Java의 데몬 스레드
-
Java에서
setDaemon()
메소드를 사용하여 데몬 스레드 생성 -
Java에서
setDaemon()
메소드를 사용하여 데몬 스레드 생성 -
Java에서
setDaemon()
메소드를 사용하여 데몬 스레드 생성
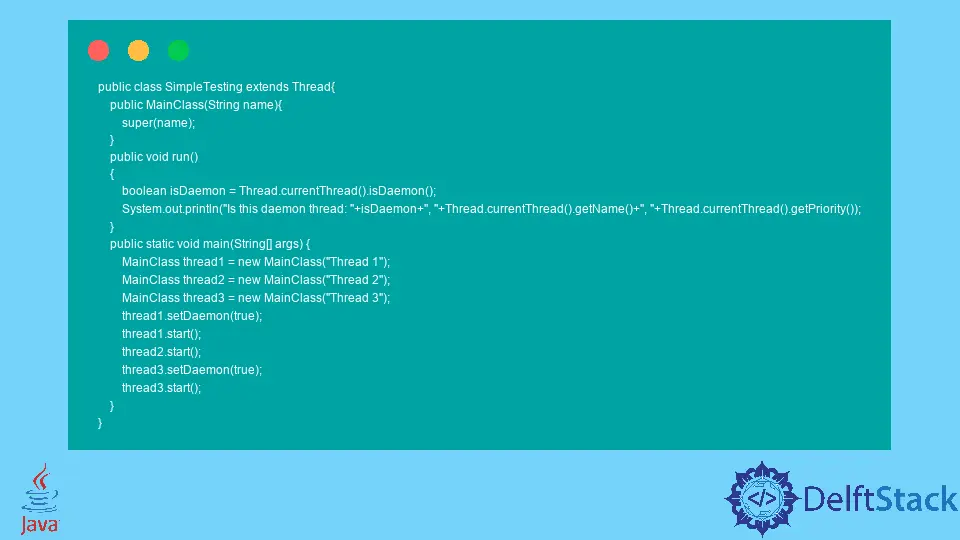
이 튜토리얼은 데몬 스레드가 무엇이며 Java에서 데몬 스레드를 만드는 방법을 소개합니다.
Java에서 데몬 스레드는 다른 스레드에 대한 백그라운드 스레드를 지원하는 특수 스레드입니다. Java는 이러한 스레드를 사용하여 사용자 스레드 및 가비지 수집 등에 대한 특수 목적을 제공합니다.
데몬 스레드를 생성하기 위해 Java는 부울 인수를 사용하는setDaemon()
메소드를 제공합니다. isDaemon()
메소드는 현재 실행중인 스레드가 데몬 스레드인지 여부를 확인할 수 있습니다.
Java에서setDaemon()
메소드를 사용하여 데몬 스레드 생성
이 예에서는Thread
클래스의setDaemon()
메소드를 사용하여 데몬 스레드를 생성합니다. 세 개의 스레드를 만들고 그중 두 개는 데몬 스레드로 설정됩니다. isDaemon()
메소드를 사용하여 현재 실행중인 스레드가 데몬 스레드인지 여부를 확인합니다. 아래 예를 참조하십시오.
public class SimpleTesting extends Thread {
public MainClass(String name) {
super(name);
}
public void run() {
boolean isDaemon = Thread.currentThread().isDaemon();
System.out.println("Is this daemon thread: " + isDaemon);
}
public static void main(String[] args) {
MainClass thread1 = new MainClass("Thread 1");
MainClass thread2 = new MainClass("Thread 2");
MainClass thread3 = new MainClass("Thread 3");
thread1.setDaemon(true);
thread1.start();
thread2.start();
thread3.setDaemon(true);
thread3.start();
}
}
출력:
Is this daemon thread: true
Is this daemon thread: false
Is this daemon thread: true
Java에서setDaemon()
메소드를 사용하여 데몬 스레드 생성
데몬 스레드는 우선 순위가 낮은 스레드이며 항상 사용자 스레드 뒤에서 실행됩니다. 정수 값을 반환하는getPriority()
메서드를 사용하여 스레드의 우선 순위를 확인할 수 있습니다. getName()
메소드를 사용하여 현재 실행중인 스레드의 이름을 가져옵니다. 아래 예를 참조하십시오.
public class SimpleTesting extends Thread {
public MainClass(String name) {
super(name);
}
public void run() {
boolean isDaemon = Thread.currentThread().isDaemon();
System.out.println("Is this daemon thread: " + isDaemon + ", "
+ Thread.currentThread().getName() + ", " + Thread.currentThread().getPriority());
}
public static void main(String[] args) {
MainClass thread1 = new MainClass("Thread 1");
MainClass thread2 = new MainClass("Thread 2");
MainClass thread3 = new MainClass("Thread 3");
thread1.setDaemon(true);
thread1.start();
thread2.start();
thread3.setDaemon(true);
thread3.start();
}
}
출력:
Is this daemon thread: true, Thread 1, 5
Is this daemon thread: false, Thread 2, 5
Is this daemon thread: true, Thread 3, 5
Java에서setDaemon()
메소드를 사용하여 데몬 스레드 생성
데몬 스레드를 생성하는 동안setDaemon()
메소드에 값을 설정 한 후 스레드를 시작합니다. 이미 실행중인 스레드를 데몬으로 설정하면 예외가 발생하기 때문에 필수적입니다. thread1
으로start()
메소드를 호출 한 다음 허용되지 않는setDaemon()
을 호출합니다. 아래 예를 참조하십시오.
public class SimpleTesting extends Thread {
public MainClass(String name) {
super(name);
}
public void run() {
boolean isDaemon = Thread.currentThread().isDaemon();
System.out.println("Is this daemon thread: " + isDaemon + ", "
+ Thread.currentThread().getName() + ", " + Thread.currentThread().getPriority());
}
public static void main(String[] args) {
MainClass thread1 = new MainClass("Thread 1");
MainClass thread2 = new MainClass("Thread 2");
MainClass thread3 = new MainClass("Thread 3");
thread1.start();
thread1.setDaemon(true);
thread2.start();
thread3.setDaemon(true);
thread3.start();
}
}
출력:
Is this daemon thread: false, Thread 1, 5
Exception in thread "main" java.lang.IllegalThreadStateException