Daemon Thread in Java
-
Create Daemon Thread Using
setDaemon()
Method in Java -
Create Daemon Thread Using the
setDaemon()
Method in Java -
Create Daemon Thread Using the
setDaemon()
Method in Java
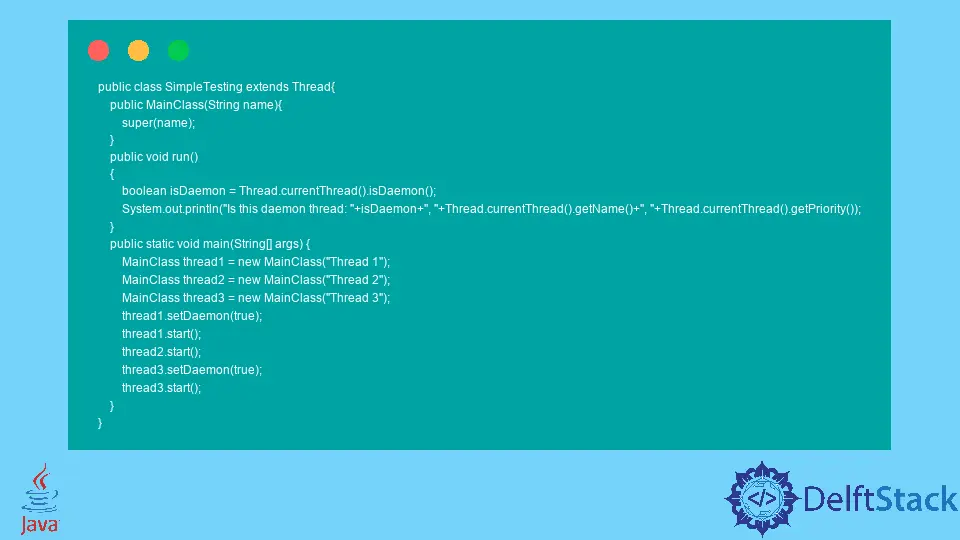
This tutorial will introduce what daemon thread is and how to create a daemon thread in Java.
In Java, a daemon thread is a special thread supporting background thread for other threads. Java uses these threads to serve special purposes for user threads and garbage collection, etc.
To create a daemon thread, Java provides the setDaemon()
method that takes a boolean argument. The isDaemon()
method can check whether the current running thread is a Daemon thread or not.
Create Daemon Thread Using setDaemon()
Method in Java
In this example, we use the setDaemon()
method of the Thread
class to create a daemon thread. We create three threads, and two of them are set as daemon threads. We use the isDaemon()
method to check whether the current running thread is a daemon thread or not. See the example below.
public class SimpleTesting extends Thread {
public MainClass(String name) {
super(name);
}
public void run() {
boolean isDaemon = Thread.currentThread().isDaemon();
System.out.println("Is this daemon thread: " + isDaemon);
}
public static void main(String[] args) {
MainClass thread1 = new MainClass("Thread 1");
MainClass thread2 = new MainClass("Thread 2");
MainClass thread3 = new MainClass("Thread 3");
thread1.setDaemon(true);
thread1.start();
thread2.start();
thread3.setDaemon(true);
thread3.start();
}
}
Output:
Is this daemon thread: true
Is this daemon thread: false
Is this daemon thread: true
Create Daemon Thread Using the setDaemon()
Method in Java
The daemon thread is the low priority thread and always runs behind the user threads. We can see a thread’s priority by using the getPriority()
method that returns an integer value. We use the getName()
method to fetch the name of the current running thread. See the below example.
public class SimpleTesting extends Thread {
public MainClass(String name) {
super(name);
}
public void run() {
boolean isDaemon = Thread.currentThread().isDaemon();
System.out.println("Is this daemon thread: " + isDaemon + ", "
+ Thread.currentThread().getName() + ", " + Thread.currentThread().getPriority());
}
public static void main(String[] args) {
MainClass thread1 = new MainClass("Thread 1");
MainClass thread2 = new MainClass("Thread 2");
MainClass thread3 = new MainClass("Thread 3");
thread1.setDaemon(true);
thread1.start();
thread2.start();
thread3.setDaemon(true);
thread3.start();
}
}
Output:
Is this daemon thread: true, Thread 1, 5
Is this daemon thread: false, Thread 2, 5
Is this daemon thread: true, Thread 3, 5
Create Daemon Thread Using the setDaemon()
Method in Java
We start the thread after setting a value to the setDaemon()
method while creating a daemon thread. It is essential because if we set an already running thread as a daemon, it will throw an exception. We call the start()
method with thread1
and then call setDaemon()
, which is not allowed. See the below example.
public class SimpleTesting extends Thread {
public MainClass(String name) {
super(name);
}
public void run() {
boolean isDaemon = Thread.currentThread().isDaemon();
System.out.println("Is this daemon thread: " + isDaemon + ", "
+ Thread.currentThread().getName() + ", " + Thread.currentThread().getPriority());
}
public static void main(String[] args) {
MainClass thread1 = new MainClass("Thread 1");
MainClass thread2 = new MainClass("Thread 2");
MainClass thread3 = new MainClass("Thread 3");
thread1.start();
thread1.setDaemon(true);
thread2.start();
thread3.setDaemon(true);
thread3.start();
}
}
Output:
Is this daemon thread: false, Thread 1, 5
Exception in thread "main" java.lang.IllegalThreadStateException