次のとおりです。
import javafx.application.Application; // Package for the whole application
import javafx.geometry.Pos; // Geometric positioning Package for the variable "Pos"
import javafx.scene.Scene; // Package for the scene
import javafx.scene.control.Button; // Package for the button
import javafx.scene.layout.HBox; // The HBox Package
import javafx.stage.Stage; // Package for the stage
これらは、含める必要がある必要なライブラリであり、その目的はコードにコメントされています。 それでは、コード全体を説明します。
主なコードは以下のようになり、各行の目的はその横にコメントされています。
public class FxHBox extends Application { // Our main class extends to the Application class
Button buttonOne, buttonTwo; // Declared two buttons
HBox _hbox; // Declared a HBox
Scene scene; // Declared a scene
public void start(Stage BasicStage)
throws Exception { // We have to use Exception Handling here as it can generate errors if
// anything goes wrong.
BasicStage.setTitle("HBox Set Allignment"); // Set the title of the application
buttonOne = new Button("Button 1"); // Created button 1 with title
buttonTwo = new Button("Button 2"); // Created button 2 with title
_hbox = new HBox(buttonOne,
buttonTwo); // Created an HBox element that holds the two buttons buttonOne & buttonTwo
_hbox.setAlignment(
Pos.CENTER); // Here we are customizing the default alignment and place our componet in
// center. Here 'Pos' provides the geometic allignment.
scene = new Scene(_hbox, 400, 300); // Creates the scene with necessary heights and weights
BasicStage.setScene(scene); // Set the scene
BasicStage.show(); // Visualize the scene
}
public static void main(String[] args) {
Application.launch(args); // Launch the application
}
}
ここで注目すべき点は、setAlignment()
メソッドの使用方法です。 これにより、Hbox の位置をカスタマイズできます。
要素に提供できるさまざまな配置があります。 これらを以下に示します。
ポジション | 説明 |
---|---|
Pos.BASELINE_LEFT |
垂直方向に整列 ベースライン 水平方向に整列 左 |
Pos.BASELINE_CENTER |
垂直方向に整列 ベースライン 水平方向に整列 中央 |
Pos.BASELINE_RIGHT |
縦揃え ベースライン 横揃え 右 |
Pos.BOTTOM_LEFT |
縦揃え 下 横揃え 左 |
Pos.BOTTOM_CENTER |
縦に下揃え 横に揃えて中央 |
Pos.BOTTOM_RIGHT |
縦揃え 下 横揃え 右 |
Pos.CENTER_LEFT |
縦に中央揃え 横に揃えて左 |
Pos.CENTER |
縦に中央揃え 横に中央揃え |
Pos.CENTER_RIGHT |
縦揃え 中央 横揃え 右 |
Pos.TOP_LEFT |
上を縦に揃える 左を横に揃える |
Pos.TOP_CENTER |
縦に上揃え 横に中央揃え |
Pos.TOP_RIGHT |
縦に上揃え 横に右揃え |
それでは、サンプル コードとその出力の全体図を見てみましょう。 以下のコードをコピーして貼り付けて、テストを実行できます。
import javafx.application.Application;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.HBox;
import javafx.stage.Stage;
public class FxHBox extends Application {
Button buttonOne, buttonTwo;
HBox _hbox;
Scene scene;
public void start(Stage BasicStage) throws Exception {
BasicStage.setTitle("HBox Set Allignment");
buttonOne = new Button("Button 1");
buttonTwo = new Button("Button 2");
_hbox = new HBox(buttonOne, buttonTwo);
_hbox.setAlignment(Pos.CENTER);
scene = new Scene(_hbox, 400, 300);
BasicStage.setScene(scene);
BasicStage.show();
}
public static void main(String[] args) {
Application.launch(args);
}
}
コンパイルと実行が成功すると、次のような出力が得られます。
IDE がライブラリの自動インクルードをサポートしていない場合は、コンパイルする前に必要なライブラリ ファイルを手動でインクルードする必要がある場合があります。
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn