JavaFX でオブジェクトを移動する
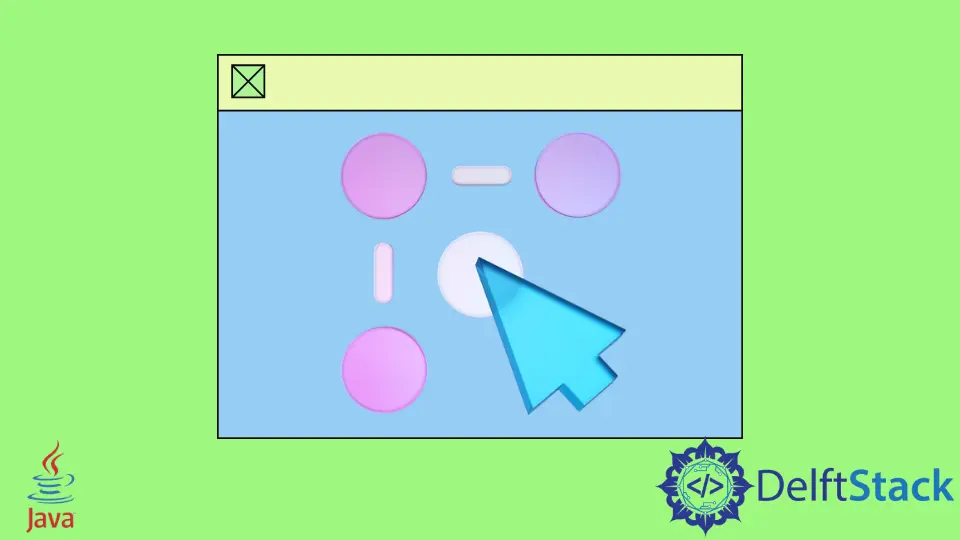
この記事では、オブジェクトを上下左右の 4 方向に動かします。 この目的のために、以下のコードを使用します。
JavaFX でオブジェクトを移動する
以下のコードを見てみましょう。 後で説明します。
// Importing necessary packages.
import javafx.application.Application;
import javafx.geometry.Insets;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.BorderPane;
import javafx.scene.layout.HBox;
import javafx.scene.layout.Pane;
import javafx.scene.paint.Color;
import javafx.scene.shape.Circle;
import javafx.stage.Stage;
public class MoveObject extends Application {
@Override public void start(Stage primaryStage) { // Our main method
BallPane BallPane = new BallPane();
HBox ButtonHolder = new HBox(5); // Create an Hbox named "ButtonHolder"
Button LeftBtn = new Button("Left"); // Create a button that move the ball left
Button RightBtn = new Button("Right"); // Create a button that move the ball Right
Button UpBtn = new Button("Up"); // Create a button that move the ball Up
Button DownBtn = new Button("Down"); // Create a button that move the ball Down
ButtonHolder.getChildren().addAll(
LeftBtn, RightBtn, UpBtn, DownBtn); // Contain all the button to the `ButtonHolder` Hbox
// Create actions for the buttons
LeftBtn.setOnAction(e -> BallPane.MoveLeft());
RightBtn.setOnAction(e -> BallPane.MoveRight());
UpBtn.setOnAction(e -> BallPane.MoveUp());
DownBtn.setOnAction(e -> BallPane.MoveDown());
BorderPane pane = new BorderPane(); // Create a BorderPane
pane.setCenter(BallPane); // Set the BallPane to pane
pane.setBottom(ButtonHolder); // Set the ButtonHolder to pane
pane.setPadding(new Insets(0, 20, 0, 20)); // Apply necessary paddings
BorderPane.setAlignment(ButtonHolder, Pos.CENTER); // Align the ButtonHolder
Scene scene = new Scene(pane, 250, 250); // Create a scene
primaryStage.setTitle("Move a Ball"); // Provide an application title "Move a Ball"
primaryStage.setScene(scene); // Set the scene to stage
primaryStage.show(); // Visualizing the stage.
}
public static void main(String[] args) {
Application.launch(args);
}
}
class BallPane extends Pane { // Create a Pane for pane
private double radius = 25; // Define the ball redius
private double width = 210; // Define the ball width
private double height = 210; // Define the ball height
Circle Ball = new Circle(width / 2, height / 2, radius); // Calcuate the circle
BallPane() { // Set ball properties
Ball.setFill(Color.GREEN);
Ball.setStroke(Color.BLACK);
getChildren().add(Ball);
}
public void MoveLeft() { // Method for moving the ball left
if (Ball.getRadius() < Ball.getCenterX()) {
Ball.setCenterX(Ball.getCenterX() - 10);
}
}
public void MoveRight() { // Method for moving the ball Right
if (Ball.getCenterX() < width - Ball.getRadius()) {
Ball.setCenterX(Ball.getCenterX() + 10);
}
}
public void MoveUp() { // Method for moving the ball Up
if (Ball.getRadius() < Ball.getCenterY()) {
Ball.setCenterY(Ball.getCenterY() - 10);
}
}
public void MoveDown() { // Method for moving the ball Down
if (Ball.getCenterY() < height - Ball.getRadius()) {
Ball.setCenterY(Ball.getCenterY() + 10);
}
}
}
コードの各行の目的については既にコメントしました。 ここで、その背後にあるアイデアについて説明します。
必要なパッケージをすべてインポートした後、primaryStage
という名前の Stage
を使用してメイン メソッドを作成しました。 また、コンテナに必要なボタンを保持する HBox も作成しました。
その後、ボタンのアクションを作成します。 そして、コードの他の部分はコマンドによって記述されました。
次に、オブジェクトを上下左右に動かすアクションの作業プロセスについて説明します。
public void MoveLeft() { // Method for moving the ball left
if (Ball.getRadius() < Ball.getCenterX()) {
Ball.setCenterX(Ball.getCenterX() - 10);
}
}
上記のメソッドは、ユーザーがこのボタンをクリックすると、オブジェクトの位置を左側に、または技術的には -x
方向に変更します。
public void MoveRight() { // Method for moving the ball Right
if (Ball.getCenterX() < width - Ball.getRadius()) {
Ball.setCenterX(Ball.getCenterX() + 10);
}
}
次に、上で共有したメソッドは、ユーザーがこのボタンをクリックすると、オブジェクトの位置を右側に、または技術的には +x
方向に変更します。
public void MoveUp() { // Method for moving the ball Up
if (Ball.getRadius() < Ball.getCenterY()) {
Ball.setCenterY(Ball.getCenterY() - 10);
}
}
その後、上で共有したメソッドは、ユーザーがこのボタンをクリックすると、オブジェクトの位置を上側に、または技術的には +y
方向に変更します。
public void MoveDown() { // Method for moving the ball Down
if (Ball.getCenterY() < height - Ball.getRadius()) {
Ball.setCenterY(Ball.getCenterY() + 10);
}
}
最後に、上記のメソッドは、ユーザーがこのボタンをクリックすると、オブジェクトの位置を下側、または技術的には -y
方向に変更します。
コードをコンパイルして実行すると、次のような出力が得られます。
出力:
IDE がライブラリの自動インクルードをサポートしていない場合は、覚えておいてください。 次に、コンパイルする前に、必要なライブラリ ファイルを手動で含める必要がある場合があります。 そうしないと、エラーが表示されます。
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn