How to Parse CSV in Java
-
Parse CSV Using
Scanner
in Java -
Parse CSV Using
String.split()
in Java -
Parse CSV Using
OpenCSV
in Java
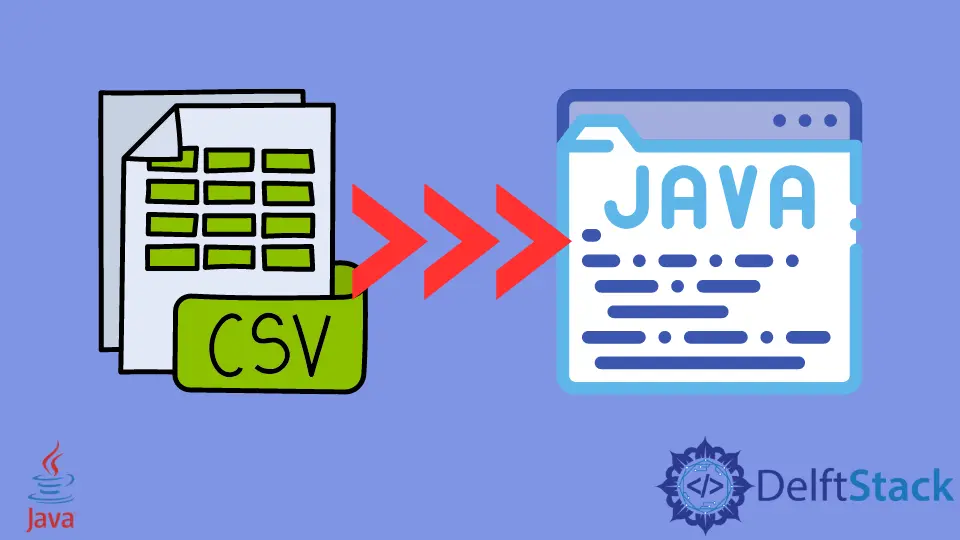
We will touch on the topic of parsing a CSV in Java. We will look at the various methods to do Java CSV parsing of the below sample CSV in Java.
Parse CSV Using Scanner
in Java
The first and most classic way to parse a CSV file is using the Scanner
class of Java. In the example, we get the file using File
and then read it by Scanner
.
The most important thing to notice in this example is that we must know the separator used in CSV. We use the comma in the original CSV as the separator; that is why we can specify ,
as a delimiter in the code.
import java.io.*;
import java.util.Scanner;
public class Main {
public static void main(String[] args) throws FileNotFoundException {
File getCSVFiles = new File("/test/example.csv");
Scanner sc = new Scanner(getCSVFiles);
sc.useDelimiter(",");
while (sc.hasNext()) {
System.out.print(sc.next() + " | ");
}
sc.close();
}
}
Output:
Id | UserName | Age | Job
1 | John Doe | 24 | Developer
2 | Alex Johnson | 43 | Project Manager
3 | Mike Stuart | 26 | Designer
4 | Tom Sean | 31 | CEO |
Parse CSV Using String.split()
in Java
In the next example, we use a split()
method that works with the String
class. We can use BufferedReader
to read the CSV file and loop through it reading every line until it reaches null
.
import java.io.*;
import java.util.Scanner;
public class Main {
public static void main(String[] args) throws FileNotFoundException {
String line = "";
final String delimiter = ",";
try {
String filePath = "/test/example.csv";
FileReader fileReader = new FileReader(filePath);
BufferedReader reader = new BufferedReader(fileReader);
while ((line = reader.readLine()) != null) // loops through every line until null found
{
String[] token = line.split(delimiter); // separate every token by comma
System.out.println(token[0] + " | " + token[1] + " | " + token[2] + " | " + token[3]);
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
Output:
Id | UserName | Age | Job
1 | John Doe | 24 | Developer
2 | Alex Johnson | 43 | Project Manager
3 | Mike Stuart | 26 | Designer
4 | Tom Sean | 31 | CEO
Parse CSV Using OpenCSV
in Java
Several libraries can help us to parse the CSV in Java. One of them is OpenCSV
. In the below example, CSVReader()
takes a fileReader
with the CSV file and then returns an array of strings.
import com.opencsv.CSVReader;
import java.io.*;
import java.util.List;
import java.util.Scanner;
import java.util.Vector;
public class Main {
public static void main(String[] args) throws Exception {
try {
String filePath = "/test/example.csv";
FileReader fileReader = new FileReader(filePath);
CSVReader openCSVReader = new CSVReader(fileReader);
String[] record;
while ((record = openCSVReader.readNext()) != null) {
for (String token : record) {
System.out.print(token + "\t");
}
System.out.println();
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
Output:
Id UserName Age Job
1 John Doe 24 Developer
2 Alex Johnson 43 Project Manager
3 Mike Stuart 26 Designer
4 Tom Sean 31 CEO
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn