Matar um thread em Java
-
Matar ou parar um thread utilizando uma bandeira
booleana
em Java -
Matar ou parar um thread utilizando
interrupt()
em Java
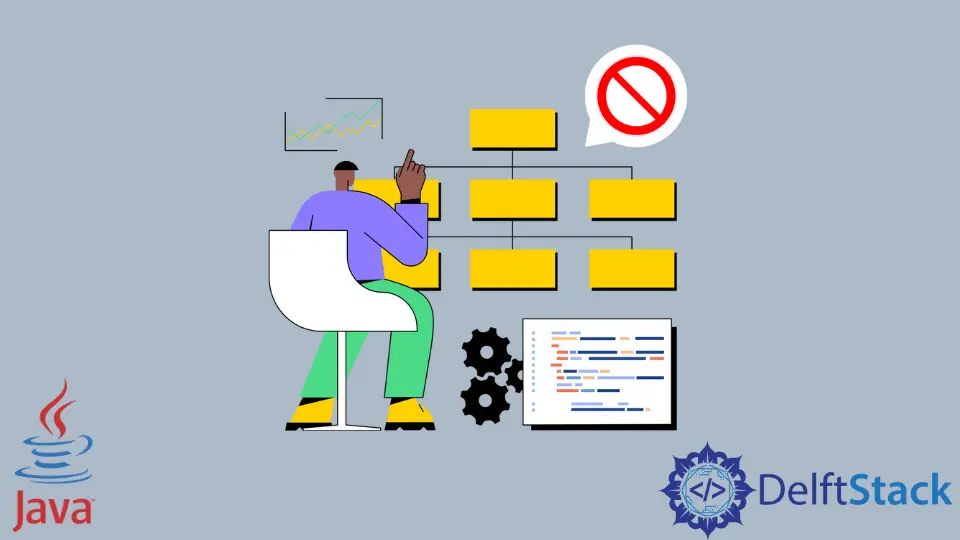
As roscas em Java permitem-nos executar várias tarefas em paralelo, o que permite multitarefas. Podemos criar um thread em Java utilizando a classe Thread
. Neste artigo, vamos introduzir dois métodos para matar um thread.
Embora o thread seja destruído pelo método run()
da classe Thread
uma vez completadas todas as tarefas, por vezes podemos querer matar ou parar um thread antes que este tenha terminado de executar completamente.
Matar ou parar um thread utilizando uma bandeira booleana
em Java
Para matar explicitamente um string, podemos usar uma bandeira booleana para notificar o string quando parar a tarefa. Abaixo, dois strings imprimem uma linha com o seu nome, e depois ambos os strings dormem durante cerca de 100 milissegundos. Os strings são executados até que a bandeira booleana exitThread
se torne verdadeira.
Os thread1
e thread2
são dois strings criados, e um nome é passado como um argumento em ambos. Como o construtor da classe ExampleThread
tem thread.start()
que inicia a execução de um thread, ambos os threads serão executados. Podemos ver que a saída imprime aleatoriamente os nomes de ambos os threads porque estão a ser executados em paralelo.
Para parar a thread, chamaremos stopThread()
, que é um método em ExampleThread
que define exitThread
como true
, e a thread finalmente pára porque while(!exitThread)
se torna false
.
public class KillThread {
public static void main(String[] args) {
ExampleThread thread1 = new ExampleThread("Thread One");
ExampleThread thread2 = new ExampleThread("Thread Two");
try {
Thread.sleep(500);
thread1.stopThread();
thread2.stopThread();
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println("**Exiting the main Thread**");
}
}
class ExampleThread implements Runnable {
private String name;
private boolean exitThread;
Thread thread;
ExampleThread(String name) {
this.name = name;
thread = new Thread(this, name);
System.out.println("Created Thread: " + thread);
exitThread = false;
thread.start();
}
@Override
public void run() {
while (!exitThread) {
System.out.println(name + " is running");
try {
Thread.sleep(100);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
System.out.println(name + " has been Stopped.");
}
public void stopThread() {
exitThread = true;
}
}
Resultado:
Created Thread: Thread[Thread One,5,main]
Created Thread: Thread[Thread Two,5,main]
Thread Two is running
Thread One is running
Thread Two is running
Thread One is running
Thread One is running
Thread Two is running
Thread One is running
Thread Two is running
Thread One is running
Thread Two is running
**Exiting the main Thread**
Thread Two has been Stopped.
Thread One has been Stopped.
Matar ou parar um thread utilizando interrupt()
em Java
Vamos utilizar aqui o exemplo anterior, mas com um novo método chamado interrupt()
. Esta função interrompe imediatamente a execução quando é chamada num string. Neste exemplo, estamos a utilizar thread.isInterrupted()
para verificar se a função interrupt()
foi chamada.
Para parar ambos os threads, chamaremos thread1.thread.interrupt()
e thread2.thread.interrupt()
, resultando na terminação dos threads.
public class KillThread {
public static void main(String[] args) {
ExampleThread thread1 = new ExampleThread("Thread One");
ExampleThread thread2 = new ExampleThread("Thread Two");
try {
Thread.sleep(6);
thread1.thread.interrupt();
thread2.thread.interrupt();
Thread.sleep(8);
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println("**Exiting the main Thread**");
}
}
class ExampleThread implements Runnable {
private String name;
Thread thread;
ExampleThread(String name) {
this.name = name;
thread = new Thread(this, name);
System.out.println("Created Thread: " + thread);
thread.start();
}
@Override
public void run() {
while (!thread.isInterrupted()) {
System.out.println(name + " is running");
}
System.out.println(name + " has been Stopped.");
}
}
Resultado:
Created Thread: Thread[Thread One,5,main]
Created Thread: Thread[Thread Two,5,main]
Thread One is running
Thread Two is running
Thread One has been Stopped.
Thread Two has been Stopped.
**Exiting the main Thread**
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn