JavaFX で KeyEvent を使用する
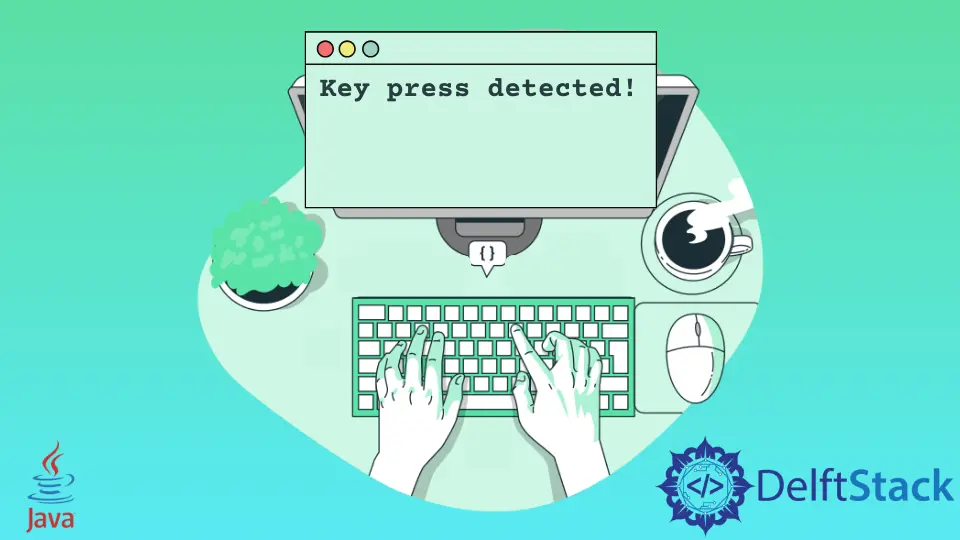
KeyEvent は、キーが押されたことを検出し、キーが押されたときに特定のコード ブロックを実行するために使用されます。
この記事では、キー イベントを作成し、ユーザーがキーを押したときに簡単なコードを実行する方法を示します。 また、理解しやすいように簡単な例も示します。
JavaFX で KeyEvent を使用する
キー イベントを作成するための一般的な形式を以下に示します。
scene.setOnKeyPressed(
(KeyEvent event)
-> {
// Create a key event that execute when any key pressed from your keyboard
// Add the actions that we are going to take when a key pressed.
});
ユーザーのキー押下を検出し、キーが押されたというメッセージを表示する例を見ていきます。
この例のソース コードは次のようになり、必要なコマンドを提供して各行を説明します。 みてみましょう。
import javafx.application.Application; // Package to create application
import javafx.scene.Scene; // Package to create a scene
import javafx.scene.control.Label; // Package to create a label
import javafx.scene.input.KeyEvent; // Package to create a key event
import javafx.scene.layout.HBox; // Package for the Hbox
import javafx.stage.Stage; // Package to create a stage
public class FxKeyListen extends Application {
Label lbl; // Declares a label
HBox _hbox; // Declares a HBox.
public void start(Stage SimpleStage) throws Exception {
SimpleStage.setTitle(
"Detect key event"); // Set the application title which is "Detect key event"
lbl =
new Label("No key is pressed !!!"); // Create a label with default text "No key pressed !!!"
_hbox = new HBox(lbl); // Create a HBox with a label
Scene scene = new Scene(_hbox, 400, 400); // Create a scene with HBox and necessary dimentions.
scene.setOnKeyPressed(
(KeyEvent event)
-> { // Create a key event that execute when any key pressed from your keyboard
lbl.setText("Key press detected !!!"); // The actions that we are going to take when a key
// pressed. In our case we are changing the label
// text to "Key press detected !!!"
});
SimpleStage.setScene(scene); // Set the scene
SimpleStage.show(); // Visualize the scent
}
public static void main(String[] args) {
Application.launch(args); // Run our application
}
}
私たちはすでに各行の目的を命じました。 それでは、コードがどのように機能するかを説明しましょう。
キーが押されていません!!!
というデフォルトのテキストを使用して、コードにラベルを作成しました。 行 lbl = new Label ("キーが押されていません!!!");
. このラベルは、キーが押されていないことをユーザーに示すことを目的としています。
次に、ユーザーがキーボードからキーを押したときに実行されるアクションとして、単純な keypress イベントを作成しました。 ラベルのデフォルト テキストを変更し、Key press detected !!!
に設定します。 したがって、この目的のために、コードは lbl.setText("Key press detected !!!");
のようなアクションとして追加します。
コンパイルが成功した後にコードを実行すると、Detect key event
というタイトルのウィンドウが開き、キーボードからキーを押すと、以下のような出力が表示されます。
出力:
IDE がライブラリの自動インクルードをサポートしていない場合は、覚えておいてください。 次に、コンパイルする前に、必要なライブラリ ファイルを手動で含める必要がある場合があります。
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn