How to Print Quotation Marks in Java
- Print Double Quotes Using Escape Sequence in Java
-
Print Double Quotes Using
char
in Java - Print Double Quotes Using Unicode Characters in Java
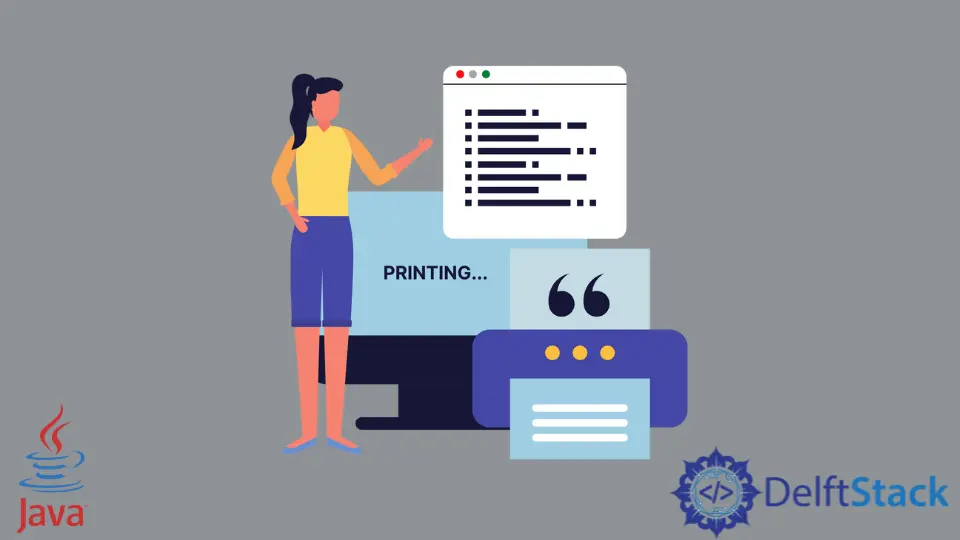
Double quotes in Java plays a vital role as they are mainly used to indicate a string. When we print any string, the double quotes are not printed but only the value inside them is printed. But how to print quotation marks in Java?
In the examples below, we will learn the various methods that we can use to print the double quotes together with the string.
Print Double Quotes Using Escape Sequence in Java
The first method to print the double quotes with the string uses an escape sequence, which is a backslash ( \
) with a character. It is sometimes also called an escape character. Our goal is to insert double quotes at the starting and the ending point of ourString
.
\"
is the escape sequence that is used to insert a double quote. Below we can see that we are using this escape sequence inside ourString
, and the output shows the string with quotations.
public class PrintQuotes {
public static void main(String[] args) {
String ourString = " \"This is a string\" ";
System.out.println(ourString);
}
}
Output:
"This is a string"
Print Double Quotes Using char
in Java
We can also use char
to print the double quotes with the string. First, we have to convert the double quote ( "
) into a char
. In the below example, we have singleQuotesChar
with a double quote surrounded by single quotes. The double-quote represents a string, and the single quote represents a char
.
Now, as our double quote has become a char
, we can concatenate it with the string at both the starting and ending points.
public class PrintQuotes {
public static void main(String[] args) {
char singleQuotesChar = '"';
String ourString = singleQuotesChar + "This is a string" + singleQuotesChar;
System.out.println(ourString);
}
}
Output:
"This is a string"
Print Double Quotes Using Unicode Characters in Java
In this example, we will use Unicode characters to print Java quotes in a string. Whenever we want to print or use any character like symbols or non-English characters, we can use Unicode characters. Every Unicode represents a character, and \u0022
means a double quote.
We need to convert the Unicode to a char
and then concatenate \u0022
with the string.
public class PrintQuotes {
public static void main(String[] args) {
String ourString = '\u0022' + "This is a String" + '\u0022';
System.out.println(ourString);
}
}
Output:
"This is a String"
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn