How to Kill Thread in Java
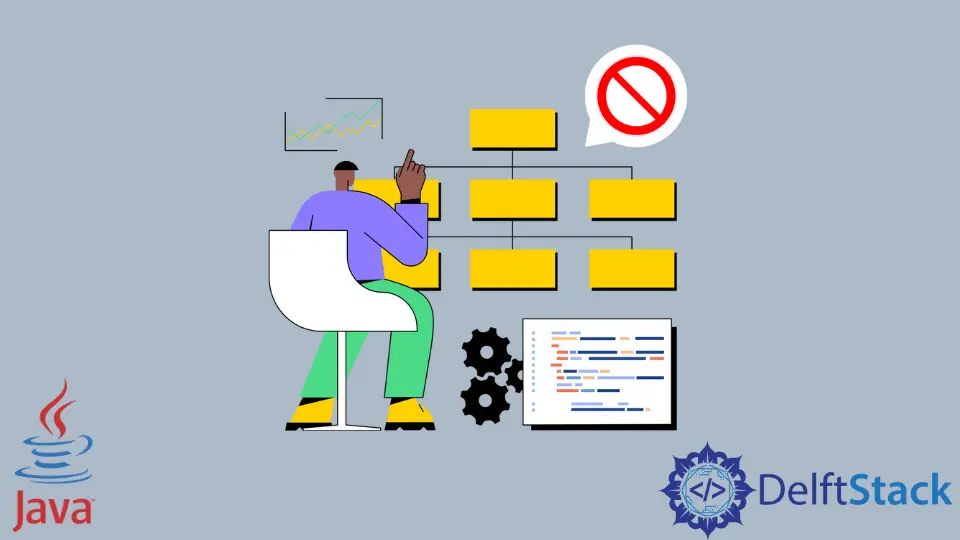
Threads in Java allow us to run several tasks in parallel, which enables multitasking. We can create a thread in Java using the Thread
class. In this article, we will introduce two methods to kill a thread.
Although the thread is destroyed by the run()
method of the Thread
class once it has completed all the tasks, sometimes we might want to kill or stop a thread before it has finished executing completely.
Kill or Stop a Thread Using a boolean
Flag in Java
To explicitly kill a thread, we can use a boolean flag to notify the thread when to stop the task. Below, two threads print a line with its name, and then both the threads sleep for about 100 milliseconds. The threads are executed until the boolean flag exitThread
becomes true.
thread1
and thread2
are two created threads, and a name is passed as an argument in both of them. As the constructor of the ExampleThread
class has thread.start()
that starts the execution of a thread, both the threads will execute. We can see that the output prints both the threads’ names randomly because they are being executed parallelly.
To stop the thread, we will call stopThread()
, which is a method in ExampleThread
that sets exitThread
to true
, and the thread finally stops because while(!exitThread)
becomes false
.
public class KillThread {
public static void main(String[] args) {
ExampleThread thread1 = new ExampleThread("Thread One");
ExampleThread thread2 = new ExampleThread("Thread Two");
try {
Thread.sleep(500);
thread1.stopThread();
thread2.stopThread();
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println("**Exiting the main Thread**");
}
}
class ExampleThread implements Runnable {
private String name;
private boolean exitThread;
Thread thread;
ExampleThread(String name) {
this.name = name;
thread = new Thread(this, name);
System.out.println("Created Thread: " + thread);
exitThread = false;
thread.start();
}
@Override
public void run() {
while (!exitThread) {
System.out.println(name + " is running");
try {
Thread.sleep(100);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
System.out.println(name + " has been Stopped.");
}
public void stopThread() {
exitThread = true;
}
}
Output:
Created Thread: Thread[Thread One,5,main]
Created Thread: Thread[Thread Two,5,main]
Thread Two is running
Thread One is running
Thread Two is running
Thread One is running
Thread One is running
Thread Two is running
Thread One is running
Thread Two is running
Thread One is running
Thread Two is running
**Exiting the main Thread**
Thread Two has been Stopped.
Thread One has been Stopped.
Kill or Stop a Thread Using interrupt()
in Java
We will use the previous example here, but with a new method called interrupt()
. This function stops the execution immediately when it is called on a thread. In this example, we are using thread.isInterrupted()
to check if interrupt()
was called.
To stop both the threads, we will call thread1.thread.interrupt()
and thread2.thread.interrupt()
resulting in termination of the threads.
public class KillThread {
public static void main(String[] args) {
ExampleThread thread1 = new ExampleThread("Thread One");
ExampleThread thread2 = new ExampleThread("Thread Two");
try {
Thread.sleep(6);
thread1.thread.interrupt();
thread2.thread.interrupt();
Thread.sleep(8);
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println("**Exiting the main Thread**");
}
}
class ExampleThread implements Runnable {
private String name;
Thread thread;
ExampleThread(String name) {
this.name = name;
thread = new Thread(this, name);
System.out.println("Created Thread: " + thread);
thread.start();
}
@Override
public void run() {
while (!thread.isInterrupted()) {
System.out.println(name + " is running");
}
System.out.println(name + " has been Stopped.");
}
}
Output:
Created Thread: Thread[Thread One,5,main]
Created Thread: Thread[Thread Two,5,main]
Thread One is running
Thread Two is running
Thread One has been Stopped.
Thread Two has been Stopped.
**Exiting the main Thread**
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn