Mod of Negative Numbers in Java
-
Get the Mod of Negative Numbers by Using
Math.floorMod
- Get the Mod of Negative Numbers by Using Custom Modulus Calculation
- Get the Mod of Negative Numbers by Using Ternary Operator
- Conclusion
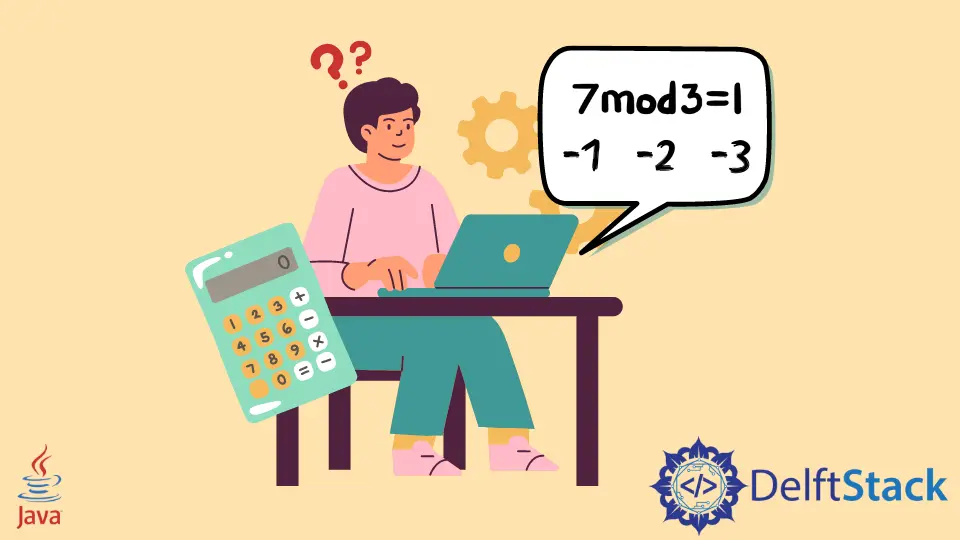
In Java programming, obtaining the modulus of negative numbers poses unique challenges, often requiring careful consideration to ensure accuracy and predictability. Three distinct methods—Math.floorMod
, custom modulus calculation
, and the ternary operator
—stand out as valuable approaches in handling this scenario.
The %
operator is the conventional choice for modulus calculations in Java. It determines the remainder when one number is divided by another.
While it performs admirably in many scenarios, it may yield unexpected results when dealing with negative numbers.
To overcome this, developers can adopt a strategy that adjusts the result based on the sign of the dividend.
In this exploration, we will delve into these three methods, understanding their significance and how they contribute to achieving reliable modulus calculations for negative numbers in Java.
Get the Mod of Negative Numbers by Using Math.floorMod
The importance of using Math.floorMod
when calculating the modulus of negative numbers lies in its ability to consistently provide the expected and mathematically correct results.
Math.floorMod
simplifies this process by offering a clear and concise solution. It always returns a non-negative result, regardless of the sign of the numbers involved.
This consistency is crucial for mathematical accuracy and simplifies code, making it more readable and less error-prone.
Let’s delve into a comprehensive code example to illustrate how Math.floorMod
can be employed to obtain the modulus of negative numbers.
public class ModulusExample {
public static void main(String[] args) {
// Example: Calculate the modulus of -17 with respect to 5
int dividend = -17;
int divisor = 5;
// Using Math.floorMod to get the modulus of negative numbers
int result = Math.floorMod(dividend, divisor);
// Display the result
System.out.println("Modulus of " + dividend + " with respect to " + divisor + ": " + result);
}
}
First, we initialize two variables, dividend
and divisor
, representing the numbers we want to find the modulus for.
Next, we apply the Math.floorMod
method by passing in the dividend
and divisor
as arguments. This method ensures that the modulus result is always non-negative, addressing the quirks associated with negative numbers and modulus operations.
Finally, we display the calculated result to the console, providing a clear and straightforward output for the modulus calculation.
Modulus of -17 with respect to 5: 3
Upon executing the code, the output will demonstrate the correct modulus calculation, even with negative numbers.
