Overview of ThreadLocal in Java
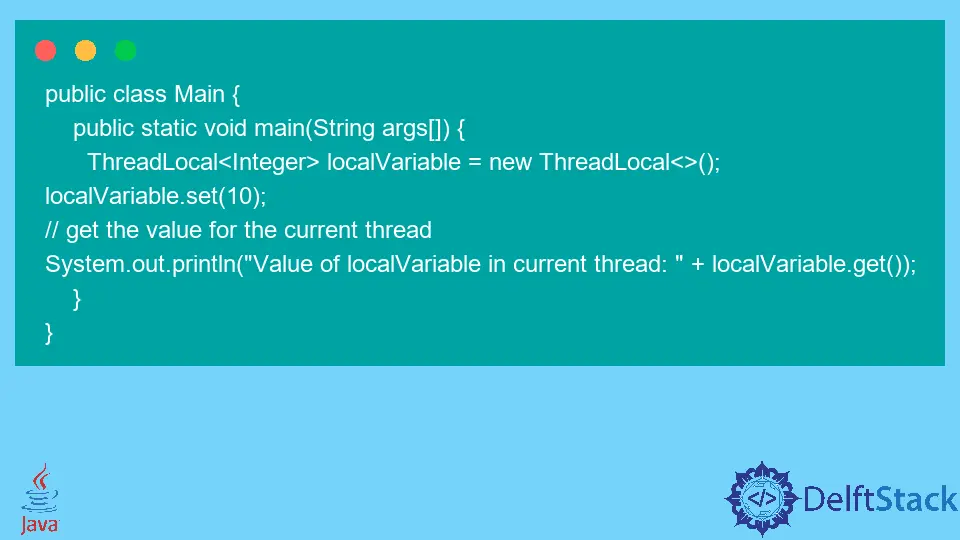
ThreadLocal
is a powerful tool in the Java language that allows developers to create variables specific to each thread of execution. This means that each thread has its copy of the variable, independent of the values of the same variable in other threads.
This is particularly useful in multi-threaded applications, where different threads may have different requirements for a given variable and where changes to the value of a variable in one thread shouldn’t affect the value of the same variable in another thread.
Main Use of ThreadLocal
Variable
The main use case for ThreadLocal
is to store a per-thread state, such as a user ID or transaction ID, that is specific to a single request and should not be shared between different requests.
For example, consider a web application that uses a single database connection to handle multiple user requests. Without ThreadLocal
, it would be difficult to ensure that each request uses the correct user information, as multiple requests could be executed simultaneously by different threads and interfere with each other.
With ThreadLocal
, each thread can store its user information, which will be used by the database connection associated with that thread.
to Use a ThreadLocal
in Java
To use ThreadLocal
in Java, you declare a new instance of the ThreadLocal
class and then use its get()
and set()
methods to access and modify the value associated with the current thread.
For example, the following code demonstrates using a ThreadLocal
to store a user ID for each thread.
public class Main {
public static void main(String args[]) {
ThreadLocal<Integer> localVariable = new ThreadLocal<>();
localVariable.set(10);
// get the value for the current thread
System.out.println("Value of localVariable in current thread: " + localVariable.get());
}
}