How to Print an Array in Java
-
Use the
for
Loop to Print an Array in Java -
Use
toString()
Method to Print an Array in Java -
Use the
stream().forEach()
Method to Print an Array in Java -
Use the
asList()
Method to Print Multidimensional Array in Java -
Use the
deepToString()
Method to Print Multidimensional Array in Java
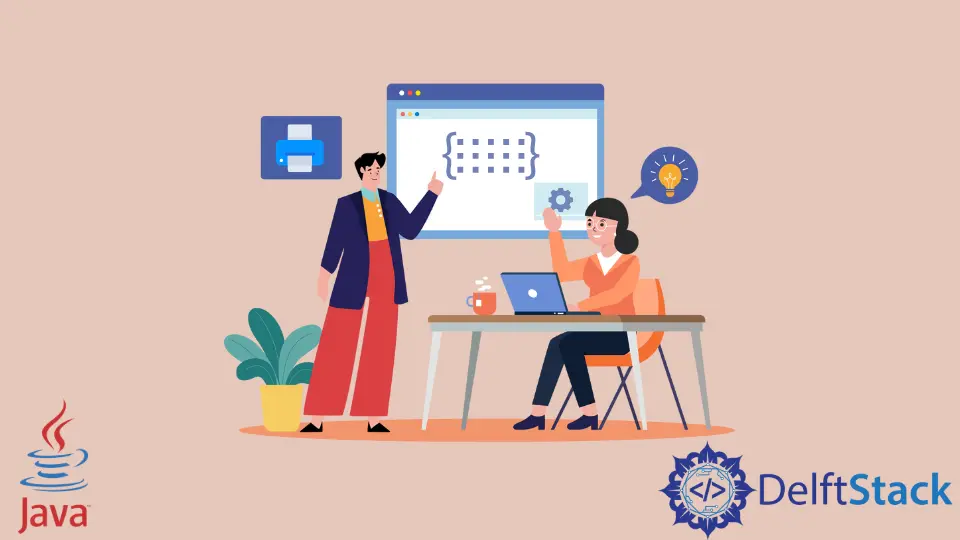
This tutorial article will introduce how to print an array in Java. There are two main ways to print an array in Java, the for
loop, and Java built-in methods.
Use the for
Loop to Print an Array in Java
We can use the for
loop to print the array in Java. With every iteration in the for
loop, we print elements of an array in Java.
The example code of printing an array in Java using the for
loop is as follows.
public class PrintingAnArray {
public static void main(String args[]) {
int Array[] = {1, 2, 3, 4, 5};
for (int i = 0; i < Array.length; i++) {
System.out.println(Array[i]);
}
}
}
Output:
1
2
3
4
5
Use toString()
Method to Print an Array in Java
The toString()
method is a static method of the Array
class in Java that belongs to the java.util
package. We need to import java.util.Arrays
in our code to use the toString()
method.
The toString()
method takes an array as an argument, converts/typecasts that array to the string, and returns that string. Each element of the integer array will be converted to a string.
The example code of printing an array in Java using the toString()
method is as follows.
import java.util.Arrays;
public class PrintingAnArray {
public static void main(String args[]) {
int Array[] = {1, 2, 3, 4, 5};
System.out.println(Arrays.toString(Array));
}
}
Output:
[1, 2, 3, 4, 5]
Use the stream().forEach()
Method to Print an Array in Java
We can use the stream().forEach()
method to print the elements of the array in Java. This method takes the array as an argument and then prints its elements iteratively but without using any explicit loop.
The example code of printing an array in Java using the stream().forEach()
method is as follows.
import java.util.Arrays;
public class PrintingAnArray {
public static void main(String args[]) {
int Array[] = {1, 2, 3, 4, 5};
Arrays.stream(Array).forEach(System.out::println);
}
}
Output:
[1, 2, 3, 4, 5]
Use the deepToString()
Method to Print Multidimensional Array in Java
We can also use deepToString()
to print the multidimensional array in Java. This method accepts the multidimensional array as an argument and converts that array into a string that would be printed directly.
The example code of printing a multidimensional array in Java using the deepToString
method is as follows.
import java.util.Arrays;
public class PrintingAnArray {
public static void main(String args[]) {
int Array[][] = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}};
System.out.println(Arrays.deepToString(Array));
}
}
Output:
[[1, 2, 3], [4, 5, 6], [7, 8, 9]]