How to Open a File in Java
-
Using the
Desktop
Class to Open a File in Java - Using FileInputStream Class in Java
-
Using the
BufferedReader
Class to Open a File in Java -
Using the
Scanner
Class to Open a File in Java
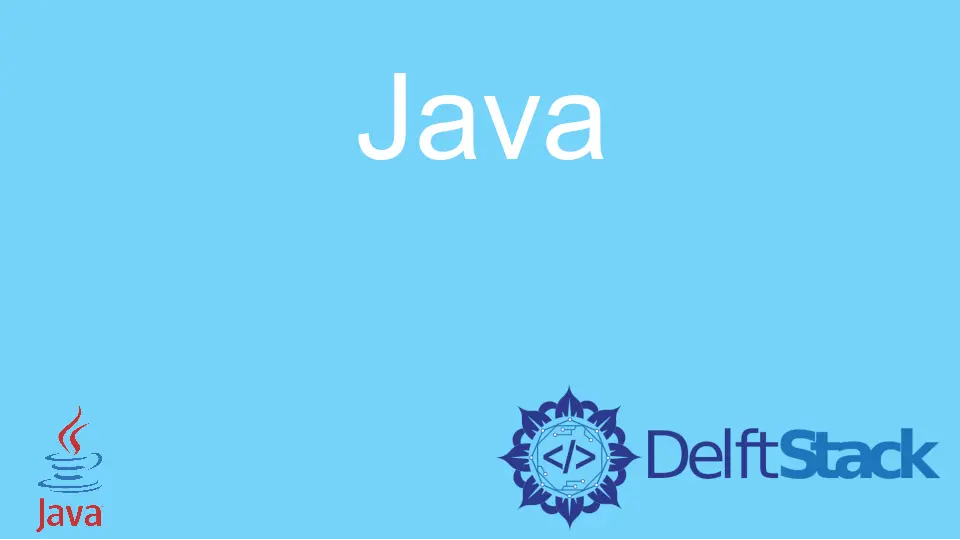
There are many ways to open a file in Java. We will discuss a few of them here in this article.
Using the Desktop
Class to Open a File in Java
The Java AWT package has a Java Desktop
class used to launch registered applications on the native desktop to handle a file or URI. Its implementation is platform-dependent; hence, it is necessary to check whether the operating system supports Desktop
or not. It throws FileNotFoundException
if there is no registered application or it fails to be launched.
The Desktop
class has the open()
method that launches the registered application to open a file which it takes as an argument.
We create a new File
class object passing the path to the text file as an argument. It returns immediately if the Desktop.isDesktopSupported()
returns false if the system does not support the Desktop. We get the Desktop
instance desktop
of the current browser context.
We use the method exists()
on the file
object to check if the file exists. We call desktop.open()
to open the file in the default text editor.
import java.awt.*;
import java.io.File;
public class OpenFile {
public static void main(String args[]) {
try {
File file = new File("/Users/john/Desktop/demo.txt");
if (!Desktop.isDesktopSupported()) {
System.out.println("not supported");
return;
}
Desktop desktop = Desktop.getDesktop();
if (file.exists())
desktop.open(file);
} catch (Exception e) {
e.printStackTrace();
}
}
}
Using FileInputStream Class in Java
The FileInputStream
class is used to read byte-oriented data such as audio, image data, video, etc. It can also be used to read streams of characters as it obtains input bytes from files. Hence it can be used to open and read files. The FileReader
class is recommended for reading files, though.
We create a FileInputStream fIS
to read from the file object, f
in our code. We read the contents of the file and writes it to the terminal. In the while
loop, we read the characters of the stream until the read()
method returns -1.
import java.io.File;
import java.io.FileInputStream;
public class OpenFile {
public static void main(String args[]) {
try {
File f = new File("/Users/john/Desktop/demo.txt");
FileInputStream fIS = new FileInputStream(f);
System.out.println("file content: ");
int r = 0;
while ((r = fIS.read()) != -1) {
System.out.print((char) r);
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
Output:
file content:
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Integer eget quam malesuada, molestie nibh id, vestibulum libero. Vestibulum sed ligula ut ligula bibendum pharetra. Suspendisse est odio, euismod vel porta ac, dapibus at lorem. In a leo vestibulum, commodo erat eget, cursus purus. Integer hendrerit orci eu erat pretium hendrerit. Etiam dignissim tempus orci. Etiam suscipit non sapien vitae condimentum. Fusce nec molestie nulla. Cras ut vestibulum tortor. Duis velit mi, dapibus vel scelerisque vitae, sagittis vitae purus. Ut et tempus eros, id scelerisque dolor. In suscipit ante sem.
Using the BufferedReader
Class to Open a File in Java
The BufferedReader class reads text from a character-based input stream. It takes a reader object that so we pass a new FileReader
to its constructor. FileReader
is a convenient class to read character files.
BufferedReader creates a buffering character-input stream that uses a default size. We read from the stream until the read()
method returns -1 and prints the output of the file.
import java.io.BufferedReader;
import java.io.File;
import java.io.FileReader;
public class OpenFile {
public static void main(String args[]) {
try {
File fil = new File("/Users/john/Desktop/demo2.txt");
BufferedReader br = new BufferedReader(new FileReader(fil));
System.out.println("file content: ");
int r = 0;
while ((r = br.read()) != -1) {
System.out.print((char) r);
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
Output:
file content:--
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Integer eget quam malesuada, molestie nibh id, vestibulum libero. Vestibulum sed ligula ut ligula bibendum pharetra. Suspendisse est odio, euismod vel porta ac, dapibus at lorem. In a leo vestibulum, commodo erat eget, cursus purus. Integer hendrerit orci eu erat pretium hendrerit. Etiam dignissim tempus orci. Etiam suscipit non sapien vitae condimentum. Fusce nec molestie nulla. Cras ut vestibulum tortor. Duis velit mi, dapibus vel scelerisque vitae, sagittis vitae purus. Ut et tempus eros, id scelerisque dolor. In suscipit ante sem.
Using the Scanner
Class to Open a File in Java
The Scanner
class in Java belongs to the java.util
package. It can parse primitive types and strings using regular expressions and can be used to read data from files. We create a Scanner
class object by specifying the file in the constructor.
We read the file line by line using the hasNextLine()
method and print each line inside the while
loop until we have a next line to read from the file.
import java.io.File;
import java.util.Scanner;
public class OpenFile {
public static void main(String args[]) {
try {
File file = new File("/Users/john/Desktop/demo1.txt");
Scanner scanner = new Scanner(file);
while (scanner.hasNextLine()) System.out.println(scanner.nextLine());
} catch (Exception e) {
e.printStackTrace();
}
}
}
Output:
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Integer eget quam malesuada, molestie nibh id, vestibulum libero. Vestibulum sed ligula ut ligula bibendum pharetra. Suspendisse est odio, euismod vel porta ac, dapibus at lorem. In a leo vestibulum, commodo erat eget, cursus purus. Integer hendrerit orci eu erat pretium hendrerit. Etiam dignissim tempus orci. Etiam suscipit non sapien vitae condimentum. Fusce nec molestie nulla. Cras ut vestibulum tortor. Duis velit mi, dapibus vel scelerisque vitae, sagittis vitae purus. Ut et tempus eros, id scelerisque dolor. In suscipit ante sem.
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn