How to Set One Array Equal to Another in Java
- Set One Array Equal to Another in Java Using a Loop
-
Set One Array Equal to Another in Java Using
System.arraycopy
-
Set One Array Equal to Another in Java Using
Arrays.copyOf
-
Set One Array Equal to Another in Java Using
Arrays.copyOfRange
-
Set One Array Equal to Another in Java Using
Array.clone
- Conclusion
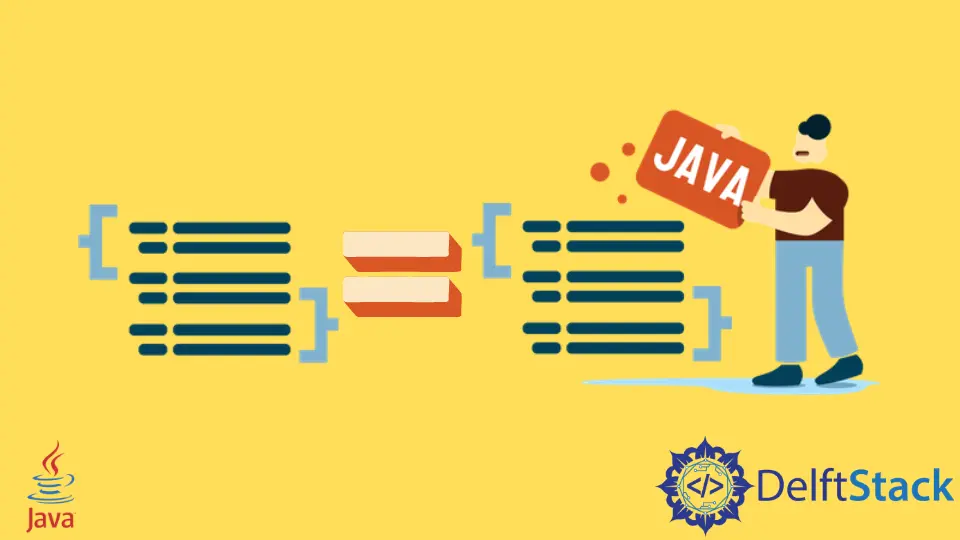
Setting one array equal to another is a common task in Java, a language where arrays are fundamental data structures. This operation is crucial in various scenarios, including data manipulation and algorithmic implementations. Understanding how to copy arrays effectively is essential for Java programmers.
This article delves into multiple methods to copy arrays in Java, providing insights into the techniques that best suit different programming contexts. Whether you’re a beginner or an experienced developer, these strategies will enhance your efficiency and accuracy in handling array-related tasks in Java.
Set One Array Equal to Another in Java Using a Loop
One straightforward method to set one array equal to another involves the use of a loop. This approach entails iterating through each element of the source array and copying its values to the corresponding positions in the destination array.
Consider the following example code:
public class ArrayCopyExample {
public static void main(String[] args) {
int[] sourceArray = {1, 2, 3, 4, 5};
int[] destinationArray = new int[sourceArray.length];
for (int i = 0; i < sourceArray.length; i++) {
destinationArray[i] = sourceArray[i];
}
System.out.print("Destination Array: ");
for (int element : destinationArray) {
System.out.print(element + " ");
}
}
}
Output:
Destination Array: 1 2 3 4 5
Here, we have a source array (sourceArray
) that contains the elements we want to copy. To store these elements, we initialize a destination array (destinationArray
) with the same length as the source array.
We start by initializing a loop variable, i
, to 0
. This variable will act as our index for both the source and destination arrays.
The loop continues as long as i
is less than the length of the source array (sourceArray.length
). This ensures that we iterate over every element in the source array.
Inside the loop, the line destinationArray[i] = sourceArray[i]
performs the actual copy operation. With each iteration, the element at index i
in the source array is copied to the corresponding position in the destination array.
After copying an element, the loop increments i
, ensuring that we move on to the next set of elements in both arrays. This process repeats until we’ve copied all elements from the source to the destination array.
