JavaFX 표시 텍스트
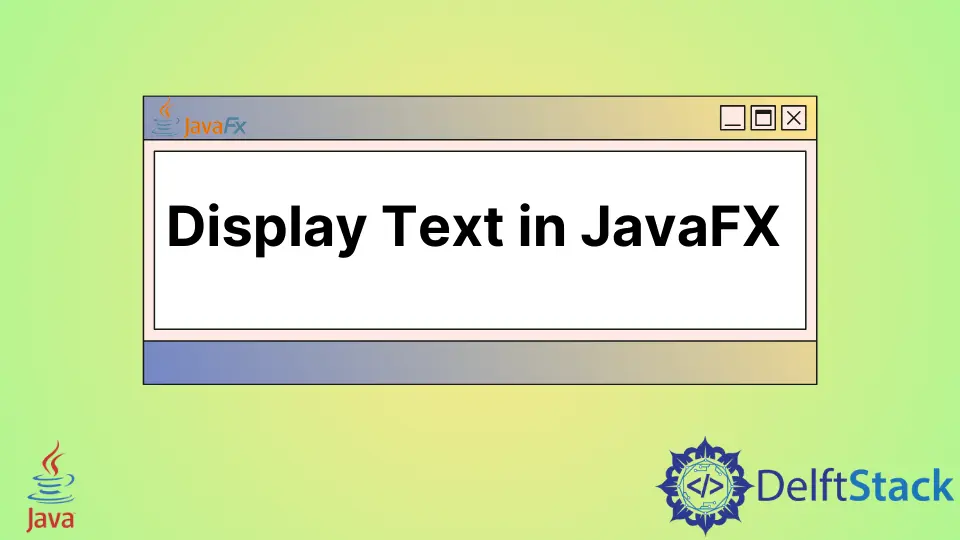
JavaFX.scene.text.Text
클래스를 사용하여 텍스트를 만들고 표시할 수 있습니다. 이 자습서는 JavaFX에서 단일 및 여러 줄 텍스트를 표시하는 방법을 보여줍니다.
JavaFX 표시 텍스트
JavaFX.scene,text.Text
는 JavaFX에서 텍스트를 만들고 표시하는 데 사용됩니다. Text
클래스를 인스턴스화하여 텍스트 노드를 만들고 장면에 표시할 수 있습니다.
통사론:
Text text = new Text(text);
여기서 매개변수인 text
는 텍스트 값입니다. 텍스트의 x 및 y 위치 값을 설정하려면 다음 방법을 사용합니다.
text.setX(30);
text.setY(30);
위의 메서드는 메서드에 지정된 x 및 y 위치에 따라 텍스트의 위치를 설정합니다. JavaFX에서 텍스트를 만들고 표시하려면 아래 단계를 따르십시오.
Application
클래스를 확장하고start()
메서드를 구현하여 클래스를 생성합니다.Text
클래스를 인스턴스화하여 텍스트를 작성하십시오. 그런 다음setX()
및setY()
메서드를 사용하여x
및y
위치를 설정합니다.그룹
클래스를 만듭니다.- 장면 개체를 만들고
장면
클래스를 인스턴스화하고그룹
개체를장면
에 전달합니다. setTitle
메소드를 사용하여 스테이지에 제목을 추가하고setScene()
메소드를 사용하여 스테이지에 장면을 추가합니다.show()
메서드를 사용하여 스테이지를 표시하고 애플리케이션을 시작합니다.
위의 단계를 기반으로 예제를 구현해 보겠습니다.
예제 코드:
package delftstack;
import javafx.application.Application;
import javafx.scene.Group;
import javafx.scene.Scene;
import javafx.scene.text.Text;
import javafx.stage.Stage;
public class JavaFX_Display_Text extends Application {
@Override
public void start(Stage Demo_Stage) {
// Create a Text object
Text Demo_Text = new Text();
// Set the text to be added.
Demo_Text.setText("Hello, This is delftstack.com");
// set the position of the text
Demo_Text.setX(80);
Demo_Text.setY(80);
// Create a Group object
Group Group_Root = new Group(Demo_Text);
// Create a scene object
Scene Demo_Scene = new Scene(Group_Root, 600, 300);
// Set title to the Stage
Demo_Stage.setTitle("Text Display");
// Add scene to the stage
Demo_Stage.setScene(Demo_Scene);
// Display the contents of the stage
Demo_Stage.show();
}
public static void main(String args[]) {
launch(args);
}
}
위의 코드는 장면에 텍스트
를 만들고 표시합니다.
출력:
Text
대신 Label
을 사용하여 여러 줄 텍스트를 표시할 수 있습니다. Label
을 만들고 Text
를 전달합니다.
여러 줄 텍스트로 표시하려면 Text
를 Label
로 래핑해야 합니다.
예제 코드:
package delftstack;
import javafx.application.Application;
import javafx.scene.Group;
import javafx.scene.Scene;
import javafx.scene.control.Label;
import javafx.scene.paint.Color;
import javafx.stage.Stage;
public class JavaFX_Display_Text extends Application {
@Override
public void start(Stage Demo_Stage) {
String Content = "DelftStack is a resource for everyone interested in programming, "
+ "embedded software, and electronics. It covers the programming languages "
+ "like Python, C/C++, C#, and so on in this website's first development stage. "
+ "Open-source hardware also falls in the website's scope, like Arduino, "
+ "Raspberry Pi, and BeagleBone. DelftStack aims to provide tutorials, "
+ "how-to's, and cheat sheets to different levels of developers and hobbyists..";
// Create a Label
Label Demo_Text = new Label(Content);
// wrap the label
Demo_Text.setWrapText(true);
// Set the maximum width of the label
Demo_Text.setMaxWidth(300);
// Set the position of the label
Demo_Text.setTranslateX(30);
Demo_Text.setTranslateY(30);
Group Text_Root = new Group();
Text_Root.getChildren().add(Demo_Text);
// Set the stage
Scene Text_Scene = new Scene(Text_Root, 595, 150, Color.BEIGE);
Demo_Stage.setTitle("Display Multiline Text");
Demo_Stage.setScene(Text_Scene);
Demo_Stage.show();
}
public static void main(String args[]) {
launch(args);
}
}
위의 코드는 여러 줄로 레이블에 래핑된 텍스트를 표시합니다.
출력:
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook