How to Display Text in JavaFX
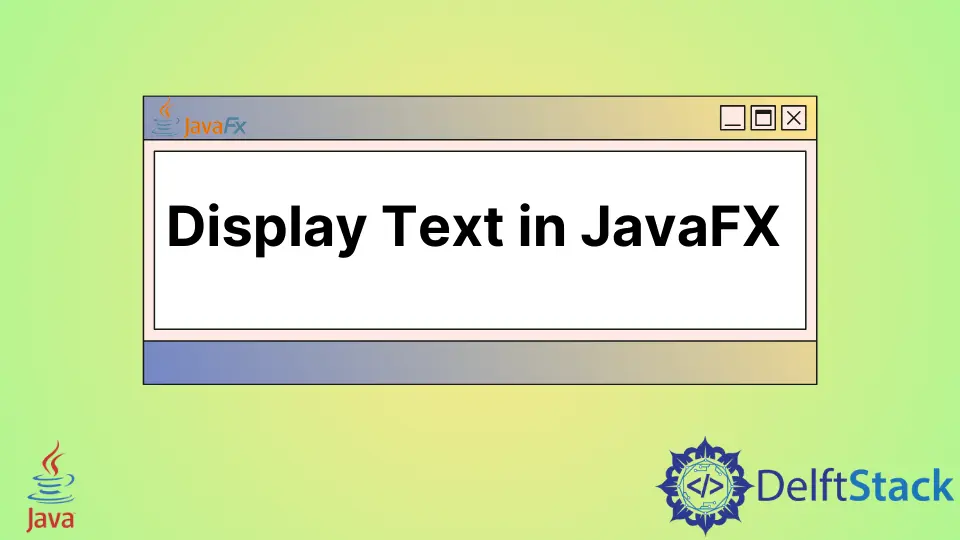
The text can be created and displayed using the JavaFX.scene.text.Text
class.
This tutorial demonstrates how to display single and multiline text in JavaFX.
The Text
class in JavaFX serves as a straightforward and effective means to display read-only, single-line text, and multiline text. Whether it’s a label, a brief message, or any other form of textual content, the Text
class proves to be an invaluable tool in the JavaFX developer’s toolkit.
In this article, we’ll explore the simplicity and versatility of the Text
class, an essential component for showcasing text in JavaFX applications.
JavaFX Display Text
JavaFX enables developers to display text in graphical user interfaces (GUIs) with ease. Using the Text class
, developers can create and manipulate text elements, setting properties such as font, color, and alignment.
JavaFX provides a straightforward way to integrate text into applications, offering a versatile and visually appealing means of presenting information within the user interface.
The Text
class in JavaFX is part of the javafx.scene.text
package and is commonly used to display a single-line and multi-line of read-only text. This class is particularly handy when you need a straightforward way to showcase static text without the need for user interaction.
Syntax:
Text text = new Text(text);
Where the text
as the parameter is the text value.
You can then add this Text
node to a layout or scene.
Let’s dive into a comprehensive example to illustrate its usage.
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.VBox;
import javafx.scene.text.Text;
import javafx.stage.Stage;
public class TextDisplayExample extends Application {
@Override
public void start(Stage primaryStage) {
// Creating a Text node for single-line text
Text singleLineText = new Text("Hello! This is delftstack.com");
// Creating a Text node for multi-line text
Text multiLineText =
new Text("\n\nDelftStack is a resource for everyone interested in programming, "
+ "\nembedded software, and electronics. It covers the programming languages "
+ "\nlike Python, C/C++, C#, and so on in this website's first development stage. "
+ "\nOpen-source hardware also falls in the website's scope, like Arduino, "
+ "\nRaspberry Pi, and BeagleBone. DelftStack aims to provide tutorials, "
+ "\nhow-to's, and cheat sheets to different levels of developers and hobbyists..");
// Creating a VBox layout to hold the Text nodes
VBox root = new VBox(singleLineText, multiLineText);
// Creating a Scene with the VBox as the root
Scene scene = new Scene(root, 400, 200);
// Setting the stage title and scene
primaryStage.setTitle("Text Display Example");
primaryStage.setScene(scene);
// Showing the stage
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
In this JavaFX example, we start by importing the necessary classes.
The TextDisplayExample
class extends the Application
class, marking it as a JavaFX application.
We then override the start
method, the entry point for JavaFX applications. Two Text
nodes are created—one for single-line text and another for multi-line text.
These nodes are vertically arranged using a VBox
layout.
A Scene
is established with the VBox
as the root, sized at 300x200 pixels. Stage properties, such as the title, are configured, and the scene is associated with it.
Finally, the stage is displayed, making the UI visible.