Once the loop completes, the destinationArray
holds an identical set of elements as the sourceArray
.
Use the Enhanced for
Loop
The enhanced for
loop simplifies iteration over arrays and collections by handling the iteration details behind the scenes. In the context of array copying, the enhanced for
loop provides a concise and readable alternative to the conventional for
loop.
Now, let’s introduce the enhanced for
loop:
public class ArrayCopyExample {
public static void main(String[] args) {
int[] sourceArray = {1, 2, 3, 4, 5};
int[] destinationArray = new int[sourceArray.length];
int i = 0;
for (int value : sourceArray) {
destinationArray[i++] = value;
}
System.out.print("Destination Array: ");
for (int element : destinationArray) {
System.out.print(element + " ");
}
}
}
Output:
Destination Array: 1 2 3 4 5
First, we initialize i
to 0
outside the loop. This variable will act as our index for the destination array.
The enhanced for
loop is structured as for (int value : sourceArray)
. This loop automatically iterates through each element in sourceArray
, and in each iteration, the current element is assigned to the variable value
.
Inside the loop, destinationArray[i++] = value;
copies the current element (value
) to the corresponding position in the destination array. The i++
ensures that we move to the next position in the destination array with each iteration.
As we can see, the enhanced for
loop achieves the same result as the conventional for
loop: the destinationArray
now holds an identical set of elements as the sourceArray
.
Set One Array Equal to Another in Java Using System.arraycopy
The System.arraycopy
method also allows you to efficiently copy elements from one array to another. Below is the syntax of System.arraycopy
:
System.arraycopy(src, srcPos, dest, destPos, length);
Parameters:
src
: The source array from which elements will be copied.srcPos
: The starting position in the source array.dest
: The destination array where elements will be copied.destPos
: The starting position in the destination array.length
: The number of elements to be copied.
Consider the following example, where we have a source array, sourceArray
, and we want to copy its elements to a destination array, destinationArray
:
public class ArrayCopyExample {
public static void main(String[] args) {
int[] sourceArray = {5, 6, 7, 8, 9};
int[] destinationArray = new int[sourceArray.length];
System.arraycopy(sourceArray, 0, destinationArray, 0, sourceArray.length);
System.out.print("Destination Array: ");
for (int element : destinationArray) {
System.out.print(element + " ");
}
}
}
Output:
Destination Array: 5 6 7 8 9
In this example, we begin by creating a source array, sourceArray
, containing the elements we want to copy. A destination array, destinationArray
, is then initialized with the same length as the source array to accommodate the copied elements.
The crucial line here is System.arraycopy(sourceArray, 0, destinationArray, 0, sourceArray.length);
, which invokes the System.arraycopy
method.
In this line, sourceArray
is specified as the source array, 0
indicates the starting position in the source array, destinationArray
is the destination array, 0
is the starting position in the destination array, and sourceArray.length
determines the number of elements to copy.
System.arraycopy
performs a low-level memory block copy, efficiently transferring a contiguous block of memory from the source to the destination. This method is significantly faster than conventional loop-based approaches, especially for large arrays.
Upon completion of the method call, destinationArray
holds an identical set of elements as sourceArray
.
Set One Array Equal to Another in Java Using Arrays.copyOf
The Arrays.copyOf
method can also be used to create a new array with the same elements as the original array.
Its syntax is as follows:
type[] Arrays.copyOf(type[] original, int newLength);
Parameters:
original
: The source array from which elements will be copied.newLength
: The new length of the copied array.
Consider the following example, where we have a source array, sourceArray
, and we want to copy its elements to a destination array, destinationArray
:
import java.util.Arrays;
public class ArrayCopyExample {
public static void main(String[] args) {
int[] sourceArray = {10, 20, 30, 40, 50};
int[] destinationArray = Arrays.copyOf(sourceArray, sourceArray.length);
System.out.print("Destination Array: ");
for (int element : destinationArray) {
System.out.print(element + " ");
}
}
}
Output:
Destination Array: 10 20 30 40 50
In this example, we start by declaring a source array, sourceArray
, containing the elements we wish to copy. To accommodate the copied elements, a destination array, destinationArray
, is initialized using Arrays.copyOf
.
