Java 中负数的模
Joel Swapnil Singh
2023年10月12日
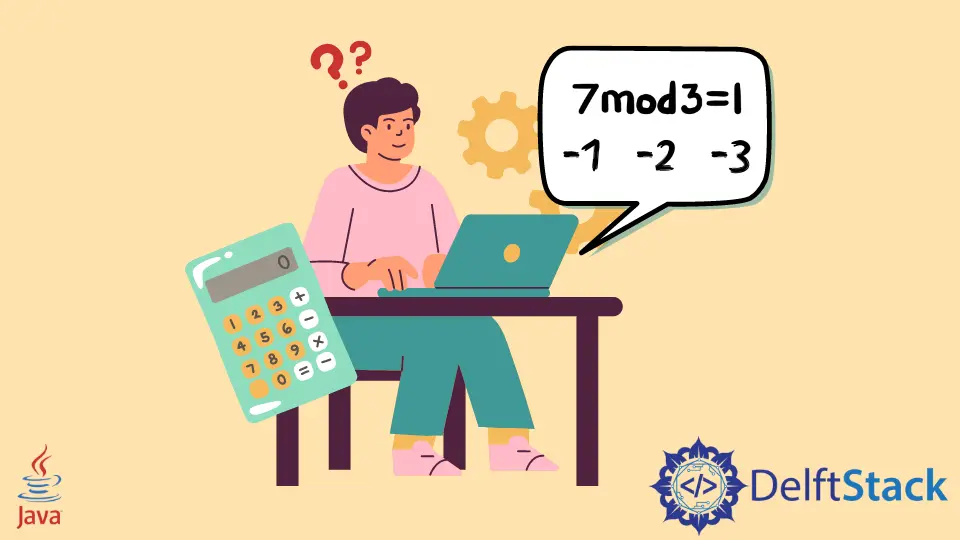
在 Java 中,我们可以借助模或余数运算符 (%
) 运算符找到两个数相除后的余数。
假设我们有两个数字,A 和 B,其中 A 是被除数,B 是除数。当我们操作 A mod B 时,当 A 除以 B 时,我们得到一个余数。
如果我们处理负数,我们有时会得到错误的结果。本文将讨论在 Java 中查找负数模的不同方法。
如果我们有负数并对其使用%
运算符,我们将得到取决于左操作数符号的结果。如果我们的左操作数为正,我们将得到结果为正,如果左操作数为负,我们将得到结果为负。
为了解决上述问题,我们可以通过使用%
运算符、floorMod()
方法和 Math.abs()
方法找到负数的模。让我们一一讨论每种方法。
在 Java 中使用 %
运算符获取负数的模
我们可以通过首先添加模因子直到进入正域然后调用%
运算符来找到负数的模。在这种方法的帮助下,我们总是使 mod 因子大于输出。
让我们看看下面的代码来了解它是如何工作的。
public class Main {
public static void main(String args[]) {
int x = -4;
int y = 3;
int res = x % y;
System.out.println("Mod value before operation = " + res);
while (x < 0) x += y;
int mod_res = x % y;
System.out.println("Mod value after operation = " + mod_res);
}
}
输出:
Mod value before operation = -1
Mod value after operation = 2
使用 floormod()
方法获取负数的模
我们还可以使用 floorMod()
方法找到负数的 mod。在这个方法的帮助下,我们得到了 int 参数的底模。
让我们看一下 floorMod()
函数的语法。
语法:
floorMod(number, mod)
当对负整数调用 floorMod()
函数时,我们会得到带有 mod 因子符号的输出。让我们看看下面的代码来了解它是如何工作的。
public class Main {
public static void main(String args[]) {
int x = -4;
int y = 3;
int res = x % y;
System.out.println("Mod value before operation = " + res);
int mod_res = Math.floorMod(
x, y); // This will have the output value as positive since the smod factor is positive
System.out.println("Mod value after operation having mod factor as positive = " + mod_res);
x = 4;
y = -3;
mod_res = Math.floorMod(
x, y); // This will have the output value as negative since the mod factor is negative
System.out.println("Mod value after operation having mod factor as negative = " + mod_res);
}
}
输出:
Mod value before operation = -1
Mod value after operation having mod factor as positive = 2
Mod value after operation having mod factor as negative = -2
使用 Math.abs()
方法获取负数的 Mod
我们还可以使用 Math.abs()
方法找到负数的模。
我们将在三元运算符的帮助下执行此任务。无论数字是正数还是负数,此运算符都将产生两种情况的输出。
让我们看看下面的代码来了解它是如何工作的。
public class Main {
public static void main(String args[]) {
int x = -4;
int y = 3;
int res = x % y;
System.out.println("Mod value before operation = " + res);
int mod_res = (x < 0) ? (y - (Math.abs(x) % y)) % y : (x % y);
System.out.println("Mod value after operation = " + mod_res);
}
}
输出:
Mod value before operation = -1
Mod value after operation = 2