Java のデーモンスレッド
-
Java で
setDaemon()
メソッドを使用してデーモンスレッドを作成する -
Java で
setDaemon()
メソッドを使用してデーモンスレッドを作成する -
Java で
setDaemon()
メソッドを使用してデーモンスレッドを作成する
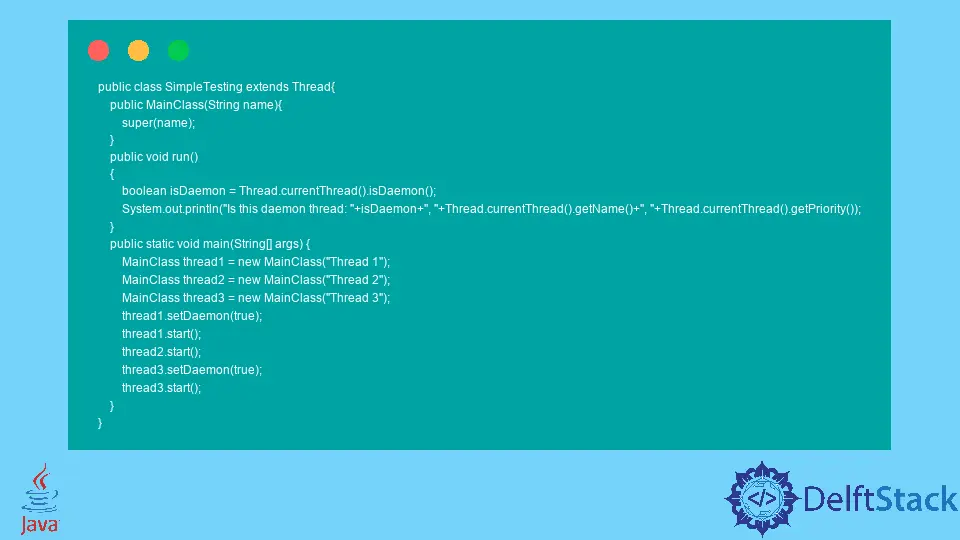
このチュートリアルでは、デーモンスレッドとは何か、および Java でデーモンスレッドを作成する方法を紹介します。
Java では、デーモンスレッドは、他のスレッドのバックグラウンドスレッドをサポートする特別なスレッドです。Java はこれらのスレッドを使用して、ユーザースレッドやガベージコレクションなどの特別な目的を果たします。
デーモンスレッドを作成するために、Java はブール引数を取る setDaemon()
メソッドを提供します。isDaemon()
メソッドは、現在実行中のスレッドがデーモンスレッドであるかどうかを確認できます。
Java で setDaemon()
メソッドを使用してデーモンスレッドを作成する
この例では、Thread
クラスの setDaemon()
メソッドを使用してデーモンスレッドを作成します。3つのスレッドを作成し、そのうちの 2つをデーモンスレッドとして設定します。isDaemon()
メソッドを使用して、現在実行中のスレッドがデーモンスレッドであるかどうかを確認します。以下の例を参照してください。
public class SimpleTesting extends Thread {
public MainClass(String name) {
super(name);
}
public void run() {
boolean isDaemon = Thread.currentThread().isDaemon();
System.out.println("Is this daemon thread: " + isDaemon);
}
public static void main(String[] args) {
MainClass thread1 = new MainClass("Thread 1");
MainClass thread2 = new MainClass("Thread 2");
MainClass thread3 = new MainClass("Thread 3");
thread1.setDaemon(true);
thread1.start();
thread2.start();
thread3.setDaemon(true);
thread3.start();
}
}
出力:
Is this daemon thread: true
Is this daemon thread: false
Is this daemon thread: true
Java で setDaemon()
メソッドを使用してデーモンスレッドを作成する
デーモンスレッドは優先度の低いスレッドであり、常にユーザースレッドの背後で実行されます。整数値を返す getPriority()
メソッドを使用すると、スレッドの優先度を確認できます。getName()
メソッドを使用して、現在実行中のスレッドの名前をフェッチします。以下の例を参照してください。
public class SimpleTesting extends Thread {
public MainClass(String name) {
super(name);
}
public void run() {
boolean isDaemon = Thread.currentThread().isDaemon();
System.out.println("Is this daemon thread: " + isDaemon + ", "
+ Thread.currentThread().getName() + ", " + Thread.currentThread().getPriority());
}
public static void main(String[] args) {
MainClass thread1 = new MainClass("Thread 1");
MainClass thread2 = new MainClass("Thread 2");
MainClass thread3 = new MainClass("Thread 3");
thread1.setDaemon(true);
thread1.start();
thread2.start();
thread3.setDaemon(true);
thread3.start();
}
}
出力:
Is this daemon thread: true, Thread 1, 5
Is this daemon thread: false, Thread 2, 5
Is this daemon thread: true, Thread 3, 5
Java で setDaemon()
メソッドを使用してデーモンスレッドを作成する
デーモンスレッドの作成中に setDaemon()
メソッドに値を設定した後、スレッドを開始します。すでに実行中のスレッドをデーモンとして設定すると、例外がスローされるため、これは不可欠です。thread1
を使用して start()
メソッドを呼び出してから、setDaemon()
を呼び出しますが、これは許可されていません。以下の例を参照してください。
public class SimpleTesting extends Thread {
public MainClass(String name) {
super(name);
}
public void run() {
boolean isDaemon = Thread.currentThread().isDaemon();
System.out.println("Is this daemon thread: " + isDaemon + ", "
+ Thread.currentThread().getName() + ", " + Thread.currentThread().getPriority());
}
public static void main(String[] args) {
MainClass thread1 = new MainClass("Thread 1");
MainClass thread2 = new MainClass("Thread 2");
MainClass thread3 = new MainClass("Thread 3");
thread1.start();
thread1.setDaemon(true);
thread2.start();
thread3.setDaemon(true);
thread3.start();
}
}
出力:
Is this daemon thread: false, Thread 1, 5
Exception in thread "main" java.lang.IllegalThreadStateException