Upon running this JavaFX application, a window titled Text Display Example
will appear. It contains two Text
nodes: one displaying the single-line text Hello! This is delftstack.com
, and the other shows the multi-line text.
Output:
The output exemplifies the flexibility of the Text
class in handling both single-line and multi-line text in a JavaFX application.
Text Display with Styling Options
In JavaFX, the ability to display text is not only functional but also highly customizable. The Text
class provides a variety of methods that allow developers to go beyond basic text rendering.
JavaFX empowers developers to display text with style in graphical user interfaces. Using the Text
class, developers can easily apply styling options such as font size, color, style, and alignment, enhancing the visual appeal of text elements.
This flexibility allows for creative and customized presentation of text within JavaFX applications, offering a seamless way to design engaging user interfaces.
This table demonstrates the methods, their descriptions, parameters, and how they can be used to customize text in JavaFX.
Method | Description | Parameters | Example |
---|---|---|---|
setFont(Font font) |
Sets the font to be used for the text. | Font font - The font to be set. |
Font font = Font.font("Arial", FontWeight.BOLD, 16); text.setFont(font); |
setFill(Paint fill) |
Sets the fill color of the text. | Paint fill - The fill color. |
text.setFill(Color.BLUE); |
setStroke(Paint stroke) |
Sets the stroke color of the text. | Paint stroke - The stroke color. |
text.setStroke(Color.RED); |
setX(double x) |
Sets the x-coordinate of the text. | double x - The x-coordinate. |
text.setX(50); |
setY(double y) |
Sets the y-coordinate of the text. | double y - The y-coordinate. |
text.setY(50); |
setStrikethrough(boolean value) |
Applies or removes strikethrough style. | boolean value - true to apply strikethrough, false to remove. |
text.setStrikethrough(true); |
setUnderline(boolean value) |
Applies or removes underline style. | boolean value - true to apply to underline, false to remove. |
text.setUnderline(true); |
setSmooth(boolean value) |
Enables or disables font smoothing. | boolean value - true to enable smoothing, false to disable. |
text.setSmooth(true); |
In this section, we’ll explore how to leverage the Text
class and its styling options to create visually appealing and dynamic text displays.
Let’s dive into a comprehensive example that showcases the versatility of the Text
class:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.StackPane;
import javafx.scene.paint.Color;
import javafx.scene.text.Font;
import javafx.scene.text.Text;
import javafx.stage.Stage;
public class StyledTextExample extends Application {
@Override
public void start(Stage primaryStage) {
// Creating a Text node with the desired text
Text styledText = new Text("JavaFX Styling");
// Applying styling options
styledText.setFont(Font.font("Arial", 24));
styledText.setFill(Color.BLUE);
styledText.setStroke(Color.RED);
styledText.setX(50);
styledText.setY(50);
styledText.setStrikethrough(true);
styledText.setUnderline(true);
styledText.setSmooth(true);
// Creating a StackPane layout to hold the Text node
StackPane root = new StackPane();
root.getChildren().add(styledText);
// Creating a Scene with the StackPane as the root
Scene scene = new Scene(root, 300, 200);
// Setting the stage title and scene
primaryStage.setTitle("Styled Text Example");
primaryStage.setScene(scene);
// Showing the stage
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
In this JavaFX example, we start by importing the necessary JavaFX classes.
Following that, a class named StyledTextExample
is created, extending the Application
class and marking it as a JavaFX application.
The start
method is then overridden, serving as the entry point.
A Text
node is created with the text JavaFX Styling
, and various styling options are applied to enhance its appearance.
This styled Text
node is then added to a StackPane
layout. A Scene
is created with the StackPane
as the root, sized at 300x200 pixels.
Stage properties, such as the title, are configured, and the scene is associated with the stage.
Finally, the stage is displayed, rendering the styled text visible to the user.
Upon running this JavaFX application, a window with the title Styled Text Example
will appear, showcasing the text JavaFX Styling
with the specified font, fill and stroke colors, position, text origin, strikethrough, underline, and font smoothing.
Output:
The output demonstrates how the combination of these styling options can be used to create visually appealing and customized text displays in a JavaFX application.
Conclusion
The Text
class in JavaFX provides a straightforward method for displaying text in a graphical user interface. Its ease of use and flexibility make it a valuable tool for both beginners and experienced JavaFX developers alike.
Customizing the appearance of text in a user interface is crucial for creating engaging and aesthetically pleasing applications. The Text
class in JavaFX goes beyond mere content presentation, offering developers a palette of styling options to enhance the visual appeal of displayed text.
The Text
class in JavaFX empowers developers to go beyond basic text rendering by providing a rich set of styling options. Whether it’s adjusting fonts, colors, positions, or text decorations, these capabilities enhance the visual appeal of text in user interfaces, contributing to a more engaging user experience.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook