How to Write Strings to CSV File in Java
-
Using
PrintWriter
to Read and Write Into a CSV File in Java -
Using
OpenCSV
Library to Read and Write Into a CSV File in Java
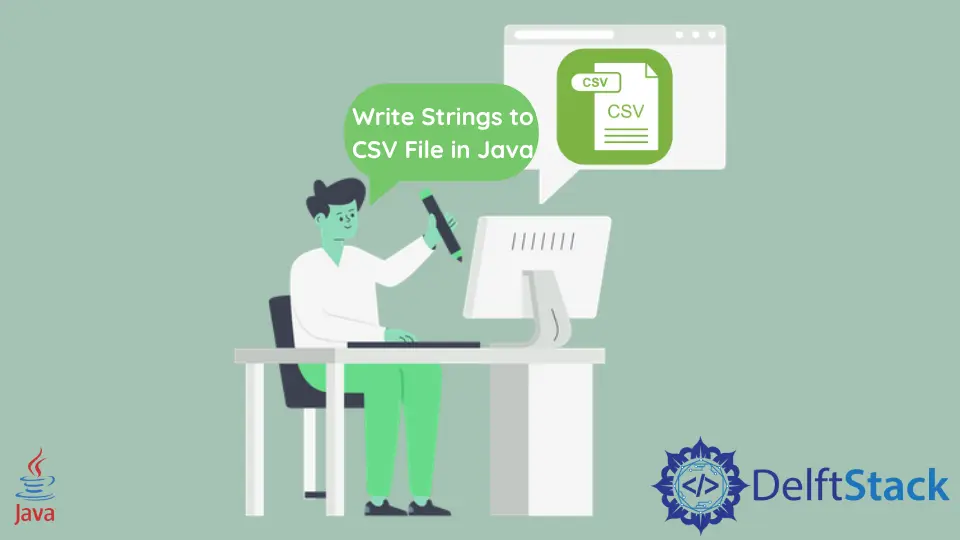
CSV stands for Comma Separated Values
; it’s a commonly used format for bulky data transfers between systems. There are also many parser libraries to be used along with Java to be able to work with this format.
Using PrintWriter
to Read and Write Into a CSV File in Java
The PrinterWriter
function in Java is a writer class used to print formatted representation of objects to a text-output stream. We create a writer
object passing a new file named test.csv
as the destination for the writer. Here, the sb
object appends a specified string to the character sequence.
The write()
method on the writer
instance writes the textual content into the stream. The flush()
method flushes the contents into the file, and the close()
method permanently closes the stream. We can also read the contents of the test.csv
file.
The readCSVFile()
method is called on the TestCSV
class instance. Here, we created an instance of the Scanner
class with values from the specified file. It breaks data into tokens using a delimiter pattern. The hasNextLine()
returns true if the scanner has another line of input. Thus, we read each line of token data using the next()
method and store it in an array, finally printing the output.
import java.io.File;
import java.io.FileNotFoundException;
import java.io.PrintWriter;
import java.util.ArrayList;
import java.util.List;
import java.util.Scanner;
public class TestCSV {
public static void main(String args[]){
try (PrintWriter writer = new PrintWriter(new File("test.csv"))) {
StringBuilder sb = new StringBuilder();
sb.append("id");
sb.append(',');
sb.append("Name");
sb.append(',');
sb.append("Address");
sb.append('\n');
sb.append("101");
sb.append(',');
sb.append("John Doe");
sb.append(',');
sb.append("Las Vegas");
sb.append('\n');
writer.write(sb.toString());
writer.close();
System.out.println("done!");
} catch (FileNotFoundException e) {
System.out.println(e.getMessage());
}
TestCSV testCSV = new TestCSV();
testCSV.readCSVFile();
}
public void readCSVFile(){
List<List<String>> records = new ArrayList<>();
try (Scanner scanner = new Scanner(new File("test.csv"));) {
while (scanner.hasNextLine()) {
records.add(getRecordFromLine(scanner.nextLine()));
}
} catch (FileNotFoundException e) {
e.printStackTrace();
}
System.out.println(records.toString());
}
private List<String> getRecordFromLine(String line) {
List<String> values = new ArrayList<String>();
try (Scanner rowScanner = new Scanner(line)) {
rowScanner.useDelimiter(",");
while (rowScanner.hasNext()) {
values.add(rowScanner.next());
}
}
return values;
}
}
Output:
done!
[[id, Name, Address], [101, John Doe, Las Vegas]]
Using OpenCSV
Library to Read and Write Into a CSV File in Java
The maven dependency for this library is given below:
<dependency>
<groupId>com.opencsv</groupId>
<artifactId>opencsv</artifactId>
<version>5.4</version>
</dependency>
The OpenCsv
is a simple parser library for java; it has a set of OpenCsv classes which we use to read and write to a CSV file. In the main()
method, we first call the method to write to a CSV file using the CSVWriter
class. This class is used to write CSV data to writer implementation.
We create a writer
instance of CSVWriter
and call the writeNext()
function on this object to generate a CSV file with data from an array of strings separated using a delimiter. The close()
method closes the writer stream.
To read the data from the file we created in the CSV format, we call the readCSVFile
method on the main class object, where we parse the CSV file. To read all the records into a list at once, we use the readAll()
method. We loop over each record and print it out.
import com.opencsv.CSVReader;
import com.opencsv.CSVWriter;
import com.opencsv.exceptions.CsvException;
import java.io.FileNotFoundException;
import java.io.FileReader;
import java.io.FileWriter;
import java.io.IOException;
import java.util.Arrays;
import java.util.List;
public class CSVLibrary {
public static void main(String args[]) {
CSVLibrary csvLibrary = new CSVLibrary();
csvLibrary.writeToCsv();
csvLibrary.readCSVFile();
}
public void writeToCsv() {
String csv = "data.csv";
try {
CSVWriter writer = new CSVWriter(new FileWriter(csv));
String[] record = "2,Virat,Kohli,India,30".split(",");
writer.writeNext(record);
writer.close();
} catch (IOException e) {
e.printStackTrace();
}
}
public void readCSVFile() {
CSVReader reader = null;
try {
reader = new CSVReader(new FileReader("data.csv"));
} catch (FileNotFoundException e) {
e.printStackTrace();
}
List<String[]> allRows = null;
try {
allRows = reader.readAll();
} catch (IOException e) {
e.printStackTrace();
} catch (CsvException e) {
e.printStackTrace();
}
for (String[] row : allRows) {
System.out.println(Arrays.toString(row));
}
}
}
Output:
[2, Virat, Kohli, India, 30]
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn