How to Fix Error: Not on FX Application Thread in Java
- Understanding the Error
- Method 1: Using Platform.runLater
- Method 2: Using Task and Service
- Method 3: Use of Event Dispatch Thread
- Conclusion
- FAQ
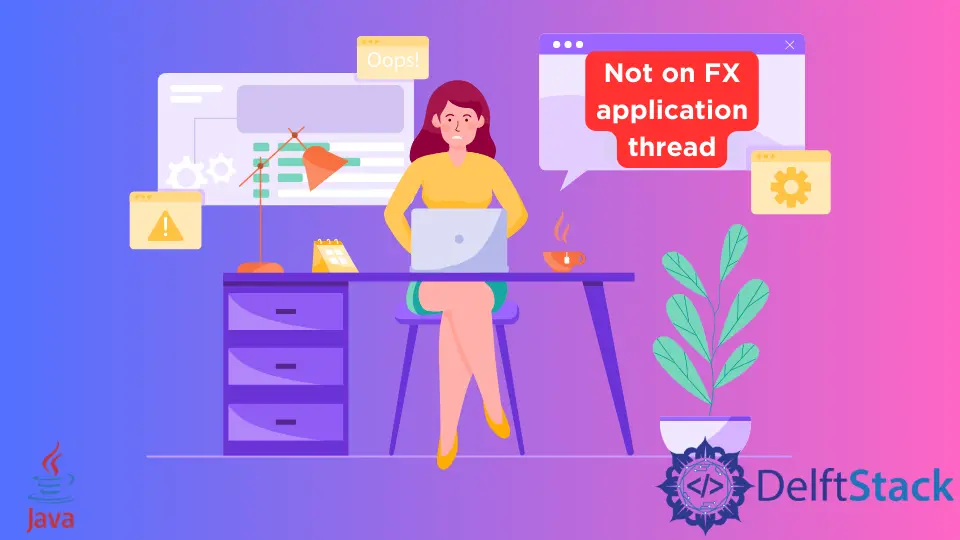
Java developers often encounter the “Not on FX Application Thread” error when working with JavaFX applications. This error arises when you attempt to update the user interface (UI) from a non-JavaFX thread, leading to unexpected behavior and crashes.
In this tutorial, we will explore the root causes of this error and provide effective solutions to fix it. Whether you’re a seasoned developer or just starting with JavaFX, understanding how to handle this error is crucial for creating responsive and stable applications. Let’s dive into the methods that can help you resolve this issue and ensure your JavaFX applications run smoothly.
Understanding the Error
Before we get into the solutions, it’s essential to understand why this error occurs. JavaFX is designed to manage the UI on a single thread, known as the JavaFX Application Thread. When any code that modifies the UI is executed on a different thread, JavaFX throws the “Not on FX Application Thread” error to prevent potential inconsistencies. This is a protective measure to maintain the integrity of the UI and ensure a smooth user experience.
Now, let’s look at some effective methods to fix this error.
Method 1: Using Platform.runLater
One of the most straightforward ways to resolve the “Not on FX Application Thread” error is by using the Platform.runLater
method. This method allows you to run a piece of code on the JavaFX Application Thread, ensuring that any UI updates are handled correctly.
Here’s how you can implement it:
import javafx.application.Platform;
public class Example {
public void updateUI() {
// Simulate a background task
new Thread(() -> {
// This will cause the error
// label.setText("Updated Text");
// Correct approach
Platform.runLater(() -> {
label.setText("Updated Text");
});
}).start();
}
}
In this code snippet, we simulate a background task using a new thread. If we attempt to update the label directly from this thread, it will throw the “Not on FX Application Thread” error. Instead, by wrapping the UI update inside Platform.runLater
, we ensure that the update occurs on the JavaFX Application Thread.
Output:
No error, label updated successfully
By using Platform.runLater
, you can effectively update UI components from any background thread without encountering threading issues. This method is particularly useful when performing long-running tasks that need to update the UI upon completion.