The line int[] destinationArray = Arrays.copyOf(sourceArray, sourceArray.length);
invokes the Arrays.copyOf
method.
Here, sourceArray
is specified as the original array to copy from, and sourceArray.length
determines the new length of the copied array.
Behind the scenes, Arrays.copyOf
handles the array copying operation with elegance. It creates a new array with the specified length and copies the elements from the original array to the new one.
This results in a concise and readable one-liner for array copying.
After the method call, destinationArray
contains an identical set of elements as sourceArray
.
Set One Array Equal to Another in Java Using Arrays.copyOfRange
If you want to copy only a specific range of elements from the source array, you can use Arrays.copyOfRange
.
Here’s the syntax of Arrays.copyOfRange
:
type[] Arrays.copyOfRange(type[] original, int from, int to);
Parameters:
original
: The source array from which elements will be copied.from
: The starting index (inclusive) of the range to copy.to
: The ending index (exclusive) of the range to copy.
Consider the following example:
import java.util.Arrays;
public class ArrayCopyExample {
public static void main(String[] args) {
int[] sourceArray = {1, 2, 3, 4, 5};
int[] destinationArray = Arrays.copyOfRange(sourceArray, 1, 4);
System.out.print("Destination Array: ");
for (int element : destinationArray) {
System.out.print(element + " ");
}
}
}
Output:
Destination Array: 2 3 4
Here, we start by declaring a source array, sourceArray
, containing the elements we wish to copy. To accommodate the copied elements within a specific range, a destination array, destinationArray
, is initialized using Arrays.copyOfRange
.
The specific line int[] destinationArray = Arrays.copyOfRange(sourceArray, 1, 4);
invokes the Arrays.copyOfRange
method.
The sourceArray
is specified as the original array to copy from. The from
parameter is set to 1
, indicating the starting index (inclusive) of the range, and to
is set to 4
, indicating the ending index (exclusive).
Arrays.copyOfRange
performs a precise array copying operation, creating a new array that includes elements from the specified range of the original array. It allows for flexibility in choosing the subset of elements to copy, making it particularly useful when dealing with partial arrays.
After the method call, destinationArray
contains the elements {2, 3, 4}
, which represent the specified range of elements from sourceArray
.
Set One Array Equal to Another in Java Using Array.clone
The clone
method of the Arrays
class can also be used to create a copy of the array.
Below is the syntax of Arrays.clone
:
type[] Array.clone();
Array
: The array to be cloned.
Consider the following example, where we have a source array, sourceArray
, and we want to create an identical copy in the destination array, destinationArray
:
import java.util.Arrays;
public class ArrayCopyExample {
public static void main(String[] args) {
int[] sourceArray = {1, 2, 3, 4, 5};
int[] destinationArray = sourceArray.clone();
System.out.print("Destination Array: ");
for (int element : destinationArray) {
System.out.print(element + " ");
}
}
}
Output:
Destination Array: 1 2 3 4 5
Here, we begin by declaring a source array, sourceArray
, containing the elements we wish to copy. To create a copy of this array, a destination array, destinationArray
, is initialized using sourceArray.clone()
.
The line int[] destinationArray = sourceArray.clone();
invokes the Array.clone
method. Here, sourceArray
is specified as the array to be cloned.
Internally, Array.clone
performs a shallow copy of the array. It creates a new array with the same length as the original and copies the elements. This method is efficient and concise, making it particularly useful for scenarios where a simple, identical copy is needed.
After the method call, destinationArray
contains an identical set of elements as sourceArray
.
Conclusion
Java offers a variety of methods for copying arrays, each with its advantages based on simplicity and efficiency requirements. Choosing the appropriate method depends on the specific use case, considering factors such as array size, performance considerations, and desired coding style.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook