Java 中負數的模
Joel Swapnil Singh
2023年10月12日
Java
Java Math
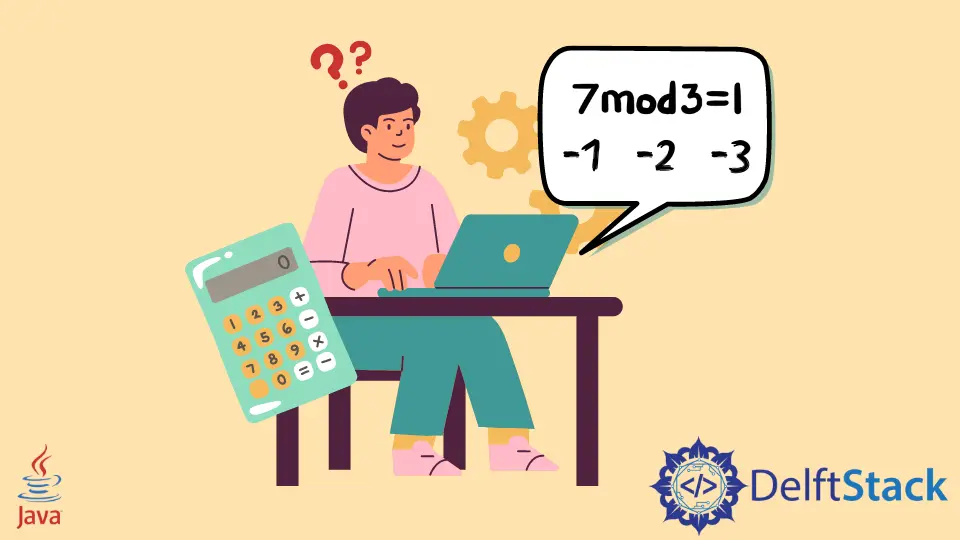
在 Java 中,我們可以藉助模或餘數運算子 (%
) 運算子找到兩個數相除後的餘數。
假設我們有兩個數字,A 和 B,其中 A 是被除數,B 是除數。當我們操作 A mod B 時,當 A 除以 B 時,我們得到一個餘數。
如果我們處理負數,我們有時會得到錯誤的結果。本文將討論在 Java 中查詢負數模的不同方法。
如果我們有負數並對其使用%
運算子,我們將得到取決於左運算元符號的結果。如果我們的左運算元為正,我們將得到結果為正,如果左運算元為負,我們將得到結果為負。
為了解決上述問題,我們可以通過使用%
運算子、floorMod()
方法和 Math.abs()
方法找到負數的模。讓我們一一討論每種方法。
在 Java 中使用 %
運算子獲取負數的模
我們可以通過首先新增模因子直到進入正域然後呼叫%
運算子來找到負數的模。在這種方法的幫助下,我們總是使 mod 因子大於輸出。
讓我們看看下面的程式碼來了解它是如何工作的。
public class Main {
public static void main(String args[]) {
int x = -4;
int y = 3;
int res = x % y;
System.out.println("Mod value before operation = " + res);
while (x < 0) x += y;
int mod_res = x % y;
System.out.println("Mod value after operation = " + mod_res);
}
}
輸出:
Mod value before operation = -1
Mod value after operation = 2
使用 floormod()
方法獲取負數的模
我們還可以使用 floorMod()
方法找到負數的 mod。在這個方法的幫助下,我們得到了 int 引數的底模。
讓我們看一下 floorMod()
函式的語法。
語法:
floorMod(number, mod)
當對負整數呼叫 floorMod()
函式時,我們會得到帶有 mod 因子符號的輸出。讓我們看看下面的程式碼來了解它是如何工作的。
public class Main {
public static void main(String args[]) {
int x = -4;
int y = 3;
int res = x % y;
System.out.println("Mod value before operation = " + res);
int mod_res = Math.floorMod(
x, y); // This will have the output value as positive since the smod factor is positive
System.out.println("Mod value after operation having mod factor as positive = " + mod_res);
x = 4;
y = -3;
mod_res = Math.floorMod(
x, y); // This will have the output value as negative since the mod factor is negative
System.out.println("Mod value after operation having mod factor as negative = " + mod_res);
}
}
輸出:
Mod value before operation = -1
Mod value after operation having mod factor as positive = 2
Mod value after operation having mod factor as negative = -2
使用 Math.abs()
方法獲取負數的 Mod
我們還可以使用 Math.abs()
方法找到負數的模。
我們將在三元運算子的幫助下執行此任務。無論數字是正數還是負數,此運算子都將產生兩種情況的輸出。
讓我們看看下面的程式碼來了解它是如何工作的。
public class Main {
public static void main(String args[]) {
int x = -4;
int y = 3;
int res = x % y;
System.out.println("Mod value before operation = " + res);
int mod_res = (x < 0) ? (y - (Math.abs(x) % y)) % y : (x % y);
System.out.println("Mod value after operation = " + mod_res);
}
}
輸出:
Mod value before operation = -1
Mod value after operation = 2
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe