How to Calculate Length of Integer in Java
-
Use the
for
Loop to Calculate the Length of an Integer in Java -
Use the
Math.log10()
Function to Calculate the Length of an Integer in Java -
Use the
toString()
Function to Calculate the Length of an Integer in Java - Use Recursion to Calculate the Length of an Integer in Java
- Conclusion
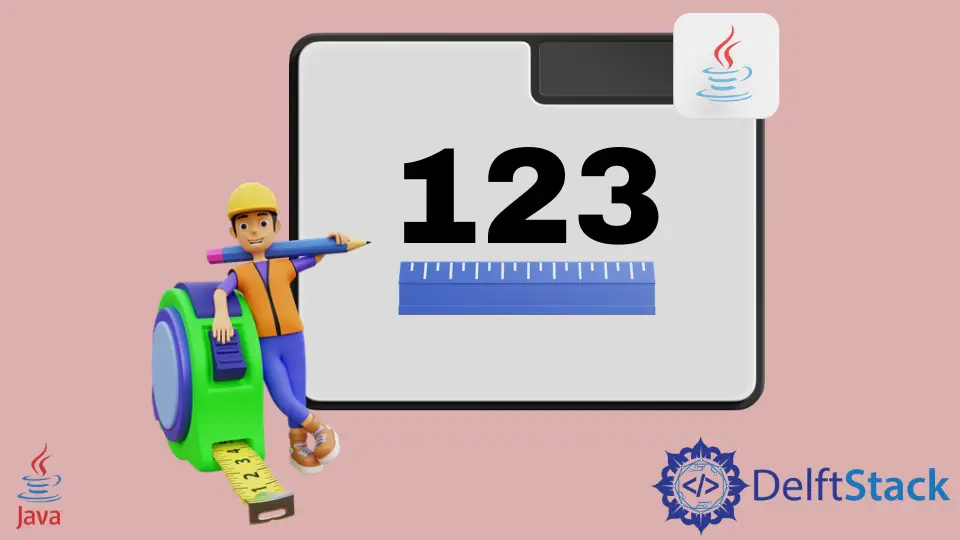
Determining the number of digits in an integer is a common task in programming, and Java provides several approaches to accomplish this.
In this article, we will explore various methods to calculate the number of digits in an integer, each showcasing a different technique.
Use the for
Loop to Calculate the Length of an Integer in Java
The for
loop in Java consists of three parts: initialization, condition, and iteration. The initialization part is executed only once at the beginning, the condition is evaluated before each iteration, and the iteration part is executed after each iteration.
This structure makes it suitable for tasks where a specific action needs to be repeated a certain number of times.
In our case, we’ll use the for
loop to iteratively divide the given integer by 10
until it becomes zero, counting the number of iterations. This count will represent the number of digits in the original integer.
public class CountDigits {
public static void main(String[] args) {
int number = 987654321;
int digitCount = 0;
for (int tempNumber = number; tempNumber != 0; tempNumber /= 10) {
digitCount++;
}
System.out.println("Number of digits: " + digitCount);
}
}
Output:
Number of digits: 9
In this example, we start by initializing the number
variable with the integer for which we want to count the digits. Next, we set up a variable digitCount
to store the count of digits, initializing it to zero.
The for
loop is the heart of this algorithm. It has three parts: initialization (int tempNumber = number
), condition (tempNumber != 0
), and iteration (tempNumber /= 10
).
The loop continues as long as tempNumber
is not zero. In each iteration, tempNumber
is divided by 10
, effectively removing the last digit. The digitCount
is then incremented.
Once the loop completes, we have the total count of digits stored in the digitCount
variable. Finally, we print the result using System.out.println()
.
Use the Math.log10()
Function to Calculate the Length of an Integer in Java
An alternative approach to determine the number of digits in an integer in Java is using the Math.log10()
and Math.floor()
methods. This method leverages the logarithmic property of base 10
to efficiently calculate the number of digits.
Note that this method will work only on positive integers.
Code Example:
public class CountDigitsWithLogarithm {
public static void main(String[] args) {
int number = 456789;
int digitCount = (int) Math.floor(Math.log10(number) + 1);
System.out.println("Number of digits: " + digitCount);
}
}
Output:
Number of digits: 6
In this example, we begin by initializing the number
variable with the integer for which we want to count the digits. The critical logic lies in the single line where we calculate the digitCount
.
We use Math.log10(number)
to find the logarithm base 10
of the given integer. The result of this operation represents the power to which 10
must be raised to obtain the original number.
However, since we are interested in the count of digits, we add 1
to the result to include the leftmost digit.
The entire expression is then cast to an integer using (int)
to discard the fractional part and obtain a whole number. This final value is assigned to the digitCount
variable.
Finally, we print the result using System.out.println()
.
Use the toString()
Function to Calculate the Length of an Integer in Java
Another method is to change the integer into a string using the toString()
function and then determine its length using the string’s length property. This is an easy way to count the digits without the need for complex mathematical operations or loops.
Code Example:
public class CountDigitsWithStringConversion {
public static void main(String[] args) {
int number = 987654;
String numberString = Integer.toString(number);
int digitCount = numberString.length();
System.out.println("Number of digits: " + digitCount);
}
}
Output:
Number of digits: 6
In this example, we start by initializing the number
variable with the integer for which we want to count the digits. The key to this approach lies in the following two lines of code.
Firstly, we use Integer.toString(number)
to convert the integer into its string representation. This allows us to treat each digit as a character within the resulting string.
The string is then assigned to the numberString
variable.
Subsequently, we use the length()
function on numberString
to retrieve the count of characters in the string, which directly corresponds to the number of digits in the original integer. The obtained value is stored in the digitCount
variable.
Finally, we print the result using System.out.println()
.
Use Recursion to Calculate the Length of an Integer in Java
Determining the count of digits in an integer can also be achieved through a recursive approach, providing an elegant solution that employs a function calling itself.
Recursion involves breaking down a problem into smaller instances of the same problem until it reaches a base case.
This method is not only a powerful programming technique but also offers a different perspective on problem-solving.
Code Example:
public class CountDigitsWithRecursion {
public static void main(String[] args) {
int number = 54321;
int digitCount = countDigits(number);
System.out.println("Number of digits: " + digitCount);
}
public static int countDigits(int n) {
if (n == 0) {
return 0;
}
return 1 + countDigits(n / 10);
}
}
Output:
Number of digits: 5
In this example, we initialize the number
variable with the integer for which we want to count the digits. The heart of the logic lies in the countDigits
method, a recursive function.
The base case of the recursion checks if the given number n
is zero. If true, it means there are no more digits to count, and the function returns 0
.
In the recursive case, the function returns 1
plus the result of calling itself with the remaining part of the number (n / 10
). This recursive call effectively removes the last digit in each iteration, and the count accumulates.
Back in the main
method, we call countDigits
with the original number and store the result in the digitCount
variable. Finally, we print the result using System.out.println()
.
Conclusion
In conclusion, Java offers several versatile methods for calculating the number of digits in an integer, each presenting unique strengths and applications. The selection of a particular method depends on factors such as code readability, efficiency, and the specific requirements of the project.
Whether employing a for
loop for its simplicity, leveraging the mathematical properties with Math.log10()
, utilizing string conversion for an intuitive approach, or embracing recursion for an elegant solution, Java provides programmers with a variety of tools to address this common programming task. Ultimately, the most suitable method will depend on the context of the problem at hand and the preferences of the developer.