Get the Mod of Negative Numbers by Using Custom Modulus Calculation
Using custom modulus calculation for negative numbers is important because it provides control and clarity in handling modulus operations, ensuring predictable and desired outcomes. Unlike some other methods, a custom approach allows developers to tailor the behavior to specific requirements.
Custom modulus calculation, often in the form of (dividend % divisor + divisor) % divisor
, ensures a positive result regardless of the sign of the numbers involved. This predictability is valuable in scenarios where negative results could lead to incorrect program behavior.
Moreover, the custom approach is straightforward, readable, and does not require additional libraries or complex logic. It provides a concise solution that can be easily understood by other developers and maintains consistency across different scenarios.
Let’s dive into a complete code example to demonstrate the custom modulus calculation method:
public class CustomModulusExample {
public static void main(String[] args) {
// Example: Calculate the modulus of -22 with respect to 7
int dividend = -22;
int divisor = 7;
// Using Custom Modulus Calculation
int result = customModulus(dividend, divisor);
// Display the result
System.out.println("Modulus of " + dividend + " with respect to " + divisor + ": " + result);
}
// Custom Modulus Calculation Method
public static int customModulus(int dividend, int divisor) {
return (dividend % divisor + divisor) % divisor;
}
}
In the code breakdown, we first set up the variables dividend
and divisor
with values representing the numbers for our modulus operation.
Following that, we introduce a custom method called customModulus
, designed to handle the modulus calculation with more control. This method takes the dividend
and divisor
as inputs and employs a concise formula to ensure a non-negative result.
We then use this custom method to calculate the modulus, providing a clear and straightforward alternative to the standard modulus operator.
Finally, the calculated result is displayed to the console for quick verification.
Modulus of -22 with respect to 7: 6
Upon executing the code, the output will demonstrate the correct modulus calculation, ensuring a positive result for negative numbers.
Get the Mod of Negative Numbers by Using Ternary Operator
Using the ternary operator for the modulus of negative numbers is important for its simplicity and efficiency. It provides a concise way to adjust the result based on the sign of the dividend, ensuring correctness without introducing unnecessary complexity.
The ternary operator, represented as (dividend < 0 ? -1 : 1)
, quickly adjusts the sign, making the result consistently positive.
This method is particularly beneficial when readability and brevity are priorities. It condenses the logic into a single line, making the code easy to understand and reducing the chance of errors.
In scenarios where a quick adjustment based on the sign is all that’s needed, the ternary operator is an elegant and efficient choice.
Let’s dive into a complete code example to illustrate the ternary operator method:
public class TernaryModulusExample {
public static void main(String[] args) {
// Example: Calculate the modulus of -15 with respect to 4
int dividend = -15;
int divisor = 4;
// Using Ternary Operator for Modulus Calculation
int result = (dividend % divisor + divisor) % divisor * (dividend < 0 ? -1 : 1);
// Display the result
System.out.println("Modulus of " + dividend + " with respect to " + divisor + ": " + result);
}
}
In the code breakdown, we first initialize the variables dividend
and divisor
with values representing the numbers in our modulus operation.
Following that, we utilize the ternary operator to calculate the modulus, adjusting the result based on the sign of the dividend
. This concise approach ensures the final result consistently maintains the correct sign.
Finally, the calculated result is displayed to the console for quick verification, providing a clear and efficient solution for handling the modulus of negative numbers.
Modulus of -15 with respect to 4: -1
Upon executing the code, the output will showcase the correct modulus calculation using the ternary operator method.
Conclusion
Whether using Math.floorMod
, custom modulus calculation, or the ternary operator, each method offers unique advantages for handling the modulus of negative numbers in Java.
Math.floorMod
stands out for its reliability and adherence to mathematical principles, ensuring consistent and expected results.
On the other hand, custom modulus calculation provides versatility and control, addressing specific needs with simplicity.
Meanwhile, the ternary operator offers an elegant and concise solution, enhancing readability and efficiency in adjusting modulus results for negative numbers.
Developers can choose the method that best aligns with their preferences and the requirements of their applications.