Method 2: Using Task and Service
Another powerful approach to handle background tasks in JavaFX is by using the Task
and Service
classes. These classes are designed to handle background operations while providing built-in support for updating the UI safely.
Here’s an example of how to use a Task
to perform a background operation:
import javafx.concurrent.Task;
public class Example {
public void performTask() {
Task<Void> task = new Task<Void>() {
@Override
protected Void call() throws Exception {
// Simulate a long-running task
Thread.sleep(2000);
// Update UI safely
updateMessage("Task Complete");
return null;
}
};
task.setOnSucceeded(event -> {
label.setText(task.getMessage());
});
new Thread(task).start();
}
}
In this example, we create a Task
that simulates a long-running operation by sleeping for two seconds. Once the operation is complete, we safely update the UI by using the updateMessage
method. The setOnSucceeded
method is then used to define what happens when the task completes successfully.
Output:
Task Complete
Using Task
and Service
not only helps in managing background operations but also provides a structured way to handle UI updates, making your code cleaner and more maintainable.
Method 3: Use of Event Dispatch Thread
In JavaFX, the concept of the Event Dispatch Thread (EDT) is crucial. The EDT is responsible for handling all the UI events. If you’re working with JavaFX, you should ensure that any UI changes are executed on this thread. Here’s how to implement this:
import javafx.application.Application;
import javafx.application.Platform;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.Label;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class Example extends Application {
private Label label;
@Override
public void start(Stage primaryStage) {
label = new Label("Initial Text");
Button button = new Button("Update Text");
button.setOnAction(e -> updateLabel());
VBox vbox = new VBox(label, button);
Scene scene = new Scene(vbox, 300, 200);
primaryStage.setScene(scene);
primaryStage.show();
}
private void updateLabel() {
new Thread(() -> {
// This will cause an error
// label.setText("Updated Text");
// Correct approach
Platform.runLater(() -> label.setText("Updated Text"));
}).start();
}
public static void main(String[] args) {
launch(args);
}
}
In this example, we create a simple JavaFX application with a button that updates a label. If we try to update the label directly from a background thread, we will encounter the error. Instead, we use Platform.runLater
to ensure the UI update happens on the EDT.
Output:
Updated Text
This method reinforces the importance of always running UI updates on the correct thread, thereby preventing the “Not on FX Application Thread” error.
Conclusion
The “Not on FX Application Thread” error can be a significant hurdle for JavaFX developers, but understanding its cause and knowing how to fix it can make your development experience much smoother. By using methods like Platform.runLater
, Task
, and ensuring that all UI updates occur on the Event Dispatch Thread, you can create responsive and stable JavaFX applications. Remember, maintaining thread safety is crucial for any UI application, and with these techniques, you can avoid common pitfalls.
FAQ
-
what is the FX application thread?
The FX application thread is the thread responsible for managing the JavaFX user interface. All UI updates must occur on this thread to ensure thread safety. -
how can I safely update UI components in JavaFX?
You can safely update UI components in JavaFX by using the Platform.runLater method or by utilizing the Task and Service classes for background operations. -
what happens if I update the UI from a non-JavaFX thread?
If you update the UI from a non-JavaFX thread, you will encounter the “Not on FX Application Thread” error, which can lead to application crashes and unexpected behavior. -
can I use JavaFX with other Java libraries?
Yes, you can use JavaFX with other Java libraries, but you must ensure that any UI updates are performed on the JavaFX Application Thread to avoid threading issues. -
how do I handle long-running tasks in JavaFX?
You can handle long-running tasks in JavaFX by using the Task and Service classes, which allow you to perform background operations while safely updating the UI.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn FacebookRelated Article - Java Thread
- Overview of ThreadLocal in Java
- Daemon Thread in Java
- How to Get Thread Id in Java
- How to Kill Thread in Java
Related Article - Java Error
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix the Missing Server JVM Error in Java
- How to Fix the 'No Java Virtual Machine Was Found' Error in Eclipse
- How to Fix Javax.Net.SSL.SSLHandShakeException: Remote Host Closed Connection During Handshake
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix Java.Lang.VerifyError: Bad Type on Operand Stack